Software security refers to the discipline of implementing processes, methodologies, and tools to design, develop, and maintain software applications that are resistant to unauthorized access, attacks, or malfunctions. It encompasses practices to prevent vulnerabilities, thwart malicious attacks, and protect valuable data by integrating security measures throughout the software development lifecycle (SDLC). As cyber threats evolve, software security ensures the confidentiality, integrity, and availability of both software solutions and the data they process.
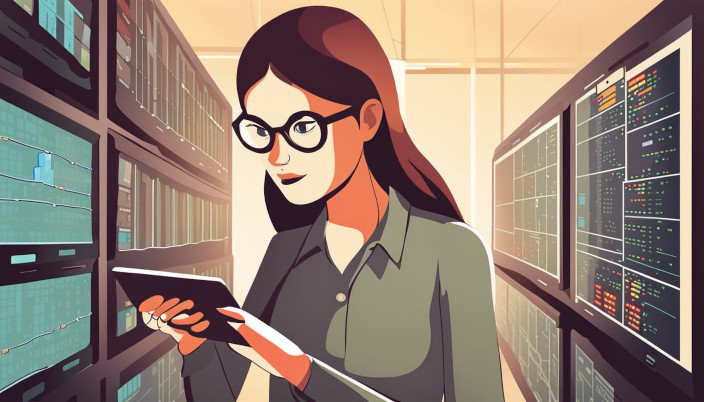
Threats and Vulnerabilities
Vulnerabilities
A vulnerability is a flaw or weakness in a system’s design, implementation, or operation and management that could be exploited to compromise the system’s security objectives.
Characteristics of Vulnerabilities
Inherent Weaknesses: Vulnerabilities arise from mistakes in software design, coding errors, or system configuration mishaps.
Exploitation: A vulnerability can be exploited by threats to breach or compromise a system. This exploitation can be unintentional (like a user mistakenly accessing data) or intentional (as with malicious actors).
Discovery: Vulnerabilities can be discovered by internal development teams, independent researchers, or even malicious actors. Once identified, they should be rectified promptly to prevent exploitation.
Examples
SQL Injection (SQLi)
SQL injection (SQLi) is one of the most well-known web application vulnerabilities. It involves the exploitation of an application’s data input fields to gain unauthorized access or interact with the database behind the application.
How SQL Injection Works
When a web application fails to properly sanitize user input, an attacker can inject malicious SQL code into input fields. This malicious input is then executed by the backend database, allowing the attacker to execute arbitrary SQL commands.
For example, consider a basic login form with two fields: username and password. Upon submission, the back-end might execute the following SQL statement:
SELECT * FROM users WHERE username = ‘[INPUT_USERNAME]’ AND password = ‘[INPUT_PASSWORD]’
If the application doesn’t sanitize the input, an attacker might input the following for the username field:
‘ OR ‘1’ = ‘1’ —
This would modify the SQL statement to:
SELECT * FROM users WHERE username = ” OR ‘1’ = ‘1’ — ‘ AND password = ‘[INPUT_PASSWORD]’
The double hyphen (–) is an SQL comment, which causes the rest of the query to be ignored. As a result, the attacker bypasses the password check entirely and might gain access to the system.
Types of SQL Injection
Classic SQLi: This is the type of injection attack described above.
Blind SQLi: The application doesn’t show errors, but the attacker can still retrieve information by sending specific queries and observing the application’s behavior.
Time-based Blind SQLi: The attacker determines if the hypothesis is true based on how long it takes the application to respond.
Out-of-band SQLi: Data is retrieved using a different communication channel (e.g., making a DNS request or HTTP request to an external server).
Mitigation
Parameterized Queries: This is the most effective way to prevent SQL injection. Instead of inserting user input directly into queries, you use parameters.
Escaping User Input: This involves making user input safe before it’s inserted into queries.
Using ORM (Object Relational Mapping): ORMs like Hibernate or Django’s ORM can automatically escape user inputs.
Web Application Firewalls (WAFs): These can be used to detect and block SQL injection attacks.
Regularly Update and Patch: Keeping your systems and software updated ensures that you’re protected from known vulnerabilities.
Least Privilege: Make sure the database user employed by the web app has only the necessary permissions, not full administrative rights.
Buffer Overflow
A buffer overflow is one of the most well-known and critical vulnerabilities in software security. It occurs when data is written into a buffer (an allocated memory region) and overflows the buffer’s boundaries, subsequently overwriting adjacent memory locations.
How Buffer Overflow Works
When a program writes more data to a buffer than it can handle due to a lack of bounds checking, this results in an overflow. This can corrupt data, crash the program, or, in the worst cases, allow attackers to execute arbitrary code.
Types of Buffer Overflows
Stack Overflow: This type of overflow targets the call stack. The most common buffer overflow attacks are against the stack because it holds function return addresses and overflowing it can redirect the program to malicious code.
Heap Overflow: This targets the heap, which is a larger area of memory where dynamic memory allocation takes place. Overflowing buffers on the heap can also lead to arbitrary code execution.
Integer Overflow: This happens when an arithmetic operation results in a value outside the range that can be represented. It can be used to cause buffer overflows by manipulating the size of memory that gets allocated or the location where data gets written.
Consequences of Buffer Overflow Attacks
Denial of Service (DoS): A program can crash if its memory is corrupted by a buffer overflow.
Arbitrary Code Execution: Attackers can inject and execute malicious code. This is often achieved by overwriting function return addresses or manipulating data structures.
Privilege Escalation: If a vulnerable program runs with elevated privileges, an attacker might gain those privileges by exploiting the buffer overflow.
Mitigation Techniques
Bounds Checking: Ensure that the program checks data lengths before copying them into buffers.
Non-executable Stack/Heap: Modern systems can mark memory sections like the stack or heap as non-executable, making it harder to run malicious code even if an overflow occurs.
Address Space Layout Randomization (ASLR): Randomly rearranges the memory addresses used by processes, making it harder for an attacker to predict the location of specific functions or buffers.
Stack Canaries: These are random values placed on the stack right before the return pointer. Before a function returns, the program checks if the canary value has changed (due to a buffer overflow). If it has, the program will terminate.
Safe Libraries: Use libraries that are designed to prevent buffer overflows. For example, prefer functions like strncpy() over strcpy() in C, though even “safe” functions can be misused.
Code Reviews & Static Analysis: Regularly review code to identify potential vulnerabilities and use automated tools to help with detection.
Threats
A threat is a potential cause of an unwanted impact on a system or application. It can exploit one or more vulnerabilities to achieve its objectives. Threats can be intentional (like hackers) or unintentional (like a natural disaster).
Characteristics of Threats
Origins: Threats can be external (originating outside the organization) or internal (originating from disgruntled employees, for instance).
Motive: Threats can be motivated by various reasons, such as financial gain, espionage, personal reasons, or even just for the thrill of it.
Magnitude: Threats can range from minor (causing brief disruption) to catastrophic (leading to significant data loss or infrastructure damage).
Examples
Phishing Attacks
Phishing attacks are a form of social engineering where cyber attackers attempt to deceive individuals into providing sensitive information, clicking on malicious links, or downloading harmful attachments, often by masquerading as a trustworthy entity.
How Phishing Works
Typically, an attacker sends a deceptive email, message, or communication that appears to come from a legitimate source (e.g., a bank, a reputable company, or even a colleague). The message might prompt the recipient to click on a link, download an attachment, or provide personal or financial information. These actions can lead to unauthorized access, financial loss, or malware infections.
Types of Phishing Attacks
Email Phishing: The most common form, where attackers send fraudulent emails to large numbers of people. These emails might contain links leading to malicious websites or attachments that can infect a user’s computer.
Spear Phishing: Targeted attacks against specific individuals or organizations. Attackers often gather detailed information about their targets to craft highly convincing messages.
Whaling: A type of spear phishing targeting high-level executives or important officials in an organization.
Vishing (Voice Phishing): Uses phone calls instead of emails. The attacker might pretend to be a bank representative, tech support, or any other legitimate call to extract sensitive information.
Smishing (SMS Phishing): Uses SMS or text messages to deceive recipients. Like vishing, it takes advantage of the trust people tend to have in text messages.
Pharming: Redirects users from a legitimate website to a fraudulent one without their knowledge or consent, often by exploiting vulnerabilities in DNS servers or manipulating a user’s hosts file.
Consequences of Phishing Attacks
Data Breach: Unauthorized access to personal and sensitive information, such as credit card details, social security numbers, or passwords.
Financial Loss: Direct theft of funds or unauthorized transactions.
Malware Infection: Downloaded malicious attachments or software can lead to ransomware infections, spyware, or trojans.
Loss of Reputation: Affected organizations can suffer significant damage to their reputation and trustworthiness.
Mitigation Techniques
Education & Training: Regularly educate employees about the risks of phishing and how to recognize suspicious messages.
Email Filtering: Use advanced email filtering solutions to detect and block phishing emails.
Multi-Factor Authentication (MFA): Even if an attacker obtains login credentials, MFA can prevent unauthorized access.
Regular Backups: In the case of ransomware infections through phishing, having backups ensures data can be restored without paying a ransom.
Updated Software: Ensure that all software, especially web browsers and email clients, are updated to protect against known vulnerabilities.
Secure Web Practices: Always look for ‘https’ in website URLs and be wary of website certificates that browsers flag as untrusted.
Phishing Simulation: Conduct regular phishing simulation tests to assess the organization’s vulnerability and raise awareness.
Distributed Denial of Service (DDoS) Attacks
A Distributed Denial of Service (DDoS) attack is a malicious attempt to disrupt the normal functioning of a targeted server, service, or network by overwhelming it with a flood of internet traffic from multiple sources.
How DDoS Works
Unlike a simple Denial of Service (DoS) attack, which originates from a single source, a DDoS attack involves multiple devices often distributed globally. These devices bombard the target with traffic, overwhelming its resources, and making it inaccessible to legitimate users.
Components of DDoS Attacks
Attackers: The individuals or groups orchestrating the assault.
Botmasters: Typically control multiple “bots” (compromised devices).
Botnets: A network of compromised computers or devices, controlled by botmasters, used to launch the coordinated attack.
Types of DDoS Attacks
Volume-based Attacks: Aim to saturate a target’s bandwidth. Examples include ICMP floods and UDP floods.
Protocol Attacks: Focus on exploiting vulnerabilities in a target’s resources, not necessarily its bandwidth. Examples include SYN floods, fragmented packet attacks, and Ping of Death attacks.
Application Layer Attacks: Target specific application services. These are often more subtle and aim to exhaust a server’s resources. Examples include HTTP GET/POST floods.
Consequences of DDoS Attacks
Service Disruption: The primary aim is to make services unavailable.
Financial Loss: For businesses that rely on online presence, such as e-commerce sites, downtime can lead to significant financial losses.
Damage to Reputation: Repeated attacks can damage an organization’s reputation, causing a loss of customers or users.
Distraction: Sometimes, attackers use DDoS as a diversion tactic to distract from another malicious activity elsewhere in the network.
Mitigation Techniques
Rate Limiting: This limits the number of requests a server will accept over a certain time window.
Web Application Firewalls (WAFs): These can detect and filter out malicious traffic.
Anomaly Detection: By monitoring network traffic, anomalies that could indicate a DDoS attack can be detected early.
DDoS Protection Services: Several third-party services specialize in detecting and mitigating DDoS attacks, rerouting traffic, and ensuring availability.
Redundant Infrastructure: Distributing resources across multiple data centers can help ensure availability even during an attack.
Intrusion Prevention Systems (IPS): These systems can be used to drop packets from suspicious IP addresses.
Blackholing: This involves directing the excessive traffic into a null route or “black hole.” While this also denies access to legitimate users, it can protect the overall integrity of the network.
Relationship Between Threats and Vulnerabilities
In the context of software security, threats and vulnerabilities are intrinsically linked. A threat actor can exploit a vulnerability to compromise the security of a system.
The relationship can be summarized as: Threats exploit Vulnerabilities to cause Harm.
For instance, if a software application has a vulnerability (like being susceptible to SQL injection), a threat actor (hacker) could exploit this vulnerability to access and steal sensitive data.
Mitigating Threats and Vulnerabilities
Regular Audits & Penetration Testing: Periodically checking the software or system for vulnerabilities can help in their early detection and rectification.
Patch Management: Keeping software and systems up-to-date ensures that known vulnerabilities are patched.
Training & Awareness: Many threats exploit human weaknesses, like susceptibility to phishing. Training employees can help mitigate such risks.
Intrusion Detection Systems (IDS) & Intrusion Prevention Systems (IPS): These can monitor and block suspicious activities in real-time.
Access Control: Limiting who can access what can reduce the risk of insider threats and prevent unwanted access.
Authentication and Authorization
Authentication is the process of verifying the identity of a user, system, or application.
Authentication
Components & Methods
Something You Know: This includes passwords, PINs, or answers to “security questions.” It’s the most common method but also susceptible to breaches if not managed correctly.
Something You Have: This refers to possession-based methods like smart cards, hardware tokens, or a code sent to a mobile device (often termed as OTP or One-Time Password).
Something You Are: Biometric methods fall under this category, including fingerprint scans, facial recognition, voice recognition, and iris scans.
Multi-Factor Authentication (MFA): This method requires users to provide multiple forms of identification from the categories mentioned above. For example, a banking app might require both a password and an OTP sent to a registered mobile number.
Authorization
Once authentication confirms the identity, authorization determines what that user, system, or application is allowed to do within the system.
Components & Methods
Access Control Lists (ACLs): These are tables that specify permissions for individual users or groups. They’re often used in file systems to control who can read, write, or execute a file.
Role-Based Access Control (RBAC): Instead of assigning permissions directly to individual users, they are grouped into “roles.” Users are then assigned roles. For instance, in a hospital system, the “Doctor” role might have different access than the “Nurse” role.
Attribute-Based Access Control (ABAC): This method uses policies to grant access based on the attributes of users, resources, and environmental conditions. It’s more dynamic and fine-grained than RBAC. For example, a user might only access certain data if they are from a specific department and are using a device within a company’s premises.
Token-Based Authorization: Once authenticated, the user might receive a token (like a JSON Web Token or JWT) that encapsulates their permissions. This token is then sent with subsequent requests to prove both identity and authorization.
Authentication and Authorization Relationship
While authentication and authorization are distinct processes, they are often used in tandem.
Sequential Process: Authentication typically occurs before authorization. Once a system establishes who you are (authentication), it then determines what you’re allowed to do (authorization).
Interdependence: Proper authentication is a prerequisite for effective authorization. Without accurate identification, permission checks have no substantive basis.
Token-Based Systems: As mentioned, in some systems, once a user is authenticated, they receive a token. This token often contains details about what the user is authorized to do, integrating the two processes.
Best Practices
Strong Authentication: Use strong password policies, enforce MFA, and ensure secure transmission of authentication data.
Principle of Least Privilege (PoLP): Grant users only the permissions they absolutely need to perform their tasks. This limits potential damage in case of breaches.
Regular Audits: Periodically review and update authentication and authorization configurations to match evolving needs and to remove any outdated or excessive permissions.
Use Established Protocols: When possible, utilize well-established authentication and authorization protocols and frameworks to avoid “reinventing the wheel” and potentially introducing vulnerabilities.
Data Encryption
Data encryption refers to the process of converting data (often called plaintext) into an unreadable format, known as ciphertext, using an algorithm and an encryption key. The primary goal is to ensure data confidentiality, so even if an unauthorized party intercepts the encrypted data, they won’t be able to understand its contents.
Components of Data Encryption
Algorithm: This is a set of mathematical procedures that transforms plaintext into ciphertext and vice versa. Examples include AES (Advanced Encryption Standard), RSA (Rivest–Shamir–Adleman), and DES (Data Encryption Standard).
Key: Encryption keys are secret values used in conjunction with the algorithm. The security of encrypted data largely depends on two things: the strength of the cryptographic algorithm and the secrecy of the key.
Types of Encryptions
Symmetric Encryption: Uses the same key for both encryption and decryption. This means both the sender and the receiver must have the key and keep it secret. AES is a commonly used symmetric algorithm.
Asymmetric Encryption: Uses a pair of keys: a public key for encryption and a private key for decryption. The public key can be shared openly, allowing anyone to encrypt data, but only the holder of the corresponding private key can decrypt it. RSA is a common example of asymmetric encryption.
Importance of Data Encryption in Software Security
Data Confidentiality: Encryption ensures that even if data is intercepted during transmission or stolen from storage, it remains confidential and unreadable to unauthorized parties.
Data Integrity: Some encryption methods can ensure that the encrypted data has not been altered during transmission.
Authentication: Asymmetric encryption can be used to verify the authenticity of a message sender.
Non-repudiation: With certain cryptographic methods, once a sender sends an encrypted message, they cannot deny sending it.
Secure Communication: Encryption is fundamental in secure communication protocols like HTTPS, SSL/TLS, and VPNs, ensuring data transferred between parties remains private.
Practical Applications of Encryption
Secure File Storage: Encrypting files on storage devices ensures they remain confidential even if the device is lost or stolen.
Secure Data Transmission: Protocols like SSL/TLS encrypt data as it’s transferred over the internet, protecting it from eavesdroppers.
Password Storage: Instead of storing user passwords in plaintext, systems often store a hash of the password, which is a form of one-way encryption.
Digital Signatures: Using encryption to verify the sender’s identity and ensure the integrity of the sent data.
Digital Rights Management (DRM): Encryption is used to control access to copyrighted digital media.
Considerations
Key Management: Maintaining the secrecy and integrity of encryption keys is crucial. Loss of the key can result in the loss of access to encrypted data, while unauthorized access to the key can lead to data breaches.
Performance: Encryption processes can be computationally intensive, potentially impacting system performance. Choosing the right algorithms and implementations is essential for balancing security and performance.
Regulatory & Compliance: Many industries and regions have regulations about how data should be encrypted, especially sensitive data like personal information or credit card details.
Backdoors: Intentional or unintentional backdoors in encryption can compromise data security. It’s crucial to use well-vetted cryptographic algorithms and software implementations.
Input Validation
Input validation is the process of inspecting data provided by users and external systems to verify that it adheres to the desired format, range, length, type, and other predefined criteria. If the input fails to meet these criteria, it’s either rejected or sanitized.
Why is Input Validation Important?
Preventing Malicious Attacks: Malicious users can exploit input fields to send harmful data to applications, potentially leading to attacks like SQL injection, cross-site scripting (XSS), and buffer overflow, among others.
Data Integrity: Validating input ensures that data remains accurate, consistent, and usable throughout an application.
User Experience (UX): Input validation can provide immediate feedback to users, guiding them to provide data in the correct format.
Reducing Error Handling Overhead: Correctly validating data upfront can reduce the necessity for error handling in downstream processes.
Types of Input Validation
Whitelisting vs. Blacklisting
Whitelisting: Only allowing known-good values. This is the more secure method since it denies everything that’s not explicitly allowed.
Blacklisting: Denying known-bad values. This is generally less secure since attackers can often find ways around blacklists.
Client-Side vs. Server-Side Validation
Client-Side: Validation occurs on the user’s device, typically using JavaScript. While this can improve UX by providing immediate feedback, it’s susceptible to tampering, so it should never be the sole validation mechanism.
Server-Side: Validation occurs on the server. This is more secure because it operates in a controlled environment, but it may require a round trip, impacting responsiveness.
Data Sanitization: Instead of outright rejecting malicious or malformed input, sanitization aims to “clean” the data, removing or replacing harmful portions.
Common Input Validation Techniques
Regular Expressions: Utilizing patterns to define and match allowable input.
Type Checks: Ensuring data matches the expected datatype, like string, integer, or date.
Range Checks: Ensuring numeric values or dates fall within an acceptable range.
Length Checks: Ensuring that input data adheres to minimum and/or maximum length constraints.
Lookup Tables: Checking input against a list of allowable values.
Pitfalls & Considerations
Relying Solely on Client-Side Validation: Client-side code can be modified by malicious users. Always ensure server-side validation.
Over-Reliance on Blacklisting: As mentioned, blacklisting is a weaker form of validation, and attackers can often bypass it.
Error Messages: Be careful with validation error messages. Revealing too much can provide attackers with insights into system architecture or validation logic.
Processing Overhead: Intensive validation processes, especially on large datasets, can introduce performance issues.
Escape, Not Reject: In some cases, especially with user-generated content, you might want to escape potential harmful characters (e.g., < or >) rather than outright reject the input.
Security Audits and Testing
Security Audits
A security audit is a systematic evaluation of the security of an organization’s information system. It measures how well the system conforms to a set of established criteria.
Key Components of Security Audits
Review of Policies and Procedures: Auditors will check if the organization has documented security policies and procedures and if they align with best practices.
Physical Security: Checking the physical environment where systems reside, ensuring unauthorized individuals can’t access critical infrastructure.
Access Control: Auditing who has access to what resources, ensuring the principle of least privilege is maintained.
Incident Response: Reviewing how an organization handles security incidents, from detection to resolution and subsequent analysis.
Outcomes
At the end of an audit, the organization usually receives a detailed report highlighting vulnerabilities, non-compliance issues, and recommendations for improvements.
Security Testing
While audits often review processes and policies, security testing is more hands-on. It’s about actively probing systems to discover vulnerabilities.
Types of Security Testing
Static Application Security Testing (SAST): Analyzes source code, bytecode, or binary code for vulnerabilities without executing the program. SAST is an automated approach to find security vulnerabilities. It involves using specialized tools that scan the source code, bytecode, or binary code of an application for patterns that suggest potential security flaws.
Dynamic Application Security Testing (DAST): Tests an application in its running state, often from an external perspective, to identify vulnerabilities exploitable during its operation.
Penetration Testing: A simulated attack on a system, often conducted by external experts, to identify vulnerabilities that could be exploited by attackers.
Security Code Review: A manual examination of source code to identify security-related issues. Security Code Review is a more manual process where human reviewers inspect the application’s source code to identify security issues. While tools might be used to aid the review, human expertise and judgment play a pivotal role.
Fuzz Testing: Feeding random, unexpected, or malformed inputs to software systems to discover coding errors and security loopholes.
Configuration Management Testing: Ensuring that configurations and deployment settings of applications and infrastructure are secure.
Threat Modeling: Identifying potential threats by understanding the system’s design and creating a model of potential attacks.
Outcomes
Security testing results in a list of vulnerabilities, their severity, potential impacts, and recommended mitigation strategies.
Importance of Security Audits and Testing
Risk Management: By identifying vulnerabilities, organizations can prioritize and manage risks effectively.
Regulatory Compliance: Many industries have regulations requiring periodic security audits and testing to ensure data protection and system integrity.
Trust and Reputation: Demonstrating a commitment to security can build trust with customers and stakeholders.
Continuous Improvement: Regular audits and testing encourage an organization to continuously refine its security posture.
Best Practices
Regular Scheduling: Both audits and testing should be conducted regularly, not just once. The threat landscape and technology environments evolve, necessitating periodic reviews.
Third-party Involvement: External experts can provide an unbiased perspective, often noticing issues that internal teams may overlook.
Remediation: After identifying vulnerabilities, it’s crucial to patch, remediate, or otherwise address them. Simply knowing about them isn’t enough.
Training and Awareness: Post-audit and testing, organizations should conduct training sessions to improve awareness and skill sets related to identified issues.
Updates and Patching
Updates
Generally, an update is a broad term that refers to any modification, enhancement, or correction made to software. This might involve fixing security vulnerabilities, but it can also encompass feature enhancements, performance improvements, and other refinements.
Patching
A patch is a set of changes applied to a software program, specifically designed to fix, or “patch up,” vulnerabilities and bugs. Patches are usually small and address specific issues, especially security-related ones.
Importance in Software Security
Vulnerability Remediation: As new vulnerabilities are discovered, patches can rectify them, preventing potential exploitation by malicious actors.
Protection Against Known Threats: Many cyberattacks target known vulnerabilities for which patches are available but haven’t been applied by users.
Functional Stability: Apart from security fixes, updates can address software bugs that cause instability or crashes.
Regulatory Compliance: In regulated industries, applying security patches within a certain timeframe may be mandated to ensure the safety of data.
Maintaining Trust: Regular updates show a commitment to security and can foster trust with users and stakeholders.
Challenges
Compatibility Issues: Sometimes, patches might cause issues with other software or systems, leading to new bugs or incompatibilities.
Downtime: Applying patches, especially to critical systems, might require downtime, which can be disruptive.
Overhead: For large organizations, the sheer volume of patches across numerous software solutions can be daunting.
Missed Patches: With many software tools in use, it’s easy to overlook a critical patch, leaving a vulnerability unaddressed.
Potential Bugs: New updates can sometimes introduce new vulnerabilities or bugs.
Best Practices
Patch Management: Establish a systematic approach to monitor, test, and apply patches. This involves prioritizing patches based on the criticality of vulnerabilities they address.
Automated Tools: Use tools that can automatically detect and deploy patches, streamlining the process.
Testing: Before rolling out a patch system-wide, test it in a controlled environment to ensure it doesn’t introduce new issues.
Regular Monitoring: Stay informed about new vulnerabilities and patches by subscribing to vendor notifications, security bulletins, and other reliable sources.
Backup Before Patching: Ensure you have recent backups before applying patches. If something goes wrong, you can restore your system to its previous state.
User Education: Educate users about the importance of updating and patching, especially if they are responsible for updating their devices or software.
Scheduled Updates: For non-critical software, schedule updates during off-hours to minimize disruptions.
End-of-Life Software: Be wary of using software that’s no longer supported by its vendor, as it won’t receive security patches.
Software security remains a paramount concern in our increasingly digital world, where the intricacies of code intertwine with the fabric of daily life and business operations. As threats evolve in complexity and scale, the need for rigorous, proactive measures in software design, development, and maintenance becomes ever more pressing.
Ensuring software security is not merely about thwarting attacks but about safeguarding trust, upholding data integrity, and guaranteeing operational resilience in the face of adversity. Ultimately, a commitment to software security is a testament to an organization’s dedication to its users, stakeholders, and the broader digital ecosystem.