Software architecture is a critical aspect of the software design process that focuses on defining the high-level structure and organization of a software system. It serves as a blueprint that outlines how different components of the system will interact, how data will flow between them, and how the system will achieve its functional and non-functional requirements.
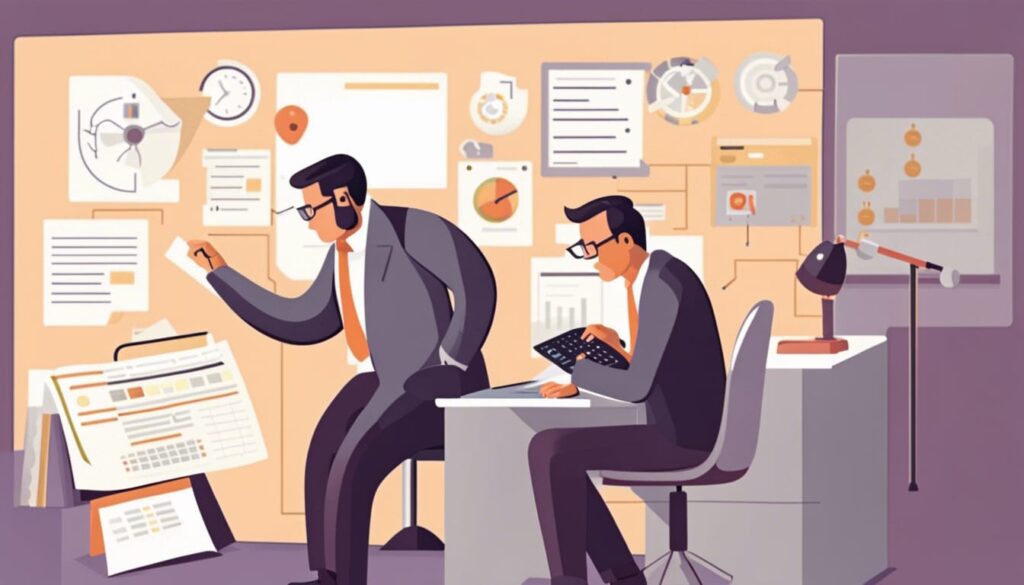
Components and Modules
Components and modules are foundational elements in software architecture, playing a critical role in system design, organization, and manageability. Understanding them is key to building scalable, maintainable, and modular software systems.
Components
A component is a self-contained unit that encapsulates a specific functionality or set of functionalities within a system. It hides the inner workings and exposes a clear interface for other components to interact with. Components often represent runtime entities, meaning they can be thought of as distinct pieces of a system that interact with each other at runtime.
Characteristics of Components
Encapsulation: Components conceal their internal logic, revealing only what’s necessary for interaction.
Reusability: Designed to be reused in different contexts or projects.
Independence: Can often operate independently, communicating through well-defined interfaces.
Interchangeability: If a component adheres to a specific contract or interface, it can often be replaced with another component that adheres to the same contract without affecting the overall system.
Usage: Components are prevalent in component-based software engineering (CBSE) and often found in modern frameworks and platforms like Angular, React, and .NET.
Modules
A module is a logical partition of the software where related classes, functions, and data are grouped together. Unlike components, modules are more about organization at the code level and don’t necessarily represent runtime entities. Modules help in breaking down a complex software system into manageable chunks.
Characteristics of Modules
Cohesion: Elements within a module are closely related and work towards a common function or feature.
Separation of Concerns: Each module typically focuses on a specific aspect or concern of the software, ensuring that unrelated functionalities don’t get mixed up.
Encapsulation: Like components, modules hide their internal workings, exposing only necessary functionalities.
Ease of Maintenance: With functionality logically grouped, making changes, or debugging becomes more straightforward.
Usage: Modules are common in many programming paradigms and languages. For instance, in Python, you can have .py files acting as modules, and in JavaScript (especially ES6 and beyond), you can use the import and export statements for module functionalities.
Relationship
While there is a clear distinction between components and modules, they often work hand in hand. For example, a component, in terms of runtime behavior, may be made up of several modules. Modern software often employs both modular design (at the code level) and component-based architecture (at the system design and runtime level).
Conclusion
Components and modules are architectural strategies to manage complexity, improve reusability, and enhance maintainability in software design. By breaking down software into distinct units (whether that’s runtime-based components or code-based modules), architects and developers can ensure clearer responsibilities, easier debugging, and more scalable structures. When used effectively, these elements enable teams to build robust, modular, and adaptable software systems.
Data Flow
In software architecture, data flow pertains to the movement, transformation, and management of information within a system. Understanding data flow is essential for designing efficient, reliable, and secure systems. Data flow refers to the path or route data takes as it moves through a system – from its point of entry, through processes or transformations, and finally to its point of exit or storage.
Elements of Data Flow
Data Sources: Where the data originates. This could be user input, sensors, external systems, databases, etc.
Processes: Actions that transform or manipulate the data. For instance, calculating, filtering, or aggregating.
Data Storage: Places where data rests or is persisted. Examples include databases, caches, logs, and file systems.
Data Sinks: Where data might end up after being processed. This could be displays (for user consumption), external systems, archives, or other storage mediums.
Data Channels: The pathways or mechanisms by which data moves. This can involve APIs, network protocols, data buses, and more.
Characteristics of Data Flow
Directionality: Data can flow in various directions – unidirectional, bidirectional, or multidirectional.
Volume: Systems can experience varying amounts of data flow, from sporadic, low-volume flows to constant, high-volume streams.
Transformation: As data flows, it might undergo multiple transformations, often dictated by the processes it encounters.
Latency: The time it takes for data to traverse from one point to another in the system.
Importance of Data Flow in Software Architecture
Performance: Understanding data flow helps architects design efficient pathways, ensuring swift data processing and minimal latency.
Scalability: Architects can identify potential bottlenecks or high-volume areas and design the system to scale as needed.
Security: Mapping data flow is vital for understanding which points need to be secured, implementing proper encryption, and ensuring data integrity.
Reliability: By analyzing data flow, architects can implement redundancy, fault tolerance, and error-handling mechanisms to ensure continuous data processing even when parts of the system fail.
Maintainability: A clear map of data flow simplifies troubleshooting, as developers can trace data pathways when debugging.
Visualization
Data flow is often visualized using Data Flow Diagrams (DFDs), which graphically represent the flow of data within a system, including sources, processes, storage, and sinks.
DFDs help in identifying how data moves and transforms, in spotting redundancies or inefficiencies, and in making informed architectural decisions based on data pathways.
Conclusion
Data flow is a fundamental aspect of software architecture, deeply influencing the system’s performance, security, reliability, and maintainability. By meticulously mapping and understanding data flow, architects can create systems that are efficient, secure, and resilient, catering to the dynamic and often demanding needs of modern applications.
Scalability and Performance
Scalability and performance are pivotal aspects of software architecture, especially in today’s demanding digital ecosystem where user expectations, data volumes, and concurrent access can vary greatly.
Scalability
Scalability refers to the capability of a system, process, or network to handle growing amounts of workload or its potential to expand to accommodate that growth.
Types of Scalabilities
Horizontal Scalability (Scale Out): Involves adding more nodes to a system. For instance, adding more machines to a cluster. It’s often associated with distributed architectures like microservices.
Vertical Scalability (Scale Up): Refers to adding more resources to a single node. For example, increasing the RAM or CPU of an existing server.
Importance of Scalability in Software Architecture
Accommodating Growth: As user base or data volume grows, the system should scale seamlessly without extensive redesign.
Cost Efficiency: Scalable systems can adjust resources based on demand, which can be more cost-effective than always maintaining peak capacity.
Resilience: Horizontal scaling, especially in distributed systems, can enhance resilience by reducing single points of failure.
Performance
Performance, in software architecture, gauges the responsiveness of a system. It measures how effectively a system uses resources to fulfill requests within an acceptable timeframe.
Factors Influencing Performance
Latency: The time it takes to process a single operation or request.
Throughput: The number of operations or requests a system can handle per unit of time.
Bandwidth: The volume of data that can be processed or transmitted in each time frame.
Efficiency: How well resources (CPU, memory, storage) are utilized during operations.
Importance of Performance in Software Architecture
User Experience: Poor performance can directly impact user satisfaction and retention.
Resource Management: Efficient systems make better use of hardware and software resources, reducing operational costs.
Reliability: Systems performing near their limits might have reduced reliability and are more prone to errors or crashes.
Interplay between Scalability and Performance
While scalability and performance are distinct concepts, they often intersect.
Balancing Act: As systems scale (especially vertically), performance might increase since there’s more computational power. However, past a certain point, adding more nodes or resources might introduce overhead, affecting performance.
Bottlenecks: Scaling a system can reveal performance bottlenecks. For instance, a database might become a limiting factor if not designed for high concurrency, even if other parts of the system scale well.
Architectural Considerations: Some architectural patterns emphasize scalability (e.g., stateless microservices) while others might prioritize performance (e.g., high-performance computing clusters). Often, architects must find a balance based on the specific needs of an application or system.
Conclusion
Scalability and performance are foundational pillars of effective software architecture. Architects must be adept at foreseeing potential bottlenecks, growth trajectories, and performance needs, employing patterns and practices that allow for both scalability and high performance.
Flexibility and Extensibility
Flexibility and extensibility are vital attributes in software architecture, especially in an ever-changing technological landscape where systems need to adapt to new requirements, technologies, or unforeseen challenges.
Flexibility
Flexibility in software architecture refers to the ability of a system to easily adapt to variations in its operational environment without causing significant changes in the existing infrastructure or architecture.
Characteristics & Implications
Adaptability: A flexible system can accommodate changes, such as different configurations or operational conditions, with minimal effort.
Configurability: Flexible architectures often allow for configurability without altering the core codebase. This might be through external configuration files, environment variables, or runtime parameters.
Loose Coupling: Systems designed for flexibility tend to have components that are loosely coupled. This means changes in one component don’t ripple extensively through others.
Reduction in Technical Debt: A flexible architecture can better accommodate future changes, thus avoiding quick fixes that lead to technical debt.
Extensibility
Extensibility is the ability of a software system to incorporate new functionalities or components with minimal impact on existing system components. This often involves anticipating future growth areas.
Characteristics & Implications
Pluggable Modules: Extensible systems might use plugins or modules to add new features. For instance, many content management systems (CMS) allow for plugins to extend functionality.
Open Interfaces/APIs: An extensible system often provides APIs or interfaces that allow other systems or modules to integrate with it, facilitating addition of new features.
Forward Compatibility: Such systems can accommodate future versions or additions without breaking the existing functionalities.
Maintainability: While extensibility can lead to more complex initial designs, it often results in easier long-term maintainability since new features can be added without major system overhauls.
Relationship & Distinction
While flexibility and extensibility might seem similar, their focus differs.
Focus of Change: Flexibility deals with adapting to different conditions or configurations without changing the system’s core. Extensibility, on the other hand, focuses on adding new features or functionalities.
Anticipation of Change: Flexibility is often about handling unforeseen changes gracefully, while extensibility involves a degree of foresight, anticipating areas where future features or modules might be added.
Importance of Flexibility and Extensibility in Software Architecture
Longevity of Systems: Flexible and extensible architectures ensure that systems remain relevant and adaptable over time, prolonging their utility.
Cost-Efficiency: Making changes to a flexible and extensible system is typically less costly than overhauling rigid systems.
Competitive Edge: Businesses with flexible and extensible systems can more rapidly adapt to market changes, customer demands, or new technologies, maintaining a competitive advantage.
Conclusion
As technology and user needs evolve, systems that embody these principles are better equipped to evolve alongside, ensuring sustained value, relevance, and functionality. Investing in flexible and extensible design not only future-proofs a system but also encapsulates a proactive, adaptive, and resilient approach to software development.
Separation of Concerns
Separation of Concerns (SoC) is the practice of splitting a software application into distinct parts, with each part addressing a separate concern. A “concern” in this context refers to a specific functionality, feature, or area of interest within an application.
Characteristics & Implications
Modularity: Implementing SoC often results in a modular architecture, where each module is responsible for a specific concern.
Encapsulation: Each segregated part encapsulates its functionality and hides the complexity from other parts.
Reusability: Components or modules developed with SoC in mind tend to be reusable across various parts of the application or even other applications.
Maintainability: Isolated concerns are easier to understand, modify, and test. A change in one module has limited impact on others, reducing the chances of unintended side effects.
Examples in Software Systems
MVC (Model-View-Controller)
This design pattern, commonly used in web applications, separates concerns into three interconnected components:
Model: Represents the data and business logic.
View: Manages the display of information.
Controller: Handles user input and updates the Model and View accordingly.
Layered Architecture
In this approach, software is divided into layers, each with its own responsibility. Common layers include:
Presentation Layer: User interface and user experience components.
Business Logic Layer: Application’s core functional logic.
Data Access Layer: Interactions with databases or other storage mechanisms.
APIs and Microservices
APIs abstract and separate the functionalities they offer, and microservices architecture breaks down applications into small services that run independently, with each addressing a specific business capability.
Importance of Separation of Concerns in Software Architecture
Simplified Development: Developers can focus on specific concerns without being overwhelmed by the entire system’s complexity. This can expedite development and reduce errors.
Enhanced Collaboration: In larger teams, developers can work on separate concerns simultaneously without causing much interference to one another.
Easier Testing: With clear separation, unit testing becomes more straightforward since each concern can often be tested independently.
Scalability: In architectures like microservices, concerns separated into different services can scale independently based on their individual demands.
Flexibility in Technology Choices: When concerns are properly separated, it’s possible to use different technologies for different parts of the system without affecting the entire architecture.
Conclusion
By emphasizing the isolation of responsibilities, SoC fosters a design approach where software components are more understandable, manageable, and adaptable. Whether one is building a small application or a large-scale enterprise system, adhering to the SoC principle can lead to more robust, maintainable, and scalable solutions, ensuring that the architecture remains resilient in the face of evolving requirements and challenges.
Error Handing and Exception Handling
Error handling and exception handling are crucial components of software development that address unexpected situations and errors that can occur during program execution. These mechanisms help ensure that software applications handle errors gracefully, recover from unexpected scenarios, and provide meaningful feedback to users.
Error Handling
Definition: Error handling involves detecting and managing errors, anomalies, and unexpected conditions that may occur during the execution of a program.
Types of Errors: Errors can be categorized into syntax errors (code violations), runtime errors (occurs during program execution), and logical errors (flaws in program logic that produce incorrect results).
Logging and Reporting: Logging mechanisms record error details, allowing developers to analyze issues and troubleshoot problems. Error messages and reports also help users understand what went wrong.
Graceful Degradation: Error handling aims to prevent complete application crashes by implementing mechanisms that allow the software to continue functioning despite encountering errors.
Exception Handling
Exceptions are specific types of errors that occur during runtime and disrupt the normal flow of the program. Exception handling is a mechanism to catch and manage these exceptions.
Try-Catch Blocks
In languages with exception handling, developers use try-catch blocks to encapsulate code that might cause exceptions. The try-catch block is a primary mechanism to handle exceptions in many programming languages like Java, C#, JavaScript, Python, etc.
How it works
Try Block
The code that might produce an exception is enclosed within a try block. If an exception occurs within the try block, the program’s control is immediately transferred to an associated catch block.
Catch Block
The code inside the catch block is executed when an exception occurs. Here, the developer can handle the exception, either by providing a fallback mechanism, logging the error, informing the user, or taking any other remedial action. Different types of exceptions can be caught and handled differently by using multiple catch blocks.
Finally Block
Many languages offer a finally block that can be attached to a try-catch. This block is executed irrespective of whether an exception occurs. It is mainly used for cleanup activities like closing files or releasing resources.
Throw/Throws
These keywords can be used to manually trigger exceptions or indicate that a method might produce a specific exception that callers should be prepared to handle.
Advantages of Try-Catch
Improved User Experience: Without exception handling, users might experience abrupt application terminations. With it, you can offer more user-friendly error messages and potential solutions.
Easier Debugging: Proper exception handling with logging can help developers pinpoint where things are going wrong.
Robustness: Applications become more stable by handling exceptional scenarios gracefully.
Points to Consider
Overusing Try-Catch: Excessive use of try-catch can lead to code that’s hard to read and maintain. Instead of using it everywhere, use it in places where exceptions are genuinely unexpected.
Performance: There’s a minor overhead associated with try-catch. While negligible in most cases, in performance-critical sections of code, this might be a concern.
Specificity: It’s generally a good idea to catch only those exceptions you know how to handle. Catching all exceptions generically can mask genuine bugs.
Throwing Exceptions: Developers can throw exceptions when they detect errors or unusual conditions. This allows code higher up in the call stack to catch and handle the exception.
Hierarchy of Exceptions: Many programming languages have a hierarchy of exception classes, ranging from generic to specific. This allows for fine-grained handling of different types of exceptions.
Best Practices for Error and Exception Handling
Provide Clear Messages: Error messages should be informative and understandable to users, helping them diagnose and address issues.
Log Errors: Implement robust logging to capture error details for analysis and troubleshooting.
Fail Safely: Design code to fail gracefully and recover whenever possible, rather than causing system crashes.
Avoid Silent Failures: Don’t allow errors to go unnoticed. Provide users with feedback when something goes wrong.
Specificity: Catch exceptions at the appropriate level of abstraction and handle them in a way that is specific to the error context.
Resource Cleanup: In languages with resource management (like closing files or database connections), ensure proper cleanup even if an exception occurs.
Importance of Error and Exception Handling
User Experience: Well-handled errors provide users with meaningful feedback, preventing confusion and frustration.
Reliability: Effective error and exception handling prevents unexpected crashes and ensures that the application remains stable and reliable.
Debugging: Proper error handling aids in diagnosing issues during development and testing phases.
Security: Thorough error handling prevents vulnerabilities and exploitation of software weaknesses.
Importance of Software Architecture
System Understanding: A well-defined architecture provides a clear view of how the software operates, aiding developers, testers, and other stakeholders in understanding the system’s structure and behavior.
Quality Assurance: A solid architecture helps ensure that the system meets quality attributes like reliability, performance, and security.
Team Collaboration: Architects guide the development team by providing guidelines and best practices for implementation, fostering consistent coding practices and collaboration.
Long-Term Maintenance: A good architecture contributes to a maintainable system by making it easier to modify, update, and fix issues as the software evolves.
In summary, software architecture is the foundation upon which the entire software system is built. It’s a strategic decision that significantly impacts the success of a project by guiding how components interact, how data flows, and how the system as a whole meets its goals and requirements.