To manage our project implementation we will use the Personal Kanban methodology. Personal Kanban is a visual task management system that allows individuals to organize and prioritize their tasks in a flexible and intuitive manner.
Drawing inspiration from the Kanban system used in lean manufacturing, Personal Kanban typically utilizes a board with columns labeled “To Do,” “In Progress,” and “Done” to represent the flow of tasks. Users place tasks, often written on sticky notes, in the relevant columns and move them as they progress.
This approach provides a clear snapshot of one’s workload, helps in managing task flow, and fosters a sense of accomplishment as tasks move to the “Done” column.
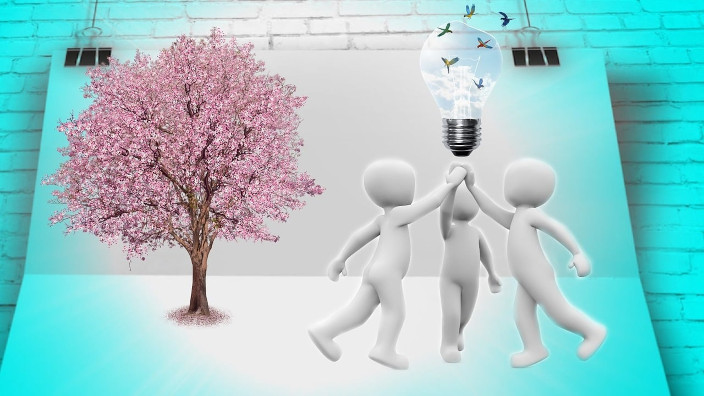
Review & Preparation
Review GDD
Read GDD to re-familiarize with the core mechanics, objectives, and game concepts.
Review TDD
Read TDD understand the technical implementations, systems, and tools proposed.
Create and Prepare Unity Project
Create a 2D Core Unity Project. This is an empty project configured for 2D apps. It uses Unity’s built-in renderer.
Add the following tags to your project: Racquet, BallSplit, Barrier, LightningBolt, PlayerGoalpost, and AIGoalpost.
Get/Create Assets
Get/Create the Visual Assets
Start scene background: A colored image (1920×1080).
Main scene background: A black image (1920×1080).
Default power-up icon: a 512×512 image, utilized when no power-up is active.
Thunder icon: a 512×512 image, representing the lightning bolt power-up activation.
Atomic energy icon: a 512×512 image, signifying the activation of the split ball power-up.
Shield icon: a 512×512 image, indicating the barrier power-up’s activation.
Logo: intended for display in the start scene.
Create the player’s paddle sprite.
Create the AI’s paddle sprite.
Create the ball sprite.
Get/Create the Audio Assets
Sound Effects (SFX)
Ball Bounce: A sound FX that plays when the ball collides with the paddles.
Player Scores: A cheerful sound FX that plays when the player scores a point.
AI Scores: A disheartened sound FX that plays when the AI scores a point.
PowerUp: A sound FX that triggers upon power-up activation.
Countdown: A countdown sound FX that plays before the ball starts moving, allowing players time to prepare.
Get/Create the Music
Background Music: A looping track that plays during gameplay. Ideally, something light and not too distracting.
Create the Power-Up Prefabs
Lightning Bolt: a prefab, depicted as a sprite (thunder icon) with a Circle Collider 2D, increases the ball’s speed.
Lightning bolt power-up animation : Create the animation and add it to the lightning bolt prefab. (Animate the x and y positions of the lightning bolt power-up to simulate a dynamic shaking movement.)
Ball Split: a prefab, represented as a sprite (atomic energy icon) with a Circle Collider 2D, introduces a second ball to the play area.
Split Ball power-up animation: create the animation and add it to the split ball prefab. (Animate the z-axis rotation of the split ball power-up to create a spinning effect.)
Barrier : a prefab, illustrated as a sprite (shield icon) with a Circle Collider 2D, activates the player’s barrier to protect the goalpost.
Barrier power-up animation: create the animation and add it to the barrier prefab. (Animate the x and y scaling values of the barrier power-up to simulate a zoom effect.
Get/Create the Scenes & UI Elements
Create the Start Scene
Add the background image.
Main Menu: It features three buttons: “Play,” which starts the game; “Settings,” which opens the settings menu; and “Exit,” which closes the game.
Settings Menu. It includes three toggles to adjust difficulty (easy, normal, and hard), as well as two toggles and two sliders to mute and adjust the volume of sound FX and music, respectively.
Total Score Panel. It features two text UI elements that display the total scores for both the player and the AI from all the games they’ve played.
Credits Panel: It displays details crediting the graphics, sounds, and music used in the game.
Add the scene features the company logo and URL.
Create the End Scene
Add the background image.
Design the end-game scene, which includes two text UI elements displaying the winner and the score and two buttons. Additionally, there are two buttons that allow the player to either play again or return to the main menu.
Create the Game Scene
Add the background image, which is a simple black sprite equipped with a Box Collider 2D, representing the court where the game is played.
Create the text UI elements displaying the player’s score and the opponent’s score, as well as an image UI element showing the active power-up.
Create the top and bottom boundaries, depicted as simple black sprites with Box Collider 2D components, which confine the ball’s movement.
Create the goalposts for both the player and the opponent, equipped with Box Collider 2D components, which trigger scoring upon collision with the ball.
Create the player barrier, represented by a translucent sprite with a Box Collider 2D. When activated by the barrier power-up, it protects the player’s goalpost.
Create the player’s paddle, a sprite (color: #94C18A) endowed with both a Rigidbody 2D and a Box Collider 2D, is player-controlled.
Create the opponent’s paddle, another sprite (color: #6B3E75) with a Rigidbody 2D and a Box Collider 2D, is managed by the AI.
Create the ball, illustrated as a sprite (color: #DAA520) with a Rigidbody 2D and a Circle Collider 2D, moves continuously back and forth across the court.
Development & Implementation
Create the GameState
Use Game State Management section in the TDD to create the GameState, a static class that houses game data and provides the required functionality.
Create the Scripts
Refer to the pseudo-code given in the ‘scripting details’ section of the TDD to create the scripts. It’s important to note that our approach here diverges slightly from standard practice. The scripts are sequenced based on their interdependencies. If followed in this order, you can develop each script without needing to integrate features from other scripts.
Often, this isn’t the usual scenario. The more common strategy involves starting with the core features and progressively filling in the gaps. However, in this instance, we’ve had the advantage of organizing our tasks in a manner that simplifies the process for us.
Create the MainMenuFunctions script.
Create the UpdateTotalScore script.
Create the EndGameMenuFunctions script.
Create the UpdateUI script.
Create the MusicManager script.
Create the SoundManager script.
Create the PauseManager script.
Create the InGameMenuFunctions script.
Create the SecondBallController script.
Create the PowerUpSpawner script.
Create the PowerUpDisplayManager script.
Create the PlayerController script.
Create the AIPredictiveTracking script.
Create the BallController script.
A Few Words about the Implementation Approach
Grouping Scripts
We can divide the scripts based on their functionality into three main groups:
Primary group: It contains the scripts that implement the core gameplay mechanic of two paddles hitting a ball. This group includes the following scripts: PlayerController, AIPredictiveTracking, and BallController.
Secondary group: It contains the scripts that implement the secondary gameplay mechanic, which is the power-ups system. This group consists of the following scripts: SecondBallController, PowerUpSpawner, and PowerUpDisplayManager.
Peripheral group: It contains the scripts that implement peripheral systems enhancing the gameplay, such as menus, sound effects and music, UI, etc. This group includes scripts like MainMenuFunctions, UpdateTotalScore, EndGameMenuFunctions, UpdateUI, MusicManager, SoundManager, PauseManager, and InGameMenuFunctions.
Your Decision
If you feel confident using Unity and writing C# scripts, you can follow an approach that more closely mirrors a real-world development process. Start with the primary group that implements the core logic of the game. Then, implement the secondary group of scripts to add the power-ups system. Finally, complete the peripheral group.
The advantage of this approach is that you can begin with an iterative process early on, taking small steps and testing your game quickly. The downside is that you may need to jump between scripts, adding functionality, which might introduce more bugs.
Choose your approach based on your objective. If you want to learn Unity and scripting, follow the simpler approach. By implementing the scripts one by one, you can focus on Unity development aspects. If you aim to gain deeper insights into the game development process, opt for the latter approach, but be prepared for a slightly steeper learning curve.
Regardless of which approach you choose; my advice is to develop the game using Test-Driven Development (TDD). Try to utilize the pseudo-code provided and craft your own script implementations. You can refer to the code provided below to assist you if you get stuck. While copying and pasting this code will speed up the implementation, it may slow down the learning process.
Testing & Iteration
Depending on the approach you decide to take, your testing will differ. If you implement the scripts one by one, you will need to focus your testing each time you complete a function or a script, ensuring that everything still works as intended. If you opt for the latter approach and start implementing the scripts based on their group (primary, secondary, and peripheral), your testing should be centered on the game logic.
As you introduce new game logic, such as controlling the player, the basic movement of the ball, or the AI logic, ensure that everything operates as expected. Regardless of the approach you choose, test as early and as frequently as possible.
Please remember that testing is not the same as debugging. While it’s crucial to find and eliminate bugs in your game—ensuring that your code functions correctly—it doesn’t guarantee that your game is enjoyable. Try to playtest your game to ensure its engaging. If you find yourself smiling or even laughing while playing, there’s a good chance you’re on the right track, and others will likely enjoy your game as well. Engaging others to playtest your game is even more beneficial.
Publishing the Game
When your game is ready, it’s time to present it to its true owners: the players. Game development is more than just a technical endeavor; it’s a form of art. The game developer has a vision which they aim to manifest into something tangible for others to experience.
Regardless of whether you feel confident or uncertain about your creation, give it a shot and publish it on a platform like itch.io. Once you’ve done that, you can proudly claim to have a game under your belt. You are now a game developer!
Indicative Schedule
Here, you’ll find a breakdown of the project into manageable tasks. In implementing the Personal Kanban method, we require a board divided into three columns: “To Do,” “In Progress,” and “Done” to visualize the flow of tasks. This board can be set up using software such as Trello or simply with post-it notes on a wall.
The crucial aspect is breaking the project down into manageable chunks. Since we cannot define an ‘average developer’, no estimated time for completing a chunk will be provided. Depending on your level, chunks might take a day, a week, or even a month. What’s important is not the duration, but to follow through and finish the process until you publish your game.
View the ‘Project Management’ section for details on each task in the chunks.
Chunk 1 – Review & Preparation
Review GDD and TDD. Create and Prepare Unity Project.
Chunk 2 – Get/Create the Assets
Get/Create the Images and the Icons.
Get/Create the Sprites.
Get/Create the Sound Effects (SFX).
Get/Create the Music.
Create the Power-Up Prefabs.
Create the animations for the power-ups.
Chunk 3 – Create the Start Scene
Design the main menu.
Design the settings menu.
Design the total score panel.
Design the credits panel, the company logo and URL.
Chunk 4 – Create the End Scene
Design the end-game menu.
Create the animations for the end-game UI.
Create the Game Scene
Chunk 5 – Create the game environment.
Create the score and the active power-up UI.
Create the top and bottom boundaries.
Create the goalposts.
Create the player barrier.
Chunk 6 – Create the game actors.
Create the player’s paddle.
Create the opponent’s paddle.
Create the ball.
Development & Implementation
Chunk 7 – GameState
Implement the GameState static class.
Scripts
Chunk 8 – Peripheral Group
Implement the MainMenuFunctions script.
Implement the UpdateTotalScore script.
Implement the EndGameMenuFunctions script.
Implement the UpdateUI script.
Implement the MusicManager script.
Implement the SoundManager script.
Implement the PauseManager script.
Implement the InGameMenuFunctions script.
Chunk 9 – Secondary Group
Implement the SecondBallController script.
Implement the PowerUpSpawner script.
Implement the PowerUpDisplayManager script.
Chunk 10 – Primary Group
Implement the PlayerController script.
Implement the AIPredictiveTracking script.
Implement the BallController script.
Chunk 11 – Testing and Playtesting the Game
Test and playtest you game. Implement the feedback.
Chunk 12 – Publishing the Game
Publish your game.