In the fourth installment of our comprehensive exploration into programming language elements, we shift our focus to the critical topic of expressions. Expressions form the backbone of nearly every program, as they are the building blocks for performing computations, making decisions, and controlling the flow of code execution.
Expressions, in essence, are combinations of values, variables, operators, and functions that, when evaluated, yield a result. They can be as simple as a single numeric value or as complex as a multi-part mathematical formula or a combination of logical conditions. Understanding how to create, manipulate, and leverage expressions is a foundational skill for any programmer.
A thorough grasp of expressions is crucial for writing code that not only performs computations accurately but is also readable and maintainable. Expressions are the means through which you communicate your program’s logic and functionality, making them a fundamental part of the programming language landscape.
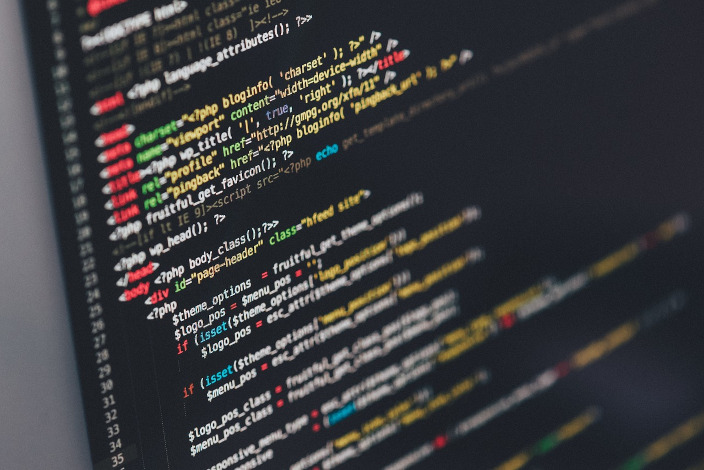
Expression Types
Expression types in programming encompass the diverse forms and structures that expressions can take, each tailored to fulfill specific purposes and perform distinct operations.
These types serve as the building blocks for constructing meaningful and efficient code sequences, enabling developers to articulate their intentions and achieve desired outcomes with precision.
Arithmetic expressions involve mathematical operations such as addition, subtraction, multiplication, and division. Relational expressions are another crucial expression type used to compare values. Logical expressions, on the other hand, are instrumental in decision-making within programs. Conditional expressions, including the ternary operator, provide a concise way to express conditional logic within a single expression.
Furthermore, understanding the use of parentheses in expressions is paramount. Parentheses not only control the order of evaluation but also help clarify the intended precedence of operations, ensuring that complex expressions are computed accurately.
Arithmetic Expressions
Arithmetic expressions are the backbone of mathematical computations in programming, enabling developers to perform a wide range of numeric operations within their code. These expressions consist of operands (numeric values or variables) and operators (symbols that represent mathematical actions).
Developers can use arithmetic expressions to perform addition, subtraction, multiplication, division, and other mathematical calculations with ease and precision. Arithmetic expressions are not limited to basic operations; they can also include more complex calculations, such as exponentiation and modular arithmetic.
Additionally, developers often use parentheses to control the order of operations within expressions, ensuring that calculations occur according to the desired precedence. Understanding and constructing arithmetic expressions are essential skills for any programmer, as they are employed in various domains, from financial calculations and physics simulations to game development and data analysis. Mastery of arithmetic expressions is fundamental to writing efficient and accurate software.
In programming, arithmetic expressions go beyond mere number crunching; they serve as integral components of broader algorithms, decision-making processes, and simulations. Whether it’s calculating financial interest, determining the dimensions of graphic elements, or simulating real-world phenomena, arithmetic expressions empower developers to bring their computational ideas to life with precision and efficiency.
Relational Expressions
Relational expressions play a pivotal role in programming by enabling developers to compare values and establish relationships between data elements. These expressions are fundamental for decision-making within software applications.
Relational expressions use relational operators, such as less than (<), greater than (>), equal to (==), and not equal to (!=), to evaluate the truth or falsity of a given condition. This evaluation outcome is crucial for controlling program flow, making decisions, and filtering or sorting data.
In addition to basic relational operators, programming languages often provide other operators that extend the functionality of relational expressions. For example, “less than or equal to” (<=) and “greater than or equal to” (>=) operators allow for inclusive comparisons.
These expressions are used extensively in various programming tasks, such as searching for specific elements in a dataset, sorting items based on specific criteria, and validating user input against predefined conditions. A firm grasp of relational expressions is essential for writing code that can efficiently and accurately handle these common programming scenarios.
Furthermore, relational expressions are integral to the creation of conditional statements and loops, which are the building blocks of decision-making in software. Conditional statements, like “if-else” constructs, rely on the outcome of relational expressions to determine which branch of code to execute.
Loops, such as “while” and “for” loops, often employ relational expressions as conditions to control the iteration process. In both cases, relational expressions serve as the logical foundation that guides program behavior, making them a crucial concept for any programmer to understand and employ effectively.
Logical Expressions
Logical expressions are essential components of programming that enable developers to make decisions and control the flow of their code based on conditions. These expressions involve logical operators such as AND, OR, and NOT, which allow programmers to evaluate the truth or falsity of one or more conditions.
They are particularly valuable for branching, where different code paths are executed depending on the outcome of these evaluations. For example, in an “if” statement, a logical expression determines whether a certain block of code should be executed based on whether the condition is true.
Logical expressions are not limited to simple true or false outcomes. They can involve complex combinations of conditions, nested expressions, and multiple logical operators. The ability to construct and evaluate these expressions accurately is crucial for creating robust and responsive software.
Moreover, logical expressions are essential for creating loops that iterate through data until a specific condition is met, making them a cornerstone of iterative algorithms and repetition structures in programming.
Understanding logical expressions and their operators is essential for writing code that makes informed decisions. These expressions are employed in various programming scenarios, such as user input validation, error handling, and complex algorithm design.
Assignment Expressions
Assignment expressions are fundamental components of programming languages that allow developers to store and manipulate data within their code. These expressions involve the assignment operator (=), which assigns a value to a variable.
Assignment expressions serve as the primary means of changing the content of variables, making them indispensable for storing, updating, and managing data throughout a program’s execution.
In addition to simple assignments, many programming languages offer compound assignment operators, such as +=, -=, *=, and /=. These operators combine an operation with assignment in a concise way. For instance, “x += 5” is equivalent to “x = x + 5,” where the value of ‘x’ is incremented by 5.
Assignment expressions are used extensively in various programming tasks, including data manipulation, algorithmic computations, and state management within applications. A solid understanding of assignment expressions is crucial for any programmer, as they are at the heart of data handling in software development.
Moreover, assignment expressions are central to many programming paradigms, including imperative and object-oriented programming. They facilitate the modification of variables and object properties, making it possible to create dynamic and responsive software.
These expressions are not only about changing values but also about maintaining the state of an application as it progresses, ultimately contributing to the behavior and functionality of a program.
Functional Expressions
Functional expressions are a pivotal concept in programming, representing the capability to treat functions as first-class citizens. In essence, this means that functions can be assigned to variables, passed as arguments to other functions, and returned as values from functions.
This powerful feature opens the door to functional programming paradigms, where functions play a central role in the structure and behavior of code. Functional expressions facilitate the creation of higher-order functions, which are functions that take other functions as inputs or produce functions as outputs.
This flexibility enables developers to write more concise and modular code, enhancing code readability and maintainability. Functional expressions are widely used in various programming languages, from JavaScript and Python to languages like Haskell and Lisp, where functional programming is a core paradigm.
Understanding functional expressions is crucial for mastering functional programming and leveraging its benefits, such as improved code quality and easier debugging.
Conditional Expressions
Conditional expressions, often referred to as ternary operators, provide a concise and elegant way to express conditional logic within a single expression. They are particularly useful when you need to make decisions and assign values based on the truth or falsity of a condition.
In most programming languages, the conditional expression takes the form of
condition ? true_value : false_value
where the condition is evaluated first. If the condition is true, the expression returns true_value; otherwise, it returns false_value. This streamlined syntax enhances code readability by encapsulating conditional operations within a single line.
Conditional expressions find applications in various programming scenarios, especially when you want to simplify decision-making and avoid the verbosity of traditional if-else statements. They are often used for tasks like validating user input, selecting appropriate values based on specific conditions, and assigning default values when necessary.
While conditional expressions provide conciseness and clarity, it’s crucial to use them judiciously, as excessively complex conditional expressions can reduce code readability and maintainability.
Moreover, conditional expressions are valuable for improving code efficiency and can lead to more optimized programs. In situations where either the true or false value involves computationally intensive operations, using conditional expressions can help avoid unnecessary calculations by only evaluating the relevant branch.
This efficiency gain, coupled with their compact syntax, makes conditional expressions a valuable tool in a programmer’s toolkit.
Member Access Expressions
Member access expressions are a fundamental component of object-oriented programming, allowing developers to access the properties and methods of objects and data structures. These expressions are employed to retrieve or manipulate the attributes and behaviors associated with an object.
In most programming languages, the member access operator (often represented as a dot, such as object.property or object.method()) serves as the means to connect with the internal elements of an object. They are pivotal for encapsulation and abstraction, two core principles of object-oriented design. They enable developers to create objects with well-defined interfaces, shielding the internal details of an object from external code.
This encapsulation enhances code modularity, as different parts of a program can interact with an object without needing to know its internal implementation. Additionally, member access expressions are essential for code readability and maintenance, as they provide a clear and intuitive way to work with objects, making it evident which properties and methods are available for use.
Furthermore, these expressions are crucial for building relationships between objects in object-oriented programming. They enable objects to communicate and collaborate by invoking methods and accessing properties of other objects, facilitating the creation of complex systems and interactions within software applications.
Lambda Expressions
Lambda expressions, often referred to simply as “lambdas,” are a powerful feature in many modern programming languages, particularly those that support functional programming paradigms.
A lambda expression is essentially an anonymous, inline function that can be created and used on the fly without the need for a formal function declaration. Lambdas are characterized by their concise syntax, typically involving parameters, an arrow (->), and an expression to be evaluated. They are particularly useful when you need to define small, one-off functions for tasks such as filtering, mapping, or reducing data.
Lambda expressions are highly valued for their ability to enhance code readability and maintainability. They allow developers to express complex operations in a compact and intuitive manner.
For example, in languages like Python or JavaScript, lambdas can be employed with functions like map, filter, and reduce to streamline operations on collections of data. By encapsulating functionality within a lambda expression, developers can focus on the specific task at hand, without the need to create separate named functions.
Moreover, lambdas promote the concept of “closures,” enabling functions to capture and remember the state of their surrounding context. This capability is particularly useful in asynchronous and event-driven programming, where lambdas can capture variables and maintain their values across different execution contexts.
This feature empowers developers to write more efficient and expressive code in scenarios like event handling and callback functions. Understanding how to effectively use lambda expressions is essential for leveraging the benefits of functional programming and creating clean, concise, and maintainable code.
Composition in Programming
Composition refers to combining simple parts to form more complex structures or functionalities. Instead of building everything from scratch, developers leverage smaller, tested components, piecing them together to achieve a broader function. This approach promotes modularity, reusability, and clarity in software design.
A developer might have a function that calculates tax and another that calculates shipping. Instead of having a huge, single function for an entire checkout process, these smaller functions can be composed together. In a checkout function, the tax and shipping functions can be called, ensuring each part works as intended and making the code more readable.
Object-Oriented Programming (OOP) offers a direct application of composition with classes and objects. Instead of inheriting properties and behaviors, a class might include other objects to provide the functionality it needs. For instance, a Car class might not inherit from an Engine class. Instead, it would have an Engine object as one of its attributes, exemplifying composition.
Composition’s power lies in its simplicity. By building systems using well-defined, self-contained pieces, developers can better understand, test, and maintain their code. If one component fails or needs a change, it can be adjusted without widespread disruption. Embracing composition leads to more resilient and adaptable software.
Evaluation
Evaluation in programming refers to the process of computing or determining the value of an expression. When a program runs, expressions aren’t just left as static combinations of variables, values, and operators; they’re actively processed to produce outcomes.
Consider the simple expression 3 + 4 * 2. Before the program can use the result of this expression, it must evaluate it. In this case, following the rules of arithmetic, multiplication occurs before addition, so the expression evaluates to 11.
But evaluation isn’t limited to arithmetic. In a conditional statement, such as if (x > y), the expression x > y needs to be evaluated to either true or false before the program can decide which branch of code to execute next.
Evaluation can get more complex in functions. When a function is called with certain arguments, the expressions inside that function are evaluated using those specific argument values. If you have a function f(z) = z + 2 and call it with f(3), the expression z + 2 is evaluated with z being 3, producing a result of 5.
Nested Expressions in Programming
Nested expressions refer to expressions that contain other expressions within them. These inner expressions are evaluated first, and their results are used in the outer expression. Nesting can provide more complex logic or calculations, but it’s essential to understand the order in which these expressions are processed to ensure accurate results.
Imagine the arithmetic expression (3 + (4 * 2)) – 5. Here, the inner expression 4 * 2 is nested within the outer expression. The inner one gets evaluated first, resulting in 8, then the outer expression becomes 3 + 8 – 5, which evaluates to 6.
Languages that support conditional statements often see nested expressions in conditions. For example, in a statement like if ((x > y) && (a < b)), there are two comparisons nested within a larger logical expression.
Function calls can also be nested. If you have a function f(z) and another g(y), you might see expressions like f(g(3)). Here, g(3) is evaluated first, and its result is passed as an argument to f.
Nested expressions, while powerful, require careful handling. Ensuring that each level of nesting is correctly evaluated, and that the overall expression’s logic is sound is crucial. Proper use of parentheses or other grouping mechanisms can help clarify the order of operations and make the expression’s intent clearer to other developers.
Order of Evaluation
Order of evaluation in programming dictates the sequence in which parts of an expression are computed. Getting this order right is vital to ensure that expressions yield the expected results. Often, programming languages define specific rules for this order, but some operations might be left undefined, leading to potential inconsistencies across different systems or compilers.
A common rule in many languages is the arithmetic order of operations, often remembered by the acronym PEMDAS: Parentheses, Exponents, Multiplication and Division (from left to right), and Addition and Subtraction (from left to right). So, in the expression 3 + 4 * 2, the multiplication is performed before the addition, resulting in 11.
Beyond basic arithmetic, order matters in more complex expressions. Consider a logical expression like (x < y) || (a == b && c > d). Here, the comparison x < y is evaluated first. If it’s true, the rest might not even be evaluated in languages that use short-circuiting for logical operations.
Another aspect is the evaluation order in function arguments. In some languages, the order in which function arguments are evaluated isn’t strictly defined. For example, in the function call f(g(), h()), there’s no guarantee whether g() or h() is evaluated first in all languages.
Understanding the order of evaluation is crucial for writing correct and predictable code. Developers should be familiar with the rules of their specific programming language and use parentheses to clarify intentions when needed. This ensures that expressions are evaluated as intended, regardless of the platform or compiler.
Side Effects
Side effects in expressions refer to changes or actions that occur because of evaluating an expression, beyond just returning a value. These side effects can include modifying global or local variables, altering data structures, or causing observable interactions like writing to a file or displaying output.
While expressions with side effects can be powerful tools, they can also introduce challenges in understanding and maintaining code. Let’s consider a simple example in many programming languages: the increment operator, often written as ++. In the expression x = y++, the value of y is assigned to x, but as a side effect, y is also incremented by one.
Another common side effect is when a function, called within an expression, changes the state of the program. If you have a function f() that, apart from returning a value, also modifies a global variable or writes to a database, then calling this function has side effects.
Side effects can complicate understanding code, especially when trying to predict how a program will behave. For instance, in an expression that combines multiple function calls, result = f(g(), h()), if both g() and h() have side effects, the outcome might differ based on the order they’re evaluated.
For cleaner and more predictable code, many developers and programming paradigms advocate for minimizing side effects, especially in expressions. Functional programming emphasizes creating functions without side effects, ensuring they always produce the same output for the same input without altering any state.
In conclusion, while side effects can provide efficient shortcuts in code, they should be used judiciously. Being aware of potential side effects helps developers avoid unforeseen complications and ensures more transparent and maintainable software.
Pure vs. Impure Expressions in Programming
Pure and impure expressions represent two different behaviors, particularly relevant in the context of functional programming and overall code design. Understanding their differences is key to ensuring code predictability and maintainability.
A pure expression is one that always produces the same output given the same input and has no side effects. In other words, its evaluation doesn’t alter any state or have observable interactions outside of returning a value.
Pure expressions offer predictability, which can simplify debugging and reasoning about code. For instance, a function f(x) that returns x * x is pure because it always gives the same output for the same x and doesn’t change anything externally.
On the other hand, an impure expression is one that might produce different outputs for the same input or cause side effects. This could be due to interactions with external data sources, global variables, or other elements of mutable state. For example, a function g() that returns the current system time is impure because its output changes every time it’s called, even with the same inputs.
Impure expressions can introduce complexity because their outcomes can be affected by external factors. However, they’re often essential for many practical purposes, like database interactions, file operations, or getting user input.
While pure expressions offer easier testing, optimization, and parallelization, real-world programs often need impure expressions to interact with the environment. The key is to strike a balance. Developers should aim to isolate and minimize impure parts, keeping the core logic as pure as possible. This approach leads to software that’s both functional and maintainable.
Take a tour of C#, the language used in Unity, which is used in our guides.