In the third installment of our exploration into programming language elements, we turn our attention to a crucial and versatile component of code: operators. Operators are the tools that empower developers to perform a wide array of operations on data, ranging from basic arithmetic calculations to complex logical comparisons. Operators can be categorized into several groups based on their primary functions.
Arithmetic operators, for instance, are used to perform mathematical calculations like addition, subtraction, multiplication, and division. These operators are essential for handling numeric data in various programming tasks.
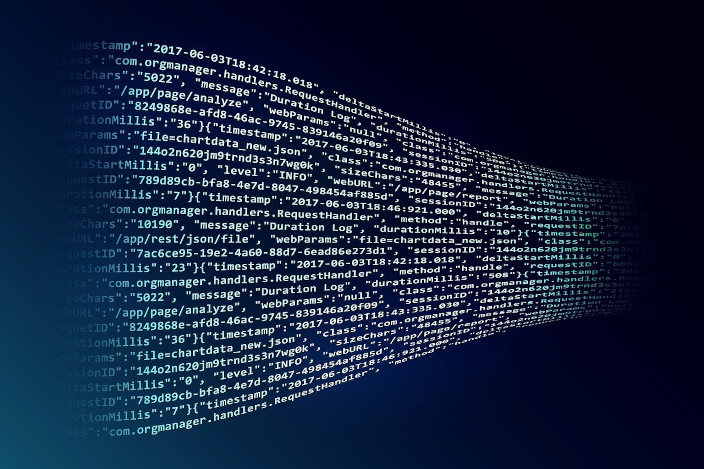
Logical operators, on the other hand, enable us to make decisions and control program flow by evaluating conditions. They include logical AND, OR, and NOT operators, allowing us to create complex decision-making processes within our code.
Comparison operators are pivotal for assessing the equality or inequality of values. These operators help us create conditional statements that guide the program’s behavior based on the results of comparisons.
Assignment operators play a central role in assigning values to variables, making them a fundamental part of variable manipulation and data storage.
Bitwise operators, while less commonly used, are essential for working with binary data at the bit level, which is particularly relevant in low-level programming tasks and certain algorithmic implementations.
By diving into the realm of operators in this part of our discussion, we aim to provide a comprehensive understanding of how these tools enable programmers to manipulate and control data effectively.
Moreover, we will explore operator precedence and associativity, which are critical concepts for ensuring that expressions are evaluated correctly. Through this exploration, you’ll gain the knowledge and skills necessary to wield operators with precision and efficiency, making you a more capable and versatile programmer.
Arithmetic Operators in Programming
Arithmetic operators are fundamental tools used for performing mathematical calculations. They resemble the basic operations we learn in elementary math but are used to instruct computers. Common arithmetic operators include “+” for addition, “-” for subtraction, “*” for multiplication, and “/” for division. Some languages also offer operators like “%” for modulus, which gives the remainder of a division.
These operators act on numerical values, either constants or variables, to produce a result. When used in programming, they enable developers to perform calculations, analyze data, and solve mathematical problems. Their behavior can sometimes vary based on the data types of the operands. For instance, division between two integers might round down in some languages.
Comparison Operators in Programming
Comparison operators are essential for making decisions and comparing values. They evaluate the relationship between two operands and return a boolean result: true or false. Common comparison operators include “==” for equality, “!=” for inequality, “>” for greater than, “<” for less than, “>=” for greater than or equal to, and “<=” for less than or equal to.
When applied, they allow developers to check conditions, such as whether a user’s input matches a stored password or if a score surpasses a certain threshold. These operators are fundamental in control structures like “if” statements, enabling the program to branch and execute different code based on certain conditions.
Logical Operators in Programming
Logical operators are used to form compound conditions by combining or modifying boolean values. They play a pivotal role in decision-making structures, determining the flow of a program based on multiple criteria.
Common logical operators include “AND” (often represented as “&&”), “OR” (often “||”), and “NOT” (often “!”). For instance, the “AND” operator returns true only if both of its operands are true. The “OR” operator returns true if at least one of its operands is true. Meanwhile, the “NOT” operator simply inverts the truth value of its operand.
By using these operators, developers can craft complex conditions. Imagine a scenario where a user can access content only if they are both a subscriber “AND” their account is active. Logical operators make such evaluations straightforward.
Assignment Operators in Programming
Assignment operators are pivotal for setting or updating the value of variables. The most basic assignment operator is the “=” sign, which assigns a value to a variable. For example, x = 5 means the variable “x” now holds the value of 5.
But there’s more to assignment than just the basic “=” operator. Many languages introduce compound assignment operators that combine arithmetic operations with assignment. Examples include “+=” (add and assign), “-=” (subtract and assign), “*=” (multiply and assign), and “/=” (divide and assign). So, if x is 10, the statement x += 5 would result in x being 15; it’s a shorthand for x = x + 5.
Bitwise Operators
Bitwise operators in programming delve into the realm of binary operations, directly manipulating individual bits of data. These operators are especially important in low-level programming, optimization, or when interacting with hardware.
& (AND): Compares each bit of the first operand to the corresponding bit of the second operand. If both bits are 1, the corresponding result bit is set to 1; otherwise, it’s set to 0.
| (OR): If either (or both) of the bits in the compared position are 1, the bit in the resulting binary representation is 1; otherwise, it’s 0.
^ (XOR): Returns 1 for each position where either of the corresponding bits of the operands is 1, but not both.
~ (NOT): Inverts the bits, turning 0s into 1s and 1s into 0s.
<< (Left Shift): Shifts the bits of the left operand to the left by the number of positions specified by the right operand.
>> (Right Shift): Shifts the bits of the left operand to the right by the number of positions specified by the right operand.
Bitwise operators are powerful tools, but they require a solid understanding of binary operations and often come into play in specific scenarios. They can optimize calculations, handle flags and masks, or work directly with hardware registers. Developers using them should tread carefully, ensuring they understand the implications on data when manipulating at the bit level.
Conditional (Ternary) Operator
The Conditional (Ternary) Operator is a concise way to execute simple if-else statements. Instead of writing a full-blown conditional block, this operator allows developers to set a value based on a condition in a single line. It’s called “ternary” because it operates on three operands: the condition, the value if the condition is true, and the value if the condition is false.
In many languages, the ternary operator uses the ? and : symbols. The format often looks like this:
condition ? value_if_true : value_if_false
For instance, consider deciding between two prices based on a membership. In a language like JavaScript, you might have:
price = isMember ? memberPrice : regularPrice;
Here, if “isMember” is true, “price” gets the “memberPrice”. If false, it gets the “regularPrice”.
This operator is prized for its brevity and clarity in straightforward scenarios. However, developers should use it judiciously. Overuse or nesting multiple ternary operations can lead to code that’s hard to read. For complex conditions, traditional if-else structures might be more suitable and readable.
Type Operators
Type operators are used to gather information or manipulate the datatype of variables or values. Their role is crucial in languages that are strongly typed or in scenarios where type safety is essential.
A common type operator is the “typeof” operator, often found in languages like JavaScript. It returns a string indicating the type of a given operand. For example, using typeof on a number might return the string “number”.
Another example is the “instanceof” operator, which checks if an object is an instance of a particular class or constructor in object-oriented languages. In Java, you might see: if (animal instanceof Dog), which would check if the “animal” object is an instance of the “Dog” class.
Some languages also provide type casting operators, allowing developers to convert one datatype into another. For instance, in C++, one can use (int) before a float variable to convert or cast it to an integer.
Understanding and properly using type operators is crucial. They ensure that data is processed and manipulated correctly, preventing potential runtime errors or unexpected behaviors. In type-sensitive scenarios, these operators help developers manage and ensure data consistency throughout their code.
Membership Operators (Inclusion)
Membership or inclusion operators are used in some programming languages to determine whether a value is a member of a collection, such as a list, array, or dictionary. Their primary role is to test for the presence of a value, making them especially useful in data manipulation and conditional testing.
In Python, for example, the “in” operator is widely used to check membership. If you have a list like fruits = [“apple”, “banana”, “cherry”], the expression “apple” in fruits would return True because “apple” is in the list. Conversely, “grape” in fruits would return False.
These operators can also work on strings to check for substrings. In the earlier example, the expression “app” in “apple” would return True as “app” is a substring of “apple”.
For dictionaries or objects, the “in” operator usually checks for the presence of a key rather than a value. For example, if you have a dictionary person = {“name”: “John”, “age”: 30}, the expression “name” in person would return True, while “John” in person would return False.
Using membership operators can simplify code by reducing the need for loop-based checks. They allow for straightforward, readable conditions that can make certain tasks, like filtering or searching, more efficient and clearer to understand.
Identity Operators
Identity operators are utilized in certain programming languages to compare the memory locations of two objects rather than their content or value. Essentially, they determine if two variables reference the exact same object in memory. These operators are especially relevant in languages where objects can be stored in memory and referenced by multiple variables.
Python, for example, offers two primary identity operators: “is” and “is not”. These operators compare the memory locations of two objects.
Consider the following:
a = [1, 2, 3]
b = a
c = [1, 2, 3]
Here, b is a would return True because both variables point to the same list in memory. However, c is a would return False, even though their contents are identical, because they are two distinct objects in memory.
Identity operators are essential for scenarios where it’s important to know if two variables are pointing to the same object, rather than just having matching values. Properly used, they can prevent unintended data modifications and help in understanding how data structures are manipulated within a program.
Unary Operators
Unary operators are a subset of operators that operate on a single operand or variable. Unlike binary operators, which require two operands, unary operators perform their action on just one input. They’re used to modify the state or value of a single variable in a particular way.
(–) The negative operator. It inverts the sign of a numerical value. For example, if x = 5, then -x would be -5.
(+) The positive operator. While often implied and not strictly necessary, it can be used to denote a positive number explicitly.
(!) Logical NOT operator, common in many languages like JavaScript and C. It inverts the truthiness of a boolean value. So, if b = true, then !b would be false.
(~) Bitwise NOT operator. It inverts the bits of a number. For instance, in binary, if a bit is 1, it becomes 0, and if it’s 0, it becomes 1.
(++) Increment operator. Increases the value of a variable by one. Found in languages like C, C++, and Java.
(—) Decrement operator. Decreases the value of a variable by one.
Using unary operators helps to simplify and clarify code, especially in scenarios that require quick modifications to a single variable’s state or value. They provide a concise way to express an operation, making code more readable and often more efficient.
Demystifying Operator Precedence in Programming
Operator precedence is a fundamental concept in programming that determines the order in which operators are evaluated in an expression. This concept plays a critical role in ensuring that complex expressions are computed correctly and can significantly impact the outcome of your code.
In programming languages, various operators exist, each with its own precedence level. Operators with higher precedence are evaluated before operators with lower precedence. For example, in many programming languages, multiplication (*) has higher precedence than addition (+). So, in the expression 2 + 3 * 4, the multiplication is evaluated first, resulting in 2 + 12, which equals 14.
Here’s a typical list of operator precedence in many programming languages, with higher precedence operators appearing at the top:
- Parentheses: ( )
- Exponentiation: **
- Multiplication and Division: *, /, % (modulus)
- Addition and Subtraction: +, –
- Bitwise Shift: << (left shift), >> (right shift)
- Bitwise AND: &
- Bitwise XOR: ^
- Bitwise OR: |
- Comparison Operators: <, <=, >, >=, ==, !=
- Logical NOT: !
- Logical AND: &&
- Logical OR: ||
- Ternary Conditional Operator: ? :
- Assignment Operators: =, +=, -= and so on
It’s important to note that this list represents a general guideline for operator precedence, and actual precedence might vary slightly depending on the programming language.
Understanding operator precedence is crucial for writing code that behaves as expected. It helps avoid the need for excessive parentheses and clarifies the order in which operations occur.
In cases where you want to override operator precedence, you can use parentheses to explicitly specify the order of evaluation. This practice not only enhances code clarity but also ensures that your intentions are correctly interpreted by the compiler or interpreter.
Understanding Operator Associativity in Programming
Operator associativity is a fundamental concept in programming that determines the order in which operators of equal precedence are evaluated within an expression. This concept is crucial for ensuring that expressions are evaluated correctly and consistently, particularly when dealing with multiple operators of the same precedence level.
In most programming languages, operators can be categorized as either left-associative or right-associative:
Left-Associative Operators
When operators are left-associative, they are evaluated from left to right within an expression. This means that if you have multiple operators of the same precedence, they are processed in the order in which they appear from left to right. For example, in the expression a + b + c, where + is a left-associative operator, the addition is performed from left to right: first a + b, and then (a + b) + c.
Right-Associative Operators
Conversely, right-associative operators are evaluated from right to left within an expression. This means that the rightmost operation is performed first, followed by the one to its left, and so on. An example of a right-associative operator is the assignment operator in some languages. In the expression x = y = 5, the assignment is carried out from right to left: first y is assigned the value 5, and then x is assigned the value of y.
Understanding operator associativity is vital for writing correct and predictable code. When you have expressions with multiple operators of equal precedence, associativity helps you determine the order in which operations are executed. It also plays a crucial role in mathematical and logical calculations, ensuring that your code produces the expected results.
To further clarify the order of operations, parentheses can be used to override operator associativity. By placing operations inside parentheses, you can explicitly specify the order of evaluation, ensuring that your intentions are accurately reflected in the code.
In summary, grasping operator associativity is an essential aspect of programming that contributes to the correctness and predictability of your code. It enables you to understand how expressions are evaluated and empowers you to write code that behaves as intended, even in situations involving multiple operators with the same precedence.
Take a tour of C#, the language used in Unity, which is used in our guides.