In this second part of our exploration into programming language elements, we will delve into the significance of identifiers and delimiters within the context of computer programming.
Identifiers, as the names assigned to variables, functions, classes, and various program elements, play a pivotal role in making code both understandable and maintainable. We’ll explore the rules and best practices associated with choosing meaningful and descriptive names, emphasizing the importance of clarity and consistency in the naming conventions.
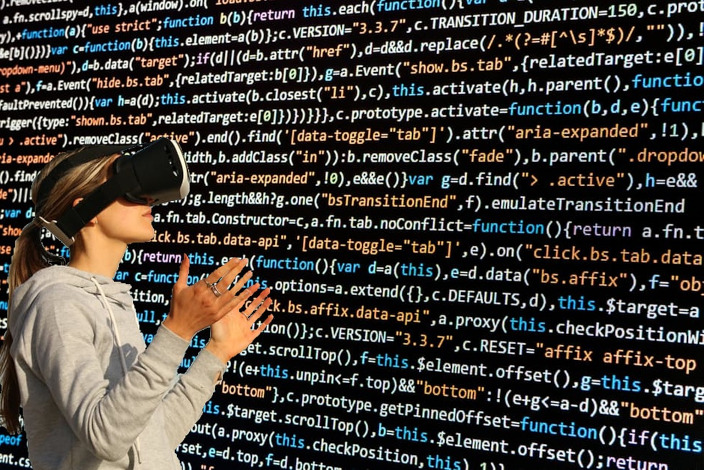
On the other hand, delimiters are essential characters or symbols that act as separators or boundaries within code or data structures. These characters come in diverse forms, such as parentheses, brackets, quotation marks, and punctuation symbols.
They serve to encapsulate code blocks, delineate function arguments, or specify the beginning and end of strings. We’ll discuss the various types of delimiters and how they contribute to code organization, data integrity, and overall program structure.
Together, our discussion on identifiers and delimiters will provide valuable insights into the foundational elements of programming languages, offering guidance on how to write code that is not only functional but also comprehensible and well-structured.
Understanding Identifiers in Programming Languages
Each programming language has specific rules for creating identifiers, such as starting with a letter or underscore and not using reserved keywords. It’s vital to choose meaningful names to make the code more readable and maintainable.
Well-chosen identifiers for variables, functions, and other program elements can frequently be the deciding factor in whether a program is difficult to comprehend or self-explanatory. This distinction is highly advantageous not only to the original developer but also to any potential future collaborators or maintainers of the codebase.
When identifiers are clear and meaningful, they act as signposts within the code, guiding readers and making the purpose and usage of various elements instantly apparent. This enhanced clarity and transparency can significantly reduce the time and effort required for troubleshooting, debugging, and extending the program.
Consequently, investing the time and thought into selecting appropriate identifiers is a practice that yields long-term benefits in terms of code quality, maintainability, and collaborative development.
Guidelines and Best Practices for Naming in Programming
Rules for naming identifiers ensure consistency and clarity in programming. These rules differ among programming languages, but many share common guidelines. First and foremost, identifiers must begin with a letter (either uppercase or lowercase) or an underscore.
Following characters can be letters, numbers, or underscores. Importantly, spaces are typically not allowed within identifiers. Reserved keywords, specific to each programming language, cannot be used as identifiers. For instance, words like “if”, “else”, or “return” in many languages have predefined meanings and cannot be repurposed as variable names.
Beyond these basic rules, good practices encourage meaningful and descriptive names. For example, a variable storing a user’s age might be named “user_age” rather than a vague “ua” or “x”. Additionally, many developers follow naming conventions like camelCase or snake_case to maintain readability, especially in multi-word identifiers.
Descriptive and Meaningful Names
Opt for names that convey the purpose or content of the element. Avoid cryptic abbreviations or overly short names. Clarity should always be a top priority.
Consistency
Maintain a consistent naming style throughout your codebase. Whether you choose camelCase, snake_case, or another convention, stick with it to create a uniform and harmonious codebase.
Use Pronounceable Names
If you can’t easily pronounce a variable or function name, it’s a sign that it might be too complex. Easily spoken names are typically easier to understand and remember.
Avoid Ambiguity
Ensure that your names are unambiguous. If there’s room for confusion, consider appending clarifying words or using more specific terms.
Mind Your Case
Depending on the programming language, naming conventions may dictate whether you use camelCase, PascalCase, or snake_case. Familiarize yourself with your language’s conventions and adhere to them.
CamelCase
CamelCase, also known as camelCase or camelBack, is a naming convention where compound words are concatenated together, and each word (except the first) starts with a capital letter, without any spaces or punctuation.
For example, “myVariableName” or “calculateTotalAmount.” This convention is often used in programming languages like JavaScript and is particularly popular for naming variables and functions. CamelCase is preferred when you want to create concise yet descriptive names that are easy to read and understand.
PascalCase
PascalCase, sometimes called UpperCamelCase, is similar to CamelCase, but it starts every word with a capital letter, including the first word. For instance, “MyClassName” or “CalculateTotalAmount.”
This convention is often used for naming classes and types in languages like C# and Java. PascalCase is chosen for class names to make them stand out and clearly indicate that they are user-defined types or objects.
snake_case
Unlike CamelCase and PascalCase, snake_case uses underscores (_) to separate words in a name, and all letters are typically in lowercase. For example, “my_variable_name” or “calculate_total_amount.”
This convention is commonly employed in languages like Python and Ruby, and it is often preferred for naming variables, functions, and file names. Snake_case is chosen for its readability and consistency, especially in situations where spaces or mixed capitalization aren’t allowed.
Avoid Reserved Words
Don’t use language-specific reserved words as identifiers, as this can lead to unexpected behavior or errors.
Length Matters
While descriptive names are crucial, excessively long names can be cumbersome. Strike a balance between clarity and brevity.
Consider Context
Be mindful of the scope and context in which an identifier is used. Local variables, for example, can have shorter names than global constants.
Use Comments Sparingly
While good naming reduces the need for comments, they can still be valuable for explaining complex logic or providing additional context.
Regular Review
As your code evolves, revisit your identifier names. As the codebase grows, names that made sense initially might need adjustments to maintain clarity.
Version Control
If you’re working in a team, coordinate with your colleagues on naming conventions to ensure consistency across the project.
Meaningful Prefixes/Suffixes
Consider using prefixes or suffixes for special cases. For example, ‘is_’ for boolean variables or ‘_callback’ for callback functions.
Scopes and Visibility Concepts in Programming
Scopes and visibility are fundamental concepts in programming that determine the accessibility and lifetime of variables and functions. In essence, they dictate where in a program a particular identifier can be accessed or modified.
Scope
Scope is a fundamental concept in programming that determines the range and validity of an identifier within a codebase. It essentially defines where a variable, function, or other named entity can be accessed and utilized.
Local Scope
Local scope is a type of scope that confines the usability of an identifier to a specific block of code, often found within functions or methods. When a variable is declared within a local scope, it is only accessible within that particular block or function.
As soon as the function completes its execution, the variables declared within its local scope typically go out of existence, freeing up memory resources and preventing unintended interactions with other parts of the code. This encapsulation of data is essential for maintaining the integrity and predictability of a program.
Global Scope
On the other hand, global scope extends the reach of an identifier to the entirety of the codebase. Variables and functions declared in the global scope are accessible from any part of the program, making them available for the entire duration of the program’s execution.
While global scope provides convenience by allowing data to be shared across different parts of the code, it also comes with potential challenges, such as the risk of unintended variable overwrites or complex debugging scenarios.
Name Conflicts
In a large codebase, it’s easy for different parts of the program to unintentionally use the same variable name. If multiple parts of the code modify the same global variable, it can lead to unexpected behavior and difficult-to-debug issues.
Lack of Encapsulation
Global variables and functions are not encapsulated within a specific context or module, which can make it challenging to determine where they are defined or used. This lack of encapsulation can reduce code clarity and maintainability.
Security Risks
In some cases, global variables may contain sensitive information. If not properly protected or restricted, this information can be accessed or modified by unauthorized parts of the code, leading to security vulnerabilities.
Testing and Debugging Complexity
Global variables can complicate the testing and debugging process. Changes to global state can affect the behavior of functions in unpredictable ways, making it harder to isolate and fix issues.
Maintainability Challenges
As a codebase grows, managing and tracking global variables and functions becomes increasingly difficult. It can be challenging to determine which parts of the code rely on global state and how changes to that state impact the program as a whole.
Visibility
Visibility is a key concept in programming that closely relates to scope and determines which parts of the codebase can interact with or manipulate a given identifier, such as a variable, function, or class. It governs the accessibility of these identifiers and plays a crucial role in encapsulation and code organization.
Private Visibility
Private visibility is a common visibility level employed in many programming languages. It restricts access to the identifier to a specific class or module, effectively encapsulating it within a limited scope. This encapsulation ensures that the identifier is only accessible and modifiable within the confines of its defining class or module.
This practice helps prevent unintended interference or modification of data, enhancing code stability and security. It also promotes the concept of information hiding, where the internal details of a class or module are hidden from external code.
Public Visibility
Conversely, public visibility allows for broader access to an identifier. When an identifier is marked as public, it can be accessed and used from any part of the codebase, including other classes, modules, or even external libraries. This accessibility is often necessary for providing a well-defined and user-friendly interface to the functionality or data offered by a class or module.
In addition to private and public visibility, some programming languages introduce further levels of visibility, such as protected or internal.
Protected and Internal Visibility
Protected visibility typically allows access to an identifier within subclasses, supporting the concept of inheritance and enabling derived classes to utilize certain attributes or methods from their parent class.
Internal visibility, on the other hand, grants access to identifiers within a specific module or package, making them available to a limited subset of the codebase. These additional visibility levels provide more nuanced control over the accessibility of identifiers, enabling developers to strike a balance between encapsulation and code reusability.
In conclusion, visibility is a critical aspect of software design and encapsulation, specifying who can interact with an identifier within a codebase. By judiciously choosing the appropriate visibility level for each identifier, programmers can maintain code integrity, promote information hiding, and create well-structured and maintainable software systems.
Different programming languages offer varying levels of visibility to cater to the needs of developers and the complexities of modern software development. Both scopes and visibility are vital for encapsulating data, a core principle in many programming paradigms.
Properly managed, they prevent unintended data alterations and keep the codebase organized, readable, and maintainable. They enable developers to control data flow, reduce errors, and promote modularity in software design.
Exploring the Significance of Delimiters in Programming
Delimiters, within the context of programming, serve as essential tools for shaping the structure and syntax of code. These characters, or sequences of characters, are strategically placed within the code to fulfill several vital roles, contributing significantly to the readability, organization, and execution of a program.
Boundary Marking
First and foremost, delimiters act as boundary markers, establishing where certain elements within the code begin and end. They set clear demarcations between different components, such as statements, expressions, or code blocks.
For instance, curly braces {} in languages like C++ or JavaScript are commonly used delimiters to define the scope of functions, loops, or conditional statements. Without these delimiters, the code’s structure would be ambiguous, leading to syntax errors and unpredictable behavior.
Separating and Structuring Data
Delimiters are also instrumental in separating and structuring data. In languages that use commas, semicolons, or spaces as delimiters, they help organize arrays, lists, and tables of information, ensuring that the data can be easily parsed and manipulated.
For instance, in a comma-separated values (CSV) file, the comma delimiter distinguishes between individual data fields, allowing data to be organized into rows and columns.
Defining the Syntax
Moreover, delimiters play a crucial role in defining the syntax of a programming language. They communicate the intended meaning of code to both developers and the compiler or interpreter.
For example, parentheses () are used as delimiters to group expressions, ensuring that operations are performed in the desired order. In mathematical expressions, delimiters like parentheses and brackets help clarify complex calculations by indicating precedence and associativity.
Lexical Analysis
Furthermore, delimiters are integral to the lexical analysis phase of compilation, where they assist in tokenization—breaking down the source code into smaller, meaningful units called tokens.
These tokens, which include identifiers, keywords, operators, and literals, are identified based on the presence of specific delimiters, whitespace characters, and other lexical cues.
Types of Delimiters
The delimiters come in various types, each with its distinct purpose and significance. One common category encompasses symbols like parentheses, brackets, and braces, which are used to group and hierarchically organize code blocks.
Another important type includes quotation marks, such as single (‘ ‘) or double (” “), which denote string literals. Additionally, special characters like commas, semicolons, and colons act as delimiters to separate elements within data structures or function arguments.
The choice and proper use of delimiters are crucial for maintaining code clarity and ensuring data integrity, making them fundamental aspects of programming languages and data representation.
Statement Delimiters
Many programming languages use delimiters to separate individual statements or expressions. A common statement delimiter is the semicolon (;), which is used to indicate the end of a statement.
Example: x = 5;
Block Delimiters
Block delimiters are used to enclose groups of statements, creating compound or block structures. These are often used with control flow statements like loops and conditionals. The most common block delimiters are curly braces {}.
Example: if (x > 0) { /*code to execute if x is greater than 0*/ }
String Delimiters
String delimiters are used to enclose string literals within the code. These delimiters define the beginning and end of a string. Common string delimiters include single quotes (‘) and double quotes (“).
Example: message = “Hello, world!”;
Character Delimiters
Character delimiters are used to represent individual characters as literals. They are typically enclosed in single quotes.
Example: char letter = ‘A’;
Pair Delimiters
Some programming languages use paired delimiters to represent hierarchical relationships or matched pairs, such as parentheses (), square brackets [], and angle brackets <>. These are often used to group expressions, pass function arguments, and define arrays or lists.
Example: int[] numbers = {1, 2, 3};
Comment Delimiters
Comment delimiters are used to enclose comments in the code, which are ignored by the compiler or interpreter. Comments are used to provide explanations, documentation, or notes to other programmers.
Example: // This is a comment. or /* This is a multiline comment. */
Escape Sequences
Some languages use special escape sequences to represent characters that are difficult to include directly in a string.
Example: in C-style languages, \n represents a newline character.
Whitespace Delimiters
While not always explicitly mentioned, spaces, tabs, and line breaks are also considered delimiters as they separate different parts of code, such as keywords, identifiers, and operators.
Take a tour of C#, the language used in Unity, which is used in our guides.