In the first part of our exploration into programming language elements, we will dive into the foundational building blocks that form the bedrock of computer programs. These core elements encompass keywords, variables, expressions, and statements, and they are the fundamental ingredients that developers use to craft software solutions.
Keywords are the reserved words within a programming language, imbued with specific meanings and functionalities. They serve as the vocabulary through which developers communicate with the computer, instructing it to perform various tasks and operations. Understanding and appropriately using keywords is essential for writing syntactically correct and logically sound code.
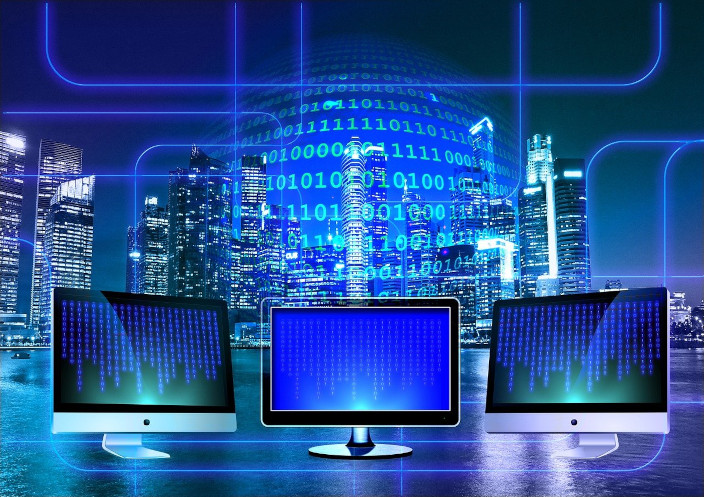
Variables, on the other hand, act as dynamic containers that hold data values. They allow programs to store, manipulate, and retrieve information as needed during execution. Variables are the workhorses of programming, enabling us to work with data in a flexible and dynamic manner.
Expressions are how we represent computations and operations in code. They are composed of operators and operands, where operators define the action to be performed, and operands are the values on which those actions are executed. Expressions enable us to perform calculations, make decisions, and control program flow.
Finally, statements are the executable units of code that carry out specific actions or operations. They are the sentences in the programming language, and they provide the sequence of instructions that dictate how a program behaves. Properly crafting statements is crucial for defining program logic and ensuring that it performs as intended.
Collectively, our exploration of keywords, variables, expressions, and statements in this first part will lay the groundwork for a deeper understanding of programming language elements. These elements are the building blocks upon which the art and science of software development are constructed and mastering them is essential for becoming proficient in the world of programming.
Keywords in Programming Languages
Keywords are special words in programming languages with set meanings, helping shape the language’s rules and format. These words can’t be used for naming variables or functions since they’re kept for particular tasks.
Programming constructs are the essential parts that define a programming language’s rules and meaning. They determine how logic, data handling, and other tasks are framed in code. While each language has unique constructs, many share common elements.
Keyword Characteristics
Keywords in programming languages serve as foundational building blocks. These reserved words have distinct characteristics that set them apart from regular identifiers or variables.
Reserved Nature
Keywords are reserved words, meaning they have a predetermined use and cannot be used for any other purpose, such as naming variables, classes, or functions. This ensures clarity in the language’s syntax.
Standardized Meaning
Each keyword has a standardized meaning across any program written in that language. For instance, “if” in many languages is always used for conditional checks, and its purpose doesn’t change from one program to another.
Language-Specific
Keywords can vary from one programming language to another. What is a keyword in one language might be a regular identifier in another.
Predictable Behavior
Due to their standardized meaning, keywords have predictable behaviors. Developers can rely on these behaviors to achieve specific tasks in the programming language.
Invariable
Keywords cannot be modified or redefined. Their functionality and definition remain constant in a given language, ensuring consistency.
Compact Representation
They often represent complex operations or structures in a concise manner. For example, the “for” keyword in many languages signifies a loop structure, saving the developer from writing multiple lines of code to create the loop manually.
Integral to Syntax
They form the essential syntax and structure of a programming language. Without them, it would be challenging to convey specific operations or commands to the computer.
Examples of Keywords
The set of keywords varies depending on the programming language, but common keywords found in many languages include:
Control Flow: if, else, switch, case, default, while, for, do, break, continue, return.
Data Types: int, float, bool, string, char, double.
Access Modifiers: public, private, protected, internal.
Class and Object-related: class, interface, new, this, base.
Exception Handling: try, catch, finally, throw.
Miscellaneous: static, const, readonly, virtual, abstract.
Variables and Data Types in Programming
Variables act like containers that store data values. Think of them as labeled boxes, where the label is the variable’s name, and the content inside is the data. Data types, on the other hand, define the kind of data a variable can hold. Common data types include integers (whole numbers), floats (decimal numbers), and strings (text).
The choice of data type ensures that the stored information is used correctly and efficiently. For instance, a variable set as an integer type can’t store text. By using variables and specifying their data types, developers can manage and manipulate data effectively in their programs.
Expressions in Programming
Expressions are combinations of variables, values, operators, and functions that the computer evaluates to produce a single result. Think of them as mathematical formulas. For example, in the expression “x + y,” both x and y are variables, and the “+” is an operator.
Expressions can be simple, like the previous example, or complex, combining multiple elements. Their main role is to perform calculations, manipulate data, or determine specific outcomes based on given conditions. By understanding and crafting expressions, developers can control the flow of a program and ensure desired results are achieved.
Statements in Programming
Statements are like complete sentences in a human language. They represent individual instructions that tell the computer what to do. In most programming languages, statements end with a semicolon or are defined by a new line.
Examples of statements include assigning a value to a variable, executing a function, or controlling the flow of a program with structures like “if” or “for” loops. While expressions evaluate to produce a value, statements carry out tasks. Grouped together, statements form the body of functions or programs, guiding the computer step by step to achieve the desired outcome.
Control Flow and Iteration in Programming
Control flow refers to the order in which the program executes statements. It determines the path or sequence followed by the code. Basic control flow structures include conditionals, like “if” statements, which allow the program to make decisions. If a specific condition is met, one block of code might be executed; if not, another block might run.
Loops, such as “for” or “while”, repeat certain actions multiple times. By using these structures, developers can create dynamic and responsive programs. Proper control flow ensures that the software behaves logically, efficiently, and meets its intended purpose, reacting to different inputs or scenarios.
Functions and Procedures in Programming
Functions and procedures are blocks of reusable code designed to perform specific tasks. They help organize and modularize code, making it more readable and maintainable. The key difference is that functions return a value, while procedures perform an action without necessarily returning anything.
For instance, a function might calculate and return the sum of two numbers, while a procedure might display a message on the screen. Both can take inputs, known as parameters or arguments, to influence their behavior. By utilizing functions and procedures, developers can avoid repetitive code, making programs more efficient and easier to debug. They represent the principle of “write once, use many times,” enhancing the overall structure and logic of software.
Classes and Objects (Object-Oriented Programming)
Object-Oriented Programming (OOP) is a programming paradigm centered around the concept of objects. Objects are instances of classes, which are essentially blueprints defining attributes and behaviors. The primary principles of OOP are encapsulation, inheritance, polymorphism, and abstraction.
Encapsulation bundles data (attributes) and methods (functions) into a single unit, the object. Inheritance allows one class to inherit properties and behaviors from another, promoting code reuse. Polymorphism lets objects of different classes be treated as instances of the same class through inheritance. Abstraction simplifies complex systems by highlighting the essential details and hiding intricate ones. Together, these principles allow for a more modular and organized approach to software design, making programs easier to maintain, scale, and understand.
Classes and objects are fundamental concepts in object-oriented programming (OOP). A class is like a blueprint that defines attributes (often called properties) and methods (functions related to the class). It encapsulates data for the object and methods to manipulate that data. For example, think of a class as a template for a “Car,” detailing attributes like color, make, and model, and methods like “start” or “brake.”
An object, on the other hand, is an instance of that class. Using our “Car” example, while the class defines what a car is in general, an object might represent a specific car, like a red Toyota Corolla. Multiple objects can be created from a single class, each having its own set of attribute values.
Together, classes and objects enable the principles of encapsulation, inheritance, and polymorphism in OOP, allowing developers to create modular, scalable, and organized code structures.
Inheritance and Polymorphism
Inheritance and polymorphism stand as pillars in Object-Oriented Programming (OOP). Inheritance lets a new class adopt features from an existing one. It streamlines code, reducing repetition by letting one class “inherit” properties and behaviors of another. This hierarchy aids both organization and efficiency.
Polymorphism, meanwhile, is about flexibility. It allows objects from various classes to be treated uniformly. In simple terms, it’s like different tools working with one handle. This adaptability ensures developers can use varied objects seamlessly, under a common umbrella.
Together, these concepts pave the way for dynamic, scalable software. They trim redundancy and elevate the code’s adaptability.
Exceptions and Error Handling in Programming
Exceptions and error handling are vita. Errors are inevitable in software development. Whether they arise from user input, unexpected scenarios, or coding mistakes, they can disrupt program flow. Exceptions are the program’s response to these errors. Instead of letting the program crash, exceptions offer a controlled way to capture the error and handle it.
Error handling mechanisms, like “try-catch” blocks in many languages, allow developers to define specific actions when an exception arises. This might include logging the error, informing the user, or even trying an alternative action. By incorporating error handling, developers ensure smoother user experiences, robust applications, and easier troubleshooting when issues arise.
Modules, Packages, and Namespaces in Programming
Modules, packages, and namespaces are organizational tools, crucial for managing large codebases and enhancing code reusability. A module typically refers to a file containing a collection of related functions, classes, or variables. Instead of cramming all code into one file, developers can split it across multiple modules for clarity and maintainability.
Packages take modularity a step further. In languages like Python or Java, a package is a collection of related modules grouped together. This hierarchy aids in structuring larger projects, making them easier to navigate and manage.
Namespaces, often seen in languages like C++ or C#, prevent naming conflicts. They provide a distinct space where identifiers like class or function names won’t clash with others from different namespaces. In essence, these tools help in structuring and organizing code, ensuring scalability, readability, and efficient collaboration among developers.
Generics (Parametric Polymorphism) in Programming
Generics, often referred to as parametric polymorphism, introduce a powerful way to create reusable and type-safe code. At its core, generics allow developers to define classes, methods, or functions where certain data types are specified as parameters. This means a single method or class can operate on different data types without sacrificing type safety.
For example, consider a list. Without generics, you might need different list structures for integers, strings, or custom objects. With generics, you create a single, generic list structure that can adapt to any data type you specify.
This concept brings two main benefits. Firstly, it promotes code reusability. Developers can write a general blueprint and use it for various data types. Secondly, it maintains type safety, ensuring that only specific, predetermined types are used, reducing runtime errors.
Concurrency and Multithreading in Programming
Concurrency and multithreading are concepts that enable programs to perform multiple tasks simultaneously, improving efficiency and responsiveness. Concurrency is a broader term, referring to the ability of a system to handle several tasks at the same time, but not necessarily all at once. It’s about managing many tasks, like juggling.
Multithreading, a subset of concurrency, is more specific. It allows a program to split itself into two or more parallel threads that can run simultaneously. Imagine a conveyor belt with several workers (threads) doing tasks at the same time.
These techniques offer benefits, especially in today’s world of multi-core processors. They can make software faster or allow it to remain responsive even when part of it is busy. For instance, a web browser might continue to respond to user input even while loading a webpage on a separate thread.
However, they also introduce complexities. Developers need to manage resources shared between threads and handle potential conflicts, ensuring that operations remain safe and data stays consistent. In essence, while concurrency and multithreading boost performance, they demand careful coding and testing.
Lambda Expressions and Closures
Lambda expressions, often simply called lambdas, are anonymous functions. They don’t have a name like traditional functions. Instead, they provide a quick way to declare small functions on the fly. For instance, in many programming languages, if you want to square a number, a lambda can be written inline where it’s needed, without formally defining a full function elsewhere.
Closures, on the other hand, are functions that capture and remember the external variables from where they were created, even if their surrounding context has finished executing. Imagine a function inside another function: the inner function can “remember” the variables of the outer one, even after the outer function has ended. This capability allows developers to maintain state across various scopes.
Both lambdas and closures enhance flexibility in coding. Lambdas offer brevity, making code more readable, especially for short, single-use operations. Closures, meanwhile, provide a unique way to retain context, enabling more dynamic and responsive programming patterns. Together, they help developers craft cleaner, more efficient code.
Take a tour of C#, the language used in Unity, which is used in our guides.