Programming logic forms the bedrock of software development. It’s the art of crafting algorithms and instructions that enable a computer to solve problems and perform tasks with precision and efficiency.
We’ll explore how to think algorithmically, dissect the conditional statements and loops that govern program flow, and delve into the intricacies of data structures and functions. By the end of this journey, you’ll possess a solid grasp of programming logic, equipping you to tackle a wide range of programming challenges and build software solutions that are elegant, robust, and effective.
Logic refers to the systematic and coherent reasoning that developers apply to create algorithms and instructions for computers to follow. It involves designing the flow of instructions in a way that achieves specific objectives and solves problems effectively. Logical thinking is essential for creating code that behaves as intended, processes data accurately, and produces the desired outcomes.
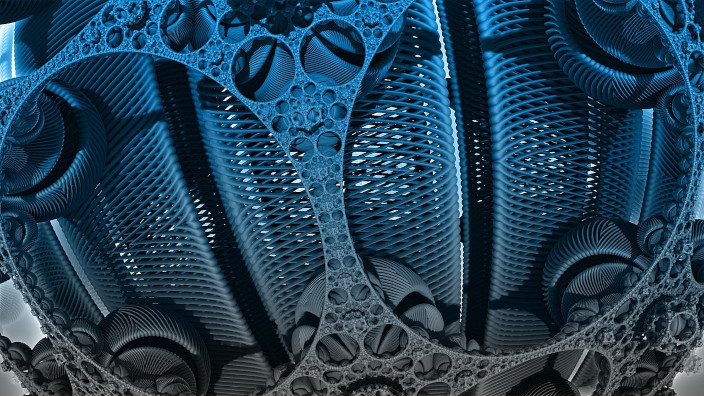
Thinking Algorithmically
Thinking algorithmically is a cornerstone skill in the world of programming and problem-solving. It’s the art of breaking down complex tasks and challenges into step-by-step, logical sequences of instructions, known as algorithms.
These algorithms serve as roadmaps that guide a computer through a series of actions to achieve a specific goal. However, algorithmic thinking is not just for computers; it’s a cognitive approach that empowers humans to solve problems more efficiently and systematically.
One of the key principles of algorithmic thinking is abstraction. This involves focusing on the essential aspects of a problem while ignoring unnecessary details. By abstracting a problem, you can create a simplified mental model that helps you identify patterns and devise strategies for solving it.
For example, when writing a sorting algorithm, you don’t need to concern yourself with the minute details of how a computer stores data; you can abstract the problem to focus solely on comparing and rearranging values.
Algorithmic thinking also emphasizes decomposition, which means breaking a problem into smaller, manageable sub-problems. This approach makes complex challenges more approachable, as you can tackle each sub-problem individually and then combine their solutions to address the overall issue. It’s akin to solving a jigsaw puzzle, where you start with small groups of connected pieces before assembling the complete picture.
Furthermore, algorithmic thinking encourages efficiency and optimization. When designing algorithms, you aim to minimize unnecessary steps and reduce computational resources, such as time and memory. This mindset fosters creative problem-solving and innovation, as you constantly seek ways to improve your algorithms’ performance.
In essence, thinking algorithmically is about developing a structured, methodical approach to problem-solving. It’s a skill that transcends programming and finds applications in various fields, from mathematics and engineering to business and everyday life. By mastering this skill, you not only become a more proficient programmer but also a more effective problem solver, capable of addressing a wide range of challenges with clarity and confidence.
Steps of Algorithmic Thinking
Algorithmic thinking involves a systematic approach to solving problems and designing algorithms. Here are the steps of algorithmic thinking:
Understanding the Problem
Begin by thoroughly understanding the problem you need to solve. Clearly define the input, output, and constraints. Take time to identify any special cases or edge conditions that need consideration.
Abstraction
Simplify the problem by abstracting away unnecessary details. Focus on the core elements and requirements. This step helps in creating a high-level overview of the problem.
Decomposition
Break the problem down into smaller, manageable sub-problems or tasks. Decomposition helps in dividing the problem into more digestible pieces that can be solved individually.
Pattern Recognition
Identify any patterns or similarities within the problem. Recognizing patterns can lead to the development of reusable solutions and can simplify the overall problem-solving process.
Algorithm Design
Design algorithms to solve each of the sub-problems. Start with a plan that outlines the logical steps to take for each part of the problem. Be sure to consider the order of operations and any conditional logic needed.
Pseudocode or Flowchart
Before implementing the solution in code, create a pseudocode or a flowchart to visualize the algorithm’s flow and logic. This step helps in refining the algorithm and identifying potential issues.
Testing and Debugging
Implement the algorithm in your chosen programming language. Test it rigorously using various inputs, including edge cases, to ensure it works as expected. Debug and refine the code as needed.
Optimization
If necessary, optimize the algorithm to improve its efficiency. This may involve reducing time complexity, minimizing memory usage, or enhancing overall performance.
Documentation
Document your algorithm, code, and any assumptions or decisions made during the problem-solving process. Clear documentation is crucial for collaboration and future reference.
Iterate and Refine
Algorithmic thinking is an iterative process. If you encounter issues or find room for improvement, go back to previous steps, adjust, and iterate until you achieve an optimal solution.
Communication
Effective communication of your algorithm and solution is vital, especially when working in a team or sharing your work with others. Clearly explain your approach, assumptions, and results.
Feedback and Continuous Learning
Seek feedback from peers or mentors to improve your algorithmic thinking skills. Continuously learn and adapt as you encounter new problems and challenges.
By following these steps, you can systematically approach problem-solving and algorithm design, making it easier to tackle complex issues and create efficient solutions.
Algorithmic Thinking Applied to a Specific Problem: Oath Finding
Here are the steps for algorithmic thinking applied to the specific problem of path finding:
Understand the Problem
Begin by understanding the path-finding problem. Identify the starting point, destination, and any obstacles or constraints within the map or environment.
Abstraction
Simplify the problem by abstracting the map or environment into a graph-like representation. Focus on the essential elements: nodes (locations) and edges (connections between locations).
Decomposition
Break down the path-finding problem into smaller sub-problems. Consider how to navigate from one node to another, potentially using algorithms like breadth-first search (BFS) or Dijkstra’s algorithm for each sub-problem.
Pattern Recognition
Recognize common patterns in path-finding problems, such as finding the shortest path between two points. Understand how different algorithms can be applied based on specific requirements, like speed vs. accuracy.
Algorithm Design
Design an algorithm or select an appropriate path-finding algorithm based on the problem’s complexity and requirements. Common algorithms include A* search, Dijkstra’s algorithm, and breadth-first search (BFS).
Pseudocode or Flowchart
Create pseudocode or a flowchart to outline the algorithm’s logic, specifying how it will explore the map, make decisions, and find the optimal path.
Testing and Debugging
Implement the selected algorithm in code and thoroughly test it with different map scenarios. Debug and refine the code to handle various edge cases and ensure correctness.
Optimization
If necessary, optimize the path-finding algorithm to improve its efficiency. This might involve data structures like priority queues or heuristic functions that guide the search.
Documentation
Document the path-finding algorithm, including its parameters, assumptions, and how it handles special cases. Clear documentation is crucial for future reference or collaboration.
Visualization
Consider visualizing the path-finding process, either through graphical representations or animations, to aid in understanding and debugging.
Communication
Effectively communicate the path-finding algorithm’s usage, limitations, and results to other team members or stakeholders. Explain how it addresses the specific problem at hand.
Feedback and Continuous Learning
Seek feedback from peers or experts in path finding and algorithm design. Continuously learn and adapt the algorithm as you encounter new path-finding challenges.
By following these steps, you can approach the path-finding problem systematically, ensuring that you develop a solution that efficiently and accurately finds optimal paths within various map or environment configurations.
The Fundamental Elements of an Algorithm
An algorithm consists of several fundamental elements that work together to solve a specific problem or perform a particular task.
Input
This is the data or information that the algorithm processes to produce a result. Input can take various forms, such as numbers, strings, arrays, or complex data structures, depending on the problem.
Output
The output represents the result or solution generated by the algorithm based on the provided input. It can be a single value, multiple values, or even modifications to the input data.
Control Structures
Control structures define the flow of the algorithm, including how it makes decisions and repeats tasks. Common control structures include conditionals (if-else statements), loops (for, while), and branching (goto or jump statements).
Operations
Operations are the individual steps or actions performed by the algorithm. These actions can involve mathematical calculations, comparisons, assignments, and more, depending on the algorithm’s purpose.
Variables
Variables are used to store and manipulate data within the algorithm. They can represent both input data and intermediate results. Variables are assigned values and can change throughout the algorithm’s execution.
Data Structures
Data structures are used to organize and manage data efficiently. Examples include arrays, lists, stacks, queues, trees, and graphs. The choice of data structure depends on the specific problem being solved.
Functions or Procedures
Algorithms often consist of modular components, such as functions or procedures, which encapsulate specific tasks or operations. These reusable components enhance code organization and readability.
Comments
Comments are explanatory notes or annotations within the algorithm’s code. They provide information about the algorithm’s purpose, logic, and any important details for human readers.
Initialization
Initialization involves setting up variables or data structures before the main execution of the algorithm begins. This step ensures that the algorithm starts with the correct initial conditions.
Termination Condition
Algorithms typically include a termination condition that specifies when the algorithm should stop executing. This condition can be based on reaching a specific result, a predefined number of iterations, or other criteria.
Error Handling
Error handling mechanisms are essential for addressing unexpected situations or errors that may occur during the algorithm’s execution. This includes handling exceptions, invalid input, or other exceptional cases gracefully.
Flow Control
Flow control statements determine the order in which operations are executed within the algorithm. These statements guide the algorithm’s logical flow and ensure that tasks are performed in the correct sequence.
Time and Space Complexity Analysis
While not directly part of the algorithm’s code, analyzing the algorithm’s time and space complexity is crucial for understanding its efficiency and performance characteristics.
These elements collectively define the structure and behavior of an algorithm, guiding its execution from input to output while ensuring correctness, efficiency, and readability. The choice and arrangement of these elements depend on the specific problem domain and the algorithm’s intended purpose.
Fundamental Elements for the Path Finding Algorithm
Here are the steps for designing an algorithm for path finding in a 2D 16×16 grid.
Understand the Grid
Begin by understanding the 2D 16×16 grid, including its dimensions (16 rows and 16 columns). Recognize that this grid represents the environment in which you need to find a path.
Define the Start and End Points
Identify the coordinates of the starting point and the destination point within the grid. These coordinates will serve as the input for the path-finding algorithm.
Create the Grid Representation
Develop a data structure to represent the grid. In this case, a 2D array (or matrix) can be used, with each cell representing a location in the grid and containing information about its accessibility (e.g., obstacles, open spaces).
Initialize Data Structures
Initialize data structures to store information about visited cells, the current path, and any other relevant data needed during the path-finding process.
Algorithm Selection
Choose an appropriate path-finding algorithm based on the grid’s characteristics. Common choices include A* search, Dijkstra’s algorithm, or breadth-first search (BFS).
Pseudocode or Flowchart
Create pseudocode or a flowchart outlining how the selected algorithm will explore the grid, make decisions, and identify the optimal path from the starting point to the destination.
Implement the Algorithm
Write the code for the selected path-finding algorithm, incorporating the grid representation and the logic defined in the pseudocode or flowchart.
Testing and Debugging
Test the algorithm with various grid scenarios, including obstacles and different start-to-end point combinations. Debug and refine the code to ensure it handles grid-specific cases correctly.
Optimize for Grid Size
Optimize the algorithm for efficiency, considering the size of the 16×16 grid. Assess its time and space complexity to ensure it performs well for larger or more complex grids.
Output Path
Design a mechanism to extract and display the path found by the algorithm on the grid, highlighting the optimal route from the start to the end point.
Documentation
Provide documentation for your path-finding algorithm, explaining its usage, inputs, outputs, and any grid-specific considerations. Include any assumptions or limitations relevant to this specific grid.
Visualization
Consider creating a visualization of the path-finding process on the 16×16 grid, which can be helpful for understanding and debugging the algorithm’s behavior.
By following these steps tailored to the 2D 16×16 grid context, you can design an algorithm that efficiently finds paths within this specific grid configuration, allowing you to navigate from the starting point to the destination while avoiding obstacles.
Key Considerations for Effective Algorithm Design
Whether you’re solving complex problems or optimizing processes, considering the following aspects is essential to create effective and efficient algorithms:
Problem Understanding
Before diving into algorithm design, thoroughly understand the problem you’re trying to solve. Define the problem’s scope, requirements, constraints, and expected outcomes. A deep understanding of the problem is the foundation of a successful algorithm.
Input and Output
Clearly define the format and type of input data your algorithm will accept and specify the expected output. Understanding data structures and formats is crucial, as it influences how your algorithm processes and delivers results.
Efficiency
Consider the algorithm’s time and space complexity. Strive for efficiency by minimizing unnecessary operations, iterations, and memory usage. Analyze and optimize your algorithm for the best possible performance.
Scalability
Ensure your algorithm can handle input data of varying sizes. Test it with both small and large datasets to verify that it remains efficient as the input scales. Scalability is especially important for real-world applications.
Correctness
Your algorithm must produce correct results. Thoroughly test it with various inputs, including edge cases and corner cases, to validate its accuracy. Consider using formal methods, assertions, or testing frameworks to ensure correctness.
Robustness
Design algorithms that can handle unexpected situations gracefully. Implement error-handling mechanisms to address exceptions, invalid input, and unexpected conditions. This prevents crashes and enhances the algorithm’s reliability.
Optimality
Aim for optimality in your algorithm, especially in optimization problems. Determine whether your algorithm produces the best possible solution or if there’s room for improvement. Striking a balance between complexity and optimality is essential.
Data Structures
Select appropriate data structures based on the problem’s requirements. Choosing the right data structure can significantly impact the algorithm’s efficiency and ease of implementation.
Algorithm Paradigm
Explore different algorithmic paradigms, such as divide and conquer, dynamic programming, greedy algorithms, and graph algorithms. Select the paradigm that best suits the problem at hand.
Code Readability
Write clear and well-structured code. Use meaningful variable names, comments, and indentation to enhance code readability. A well-documented algorithm is easier to understand and maintain.
Reusability
Encapsulate common tasks or components into functions or modules for reuse. Reusable code promotes code maintainability and reduces redundancy in your algorithm.
Documentation
Document your algorithm thoroughly. Explain its purpose, input, output, and any assumptions or limitations. Clear documentation facilitates collaboration and future maintenance.
Parallelism
Consider whether your algorithm can be parallelized to take advantage of multi-core processors or distributed computing environments. Parallel algorithms can improve performance significantly for certain tasks.
Real-world Constraints
Take into account real-world constraints, such as hardware limitations, network latency, and user experience. An algorithm should be practical and usable within its intended context.
Feedback and Refinement
Seek feedback from peers, experts, or users. Iteratively refine your algorithm based on feedback and new insights. Algorithm design is often an evolving process.
Ethical Considerations
Consider ethical implications, especially when dealing with data or decision-making algorithms. Ensure fairness, transparency, and accountability in your algorithm’s design and implementation.
By carefully considering these factors during algorithm design, you can create solutions that not only solve problems effectively but also meet performance expectations, maintainability requirements, and ethical standards.
Take a tour of C#, the language used in Unity, which is used in our guides.