Have you ever wondered what lies beneath the magic of a truly engaging video game? You know, the kind that keeps players hooked for hours, immersed in a world of excitement, challenge, and unpredictability. The answer, my friends, often lies in the mastery of gameplay dynamics, and one powerful tool in our arsenal for achieving this mastery is Bayesian Networks.
In this article, we’ll take a deep dive into the fascinating world of Bayesian Networks and explore how they can be a game-changer (literally!) for indie game developers. We’ll break down complex concepts into bite-sized pieces and provide real-world examples that highlight their effectiveness.
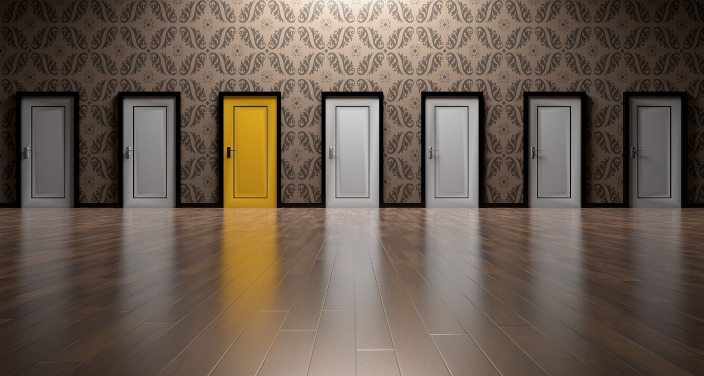
Understanding Bayesian Networks
Before we embark on our journey into the world of Bayesian Networks in video games, let’s start with the basics. If you’re not already familiar with Bayesian Networks, fear not; we’ll break it down in a friendly and accessible manner.
What Are Bayesian Networks?
Imagine you’re playing a game, and you have to make a decision that will affect the outcome. You consider various factors, like your character’s health, the strength of your opponent, and the weapon you’re wielding. Bayesian Networks are like a smart, behind-the-scenes helper that helps your game understand and make decisions based on these factors.
At their core, Bayesian Networks are graphical models that represent relationships between different pieces of information or events. These models consist of two main elements:
Nodes
Nodes are like information hubs. Each node represents something meaningful in the game, such as the health of a character, the probability of finding loot, or the likelihood of an enemy attacking. Think of nodes as circles or ovals in our graphical representation.
Edges
Edges are the connections between nodes and represent how one piece of information affects another. They are like arrows that show the direction of influence between nodes. If one node’s value changes, it can impact the values of other connected nodes.
Conditional Probability
The magic of Bayesian Networks lies in conditional probability. Each node in the network has a conditional probability distribution associated with it. This distribution tells the game how likely different values of that node are, given the values of its parent nodes.
Let’s simplify this with a real-world example: shooting in a first-person shooter game. If you’re trying to shoot an enemy, the accuracy of your shot might depend on factors like your character’s skill level, the type of weapon you’re using, and the distance to your target. These factors are the parent nodes that influence the accuracy node.
The conditional probability distribution for accuracy would tell the game how likely you are to hit your target based on the values of these parent nodes. If you have a high-skill character and a powerful weapon, your accuracy is more likely to be on target.
Now that we’ve laid the groundwork, you might be wondering, “How does all this relate to video games?” Well, that’s precisely what we’ll explore in the next section as we delve into the role of Bayesian Networks in shaping the dynamics of video games.
Bayesian Networks: Simple Example
//To add the necessary packages. Run this command in the NuGet Package console.
//dotnet add package Microsoft.ML.Probabilistic.Compiler
using Microsoft.ML.Probabilistic.Models;
using Microsoft.ML.Probabilistic.Distributions;
class Program
{
static void Main()
{
// Define player skill and game difficulty as random variables
Variable<double> playerSkill = Variable.New<double>().Named("PlayerSkill");
Variable<double> gameDifficulty = Variable.New<double>().Named("GameDifficulty");
// Define prior distributions for player skill and game difficulty
// These are our initial beliefs about the player's skill and game difficulty.
// In practice, these could be based on historical data or subjective assessments.
playerSkill.SetTo(Variable.GaussianFromMeanAndPrecision(0.7, 0.1));
gameDifficulty.SetTo(Variable.GaussianFromMeanAndPrecision(0.5, 0.1));
// Define a model for the likelihood of winning the game based on player skill and game difficulty
// In this simple model, we assume that the player wins if their skill is greater than the game's difficulty.
Variable<bool> winGame = (playerSkill > gameDifficulty);
// Create an inference engine
var inferenceEngine = new InferenceEngine();
// Perform inference to calculate the probability of winning the game
// We use the inference engine to estimate the probability of winning the game based on our model.
// This represents the updated belief after considering the player's skill and game difficulty.
double probabilityOfWinning = inferenceEngine.Infer<Bernoulli>(winGame).GetProbTrue();
// Display the result
Console.WriteLine("Probability of winning the game: " + probabilityOfWinning.ToString("0.00"));
}
}
Bayesian Networks in Video Games
Now that we’ve grasped the fundamentals of Bayesian Networks, let’s dive into why they matter so much in the world of video game development, particularly for indie game developers.
The Challenge of Crafting Engaging Gameplay
Indie game development is a unique beast. It often involves small teams with limited budgets, competing in a vast and competitive market. To stand out and succeed, indie games need something special—captivating gameplay that keeps players coming back for more.
However, crafting engaging gameplay is no small feat. It requires an intricate balance of challenge and reward, surprise and predictability, immersion and agency. Players today have high expectations, and they can quickly abandon a game if it doesn’t offer a compelling experience.
Real-World Examples
Learning from real-world examples is often the best way to understand the practical applications of a concept. In this section, we’ll delve deeper into video games that have successfully harnessed the power of Bayesian Networks to create engaging and dynamic gameplay experiences.
F.E.A.R. (First Encounter Assault Recon): Adaptive Enemy AI
If you’ve ever played F.E.A.R., you’ve likely been awed (and occasionally terrified) by the seemingly intelligent behavior of the enemy soldiers. The game’s developers achieved this by implementing Bayesian Networks to govern the actions of these adversaries.
How It Works: In F.E.A.R., Bayesian Networks analyze the player’s behavior, such as movement patterns, use of cover, and weapon choice. Based on this analysis, the enemy AI dynamically adjusts its tactics. If you frequently hide behind cover, enemies may attempt to flank you. If you’re aggressive and push forward, they’ll take cover and lay suppressing fire. This adaptability ensures that the gameplay remains challenging and unpredictable, even on repeated playthroughs.
The Sims: Guiding Virtual Lives
The Sims series has been a beloved franchise for years, captivating players with its sandbox-style gameplay and lifelike character behavior. What you may not realize is that Bayesian Networks play a pivotal role in driving the actions of your virtual inhabitants.
How It Works: Each Sim in the game has its own set of desires, needs, and preferences. Bayesian Networks model the relationships between these factors and guide Sim behavior. For example, a Sim might decide to cook dinner because they’re hungry, but their choice of dish may depend on their culinary skill level and personal preferences. This creates a dynamic and diverse array of behaviors among Sims, making each gameplay experience unique.
Plague Inc.: Simulating Global Pandemics
In the strategy game Plague Inc., your goal is to infect and ultimately wipe out humanity with a deadly pathogen. The game’s simulation of disease spread is surprisingly accurate, thanks to the use of Bayesian Networks.
How It Works: Bayesian Networks in Plague Inc. model the complex interactions that determine how diseases spread. Factors such as transmission vectors, symptoms, and resistance to treatment are all interconnected in a web of probabilities. As you make choices to evolve your pathogen, the Bayesian Network calculates the consequences, leading to a compelling and challenging simulation of a global pandemic.
These real-world examples illustrate the versatility of Bayesian Networks in shaping various aspects of gameplay, from enemy AI behavior and character interactions to complex simulations. For indie game developers, the lesson is clear: by harnessing Bayesian Networks, you can create games that adapt, surprise, and captivate your players. But how can you implement these networks in your own projects? In the next section, we’ll explore practical steps for doing just that.
Implementing Bayesian Networks in Indie Games
Now that we’ve seen the magic of Bayesian Networks in action through real-world examples, you might be eager to implement them in your own indie game projects. Good news – we’re about to explore the practical steps for bringing Bayesian Networks into your game development toolkit.
Identifying Gameplay Elements to Model
The first step is to identify which aspects of your game can benefit from Bayesian modeling. Consider elements where uncertainty, player choices, or dynamic responses are crucial. These could include:
Enemy AI behavior: Like in F.E.A.R., you can use Bayesian Networks to make your game’s enemies adapt to the player’s tactics.
Character interactions: If your game involves NPCs (non-player characters), you can use Bayesian Networks to model how they respond to the player’s actions and choices.
Resource management: For strategy or simulation games, Bayesian Networks can simulate resource allocation, trade, or economic systems.
Environmental changes: In open-world games, use Bayesian Networks to control weather patterns, day-night cycles, or other dynamic elements.
Data Collection and Analysis
To build a Bayesian Network, you need data. Collect information about how different game variables interact and affect each other. This could involve player behavior data, gameplay statistics, or other relevant metrics.
Once you have your data, perform analysis to determine the probabilities and conditional dependencies between variables. This step is crucial for setting up accurate Bayesian Networks that reflect the dynamics of your game world.
Integration with Your Game Engine
Most game development environments provide tools and libraries for working with probabilistic models like Bayesian Networks. Depending on your chosen engine (Unity, Unreal Engine, Godot, etc.), you may need to adapt or create custom solutions for integrating Bayesian Networks into your game.
Iterative Testing and Fine-Tuning
Once your Bayesian Networks are integrated into your game, it’s essential to iterate and test extensively. Gather feedback from playtesting to ensure that the dynamic elements feel balanced and engaging.
Adjust the conditional probabilities and relationships within your Bayesian Networks to fine-tune gameplay dynamics.
Consider implementing player feedback mechanisms that allow your game to adapt not only to player actions but also to their preferences and enjoyment.
Continuously analyze player data to improve the accuracy and effectiveness of your Bayesian Networks.
By following these steps and being willing to iterate and refine your models, you can effectively integrate Bayesian Networks into your indie games, enhancing their depth, immersion, and player engagement.
In the next section, we’ll explore how to optimize gameplay with Bayesian Networks, ensuring that they enhance the player experience rather than overwhelming it.
Optimizing Gameplay with Bayesian Networks
Implementing Bayesian Networks in your indie game is just the first step. To truly master gameplay dynamics, it’s crucial to optimize your use of Bayesian Networks. Here, we’ll discuss strategies for fine-tuning your models to create a captivating and balanced player experience.
Balancing Difficulty
One of the most powerful applications of Bayesian Networks in gaming is dynamically adjusting the difficulty to match the player’s skill level. Here’s how you can achieve this:
Skill-based difficulty: Analyze player performance data and adjust enemy AI behavior or environmental challenges to provide an appropriate level of challenge. If players are struggling, make the game slightly easier; if they’re breezing through, ramp up the difficulty.
Adaptive learning: Implement Bayesian Networks that learn from player actions over time. This allows your game to adapt to a player’s playstyle and preferences.
Dynamic pacing: Use Bayesian Networks to control the pacing of your game. For example, in a survival horror game, adjust the frequency and intensity of scares based on player reactions.
Player Experience Enhancement
Bayesian Networks can also be employed to personalize gameplay experiences, making players feel more connected to the game world:
Tailored narratives: Create branching storylines that adapt to player choices and actions. Bayesian Networks can determine the consequences of decisions, ensuring that the narrative remains coherent and engaging.
Procedural content generation: Generate game content, such as levels, quests, or challenges, using Bayesian Networks. This can lead to more varied and interesting gameplay experiences.
Adaptive tutorials: Use Bayesian Networks to identify the player’s level of familiarity with the game mechanics and provide tutorials or hints when needed.
Dynamic sound and music: Adjust the game’s audio elements, including music, sound effects, and voiceovers, to match the current mood and situation in the game, enhancing immersion.
Remember that the key to success is finding the right balance. You want to challenge players enough to keep them engaged but not frustrate them to the point of quitting. Similarly, personalization should enhance the experience without feeling invasive or forced.
Common Pitfalls and Challenges
While Bayesian Networks offer tremendous potential, there are some common pitfalls and challenges to be aware of:
Overly complex models: Avoid creating overly complex Bayesian Networks that become unwieldy and difficult to fine-tune.
Data scarcity: If you don’t have enough data to build accurate models, your Bayesian Networks may not perform as expected. Consider methods for data augmentation or simplifying your models.
Performance concerns: Be mindful of the computational resources required for Bayesian Network calculations, especially in real-time applications. Efficient algorithms and optimizations can mitigate this issue.
By navigating these challenges and continually testing and refining your Bayesian Network implementations, you can optimize gameplay dynamics to create a gaming experience that resonates with players and keeps them coming back for more.
In our next section, we’ll conclude our exploration by looking at future trends in the use of Bayesian Networks in video games. Stay tuned to stay ahead in the ever-evolving world of game development!
Future Trends
As technology and game development practices continue to evolve, so too will the role of Bayesian Networks in shaping the future of video games. Let’s take a glimpse into the crystal ball and explore some exciting trends on the horizon:
Machine Learning Integration
The integration of machine learning (ML) and Bayesian Networks holds enormous potential for the gaming industry. ML algorithms can help optimize the parameters of Bayesian Networks in real-time, making gameplay dynamics even more adaptive and engaging. For example, ML can be used to predict player behavior and preferences, allowing games to proactively tailor experiences.
Enhanced Player Agency
Future games are likely to offer even greater player agency. Bayesian Networks can play a central role in this evolution, enabling games to respond dynamically to player choices and actions. This means not only branching narratives but also more complex and nuanced reactions from NPCs, environment interactions, and gameplay mechanics.
Cross-Platform Adaptation
With gaming experiences spanning across multiple platforms and devices, Bayesian Networks can facilitate seamless cross-platform adaptation. Games can analyze player behavior on one platform and carry over insights to provide a consistent experience on another. Imagine starting a game on your console and continuing the adventure on your mobile device, with the gameplay dynamics adjusting accordingly.
Emergent Storytelling
The lines between traditional storytelling and emergent storytelling will continue to blur. Games will increasingly leverage Bayesian Networks to craft stories that evolve dynamically based on player choices, creating unique narratives for each player. This approach not only enhances replayability but also deepens player immersion.
AI-Driven NPCs and Foes
Expect AI-controlled characters and enemies to become even more lifelike. Bayesian Networks, coupled with advanced AI techniques, will enable NPCs to exhibit complex emotions, make decisions influenced by their unique traits and experiences, and adapt to changing circumstances in ways that feel uncannily human.
Ethical Considerations
As games become more sophisticated in their use of player data and behavioral analysis, ethical considerations will become increasingly important. Game developers will need to balance the benefits of personalization with player privacy and consent, addressing concerns about data usage and player profiling.
These trends represent a glimpse into the exciting future of Bayesian Networks in video games. As indie game developers, embracing these advancements can help you create cutting-edge gaming experiences that captivate and resonate with players in ways previously unimagined.
Conclusion
In our journey through the world of Bayesian Networks in video games, we’ve seen how this powerful tool can transform gameplay dynamics, enhancing player experiences and keeping them engaged.
From adapting enemy AI behavior to crafting dynamic narratives and personalizing gameplay, Bayesian Networks offer a world of possibilities for indie game developers. While challenges exist, they are surmountable with dedication and creativity.
As you embark on your game development endeavors, remember that mastering gameplay dynamics is a continuous journey. Stay curious, keep experimenting, and embrace emerging technologies to stay at the forefront of game development.
May your future projects be filled with dynamic and unforgettable gaming experiences, all thanks to the mastery of Bayesian Networks. Happy game development!
If you enjoyed this read, you might also find this article interesting.
Machine Learning Through Probabilistic Programming, Microsoft Learn.