Component-Based Development (CBD) is a software development approach that focuses on creating software by assembling reusable and self-contained components or modules. In game development, component-based development is widely used to create flexible, modular, and reusable game systems.
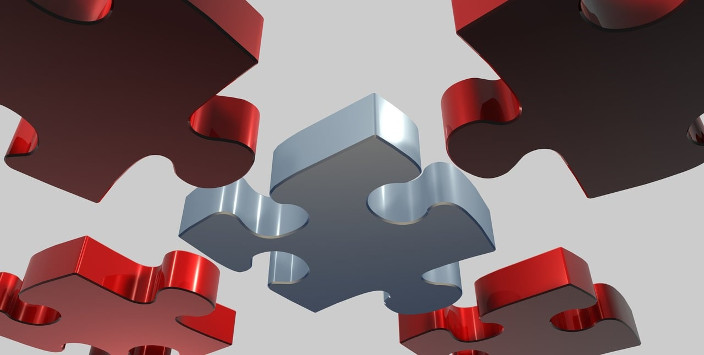
Key Concepts
Components
Components are self-contained units of functionality that encapsulate specific features or behaviors. Each component focuses on a single responsibility and can be reused across different parts of the game.
Each component encapsulates a specific functionality or set of functionalities and can operate independently. This design principle prioritizes modularization and promotes reusability, leading to potentially more manageable and scalable systems.
A component is a modular, deployable, and replaceable part of a system that encapsulates functionality and behaviors. It communicates with other components through well-defined interfaces. By standardizing the interfaces, various components can be plugged together to create different systems or applications.
An interface defines how a component will interact with other parts of the system. It specifies the methods, properties, and events that are available for other components or systems to use. Adhering to defined interfaces ensures that components can be easily swapped or updated without affecting the rest of the system.
A component framework provides the runtime environment in which components operate. Frameworks handle component lifecycle, manage the interactions between components, and sometimes provide services such as security, transactions, and persistence. Examples include the .NET framework, Java’s Spring, and Enterprise JavaBeans (EJB).
A component repository or library is a centralized place where developed components are stored, cataloged, and made available for reuse. By maintaining a repository, developers can search for and reuse components that have already been developed, tested, and validated, speeding up software development and ensuring consistent behavior.
The process of combining multiple components to form a complete system or application is called composition. In CBSD, the emphasis is on assembling pre-built components rather than coding new functionalities from scratch. Composition tools or software might be used to visually combine components, define interactions, and configure application behavior.
In the context of CBSD, a contract defines the rules and specifications that a component must adhere to. This includes its interface definition, expected behavior, and any other conditions or constraints. By maintaining clear contracts, components can remain interchangeable and maintain system integrity as changes occur.
Composition
In component-based software design (CBSD), composition is a foundational concept. It refers to the act of assembling individual, reusable components to form a complete system or application. Unlike traditional development where software is written from scratch, CBSD emphasizes on assembling pre-built and often pre-tested components, much like constructing a building using Lego blocks.
Composition involves taking individual components, each encapsulating specific functionality, and combining them to produce more complex behavior or to achieve broader system functionalities. For example, in a web application, one component might handle user authentication, another might manage database interactions, and yet another might deal with displaying content. By composing them, a complete web application that takes a user from login to viewing content can be created.
For composition to be effective, each component must have well-defined interfaces that specify how they interact with other components. These interfaces are like contracts that ensure components can be easily connected or integrated with one another without unexpected behaviors.
One of the significant advantages of composition in CBSD is that components can be rearranged, replaced, or updated without disturbing the entire system. If a particular component needs an upgrade or if a more efficient component becomes available, it can be swapped out with minimal impact on the surrounding components, given the interactions are maintained through the defined interfaces.
Many modern software development environments offer visual tools that aid in component composition. These tools allow developers to visually drag, drop, and connect components, making it easier to design, understand, and modify the overall structure of a software application.
Composition promotes modularity and reusability. Software developers can focus on creating highly specialized components that do one thing and do it well. When a new project or feature requires that functionality, instead of rewriting the code, developers can simply use (or compose) the existing component. This not only speeds up the software development process but also leads to more robust systems since components are often tested and refined over multiple uses.
Entity-Component System (ECS)
Entity-Component Systems (ECS) is a specific architectural pattern that has gained popularity, especially in game development, due to its flexibility and performance advantages.
Entities are the primary objects in the game or system. However, unlike traditional object-oriented design, entities in ECS are often just unique identifiers, with little to no behavior or data of their own. For example, a spaceship, an alien, and a bullet in a game might all be entities.
Components, on the other hand, are data structures that represent various aspects or attributes of entities but don’t typically include behavior. For instance, a Position component might store x, y, and z coordinates. A Render component might contain information about textures and meshes. If an entity is meant to have a certain attribute, it’s given the corresponding component. So, a spaceship entity might have Position, Render, and Health components, while a bullet might only have Position and Render components.
Finally, systems are where the behavior or logic resides in ECS. They operate on entities that have specific component combinations. For instance, a MovementSystem might look for all entities with both Position and Velocity components, and update their positions based on their velocities. Systems allow for decoupling of logic from data, leading to more maintainable and scalable code.
The separation of concerns in ECS offers several advantages. ECS often aligns better with modern computer architectures, particularly when it comes to data locality. This means that when a system processes multiple components, they are often closely packed in memory, making operations more cache-efficient.
It’s easy to add, remove, or modify features. If a developer wants to introduce a new attribute to an entity, they can simply add a new component to it. Similarly, new behavior can be introduced by adding a new system without modifying existing code. Components and systems can be reused across different entities and even different games. This modular approach reduces code redundancy and accelerates development.
In the context of game development, ECS facilitates the construction of complex, interactive worlds. Games inherently involve numerous entities interacting in varied ways, and traditional object-oriented approaches can quickly become unwieldy. ECS provides a cleaner, more organized approach, making it easier for developers to add features, optimize performance, and manage the game’s complexity.
Component Lifecycle
The process of creating components in component-based software design (CBSD) is strategic and meticulous. Components are standalone units of functionality meant to be reusable, modular, and integrable with other components. The journey starts by understanding the software requirements. Determine the functionalities the software should provide and how they could be split into independent, logical units. These units are the potential candidates for components.
Read more about Requirement Analysis here.
Once requirements are understood, starts the component identification of which parts of the software can be modularized into individual components. Consider aspects like reusability, logical independence, and the single responsibility principle. Each component should do one thing and do it well.
Component identification is followed by component specification. In this phase, the purpose and behavior of the component is defined. This includes detailing what the component should do, its inputs, outputs, and its interaction mechanisms (interfaces). This step often involves designing the component’s public interface, which other components or systems will use to interact with it.
With the specifications in hand, the internal workings of the component can be designed. This involves deciding data structures, algorithms, and internal logic. The component is then coded using a suitable programming language. While the internal workings can be complex, the external interface should remain as simple and intuitive as possible.
Before a component is deemed reusable, it must be rigorously tested. This ensures that it behaves as expected and can handle a range of inputs and scenarios. Component testing focuses on testing the component in isolation from the rest of the system. For a component to be reusable, it’s not enough that it works well; developers must understand how to use it. Proper documentation outlines the component’s purpose, how it should be integrated, any dependencies it has, and details about its interfaces.
Once tested and documented, the component can be packaged (often as a library or a module) and stored in a component repository. This repository acts as a central location where developers can find and reuse components across different projects. As software evolves, components might need updates or modifications. Proper versioning is essential so that any changes to the component don’t unintentionally break systems that rely on older versions.
Advantages of Component-Based Development in Game Development
Modularity and Reusability: Components can be reused across different game entities, promoting code reusability and reducing redundancy.
Flexibility: Game objects can be constructed by mixing and matching different components, allowing for dynamic customization of gameplay and features.
Maintenance: Changes to one component don’t necessarily impact others, making maintenance and updates more manageable.
Collaboration: Different developers or teams can work on different components in parallel, promoting collaboration and specialization.
Challenges of Component-Based Development in Game Development
Design Complexity: Planning and defining component interactions and dependencies can become complex, especially in large games.
Performance Overhead: The additional abstraction and communication overhead between components can introduce a slight performance cost.
Integration: Integrating and managing various components within a game entity or system requires careful design and consideration.
Debugging: Debugging issues related to component interactions and dependencies can be more intricate due to the distributed nature of components.
Characteristics of Components
Modularity: Components are self-contained and encapsulate a specific set of functionalities or features. This modularity allows for easier development, testing, and maintenance.
Reusability: Components are designed to be reusable across different parts of the software or even in different projects. This reduces redundancy and promotes efficient development.
Independence: Components are relatively independent, meaning that changes to one component shouldn’t heavily impact others. This isolation makes it easier to modify or replace components without affecting the entire system.
Interchangeability: Components can often be swapped with other components that offer similar functionality without significant changes to the rest of the system. This flexibility aids in adapting the system to changing requirements.
Types of Components
User Interface (UI) Components
User Interface (UI) components are essential elements in software applications, including games, that allow users to interact with and navigate through the application’s interface. In the context of games, UI components play a crucial role in providing players with information, feedback, and controls that enhance the overall gaming experience.
Types of UI Components
Buttons: Buttons are interactive elements that players can click or tap to trigger specific actions, such as starting the game, pausing, or accessing menus.
Text Elements: Text components display information to the player, including game status, score, dialogues, instructions, and more.
Images and Icons: Visual elements like images, icons, and illustrations enhance the visual appeal of the UI and can represent items, characters, or concepts.
Menus: Menus provide players with options to navigate through different game modes, settings, and features. They can include main menus, pause menus, options menus, and more.
HUD (Heads-Up Display): The HUD displays real-time information directly on the game screen, such as health bars, minimaps, ammo counts, and player indicators.
Sliders and Bars: Sliders and progress bars allow players to adjust settings (e.g., volume) or visualize progression (e.g., loading bars).
Checkboxes and Radio Buttons: These components enable players to make selections from a list of options, such as enabling or disabling certain game features.
Key Considerations for UI Components
Usability: UI components should be designed with usability in mind, ensuring they are intuitive and easy to interact with.
Consistency: Maintaining a consistent visual style and layout across UI components creates a cohesive user experience.
Accessibility: Designing UI components with accessibility features, such as adjustable text size and high contrast options, ensures inclusivity for all players.
Performance: UI components should be optimized for performance to prevent delays or lags in player interactions.
Responsive Design: UI components should adapt well to various screen sizes and resolutions, including those of different devices and platforms.
Integration with Gameplay
Game State Feedback: UI components provide feedback to players about their progress, health, score, and other relevant game information.
Interaction: UI components facilitate player interaction by enabling them to control the game’s flow, settings, and actions.
Immersive Elements: UI components can be designed to blend seamlessly with the game’s theme and aesthetics, enhancing the overall immersion.
UI components play a vital role in guiding players, providing crucial information, and enhancing engagement in games. Effective UI design considers both functionality and aesthetics, ensuring that players can easily navigate, interact with, and enjoy the game’s interface.
Read more about Interface Design here.
Game logic Components
Game logic Components are a fundamental part of software development, including game development. These components encapsulate the core rules, algorithms, and processes that define the behavior and functionality of a game.
Role of Game logic Components
Game Rules and Logic: Game logic components define the rules and mechanics that govern gameplay. This includes things like character movement, combat systems, resource management, and win/lose conditions.
Game Progression: Game logic components determine how the game progresses as players achieve objectives, complete challenges, and move through levels or stages.
AI Behavior: Components that control artificial intelligence (AI) behavior, such as enemy actions, pathfinding, and decision-making, are part of the game’s Game logic.
Event Handling: Logic components handle various events triggered by player actions, game state changes, or external inputs. They determine the appropriate responses and outcomes.
Examples of Game logic Components
Gameplay Manager: This component orchestrates the overall flow of the game, managing transitions between menus, levels, and gameplay states.
Combat System: A complex Game logic component that governs how combat is handled, including damage calculations, hit detection, and character interactions.
Inventory System: Manages player inventory, item interactions, and resource management, handling the acquisition, usage, and disposal of in-game items.
Quest/Task System: Controls the progression of quests, objectives, and tasks for players to complete, ensuring the game’s narrative and objectives are followed.
Resource Management: Manages in-game resources such as currency, energy, or building materials, determining how they are earned, spent, and utilized.
Design Considerations
Modularity: Game logic components should be designed in a modular manner, allowing for easy updates and changes without affecting unrelated parts of the game.
Separation from UI: To maintain clean architecture, Game logic should be separate from user interface (UI) components, allowing for independent updates and changes.
Reusability: Well-designed Game logic components can be reused across different game projects, saving development time and effort.
Testing and Debugging
Game logic components should be thoroughly tested and debugged to ensure they work as intended and provide a consistent gameplay experience.
Integration with Other Components
User Interface: Game logic components communicate with UI components to display relevant information, status updates, and gameplay feedback.
AI and Physics: Game logic components influence AI behavior and physics simulations by providing the rules and conditions for these systems to function.
Event Systems: Event-driven architectures can connect Game logic components to event systems, allowing them to respond to various events triggered during gameplay.
Game logic Components are the heart of a game, defining how the game behaves, reacts to player inputs, and maintains its internal state. Careful design and implementation of these components are essential for creating engaging and immersive gameplay experiences.
Read more about Algorithms and Logic Design here.
Data Access Components
Data Access Components are essential elements in software development that facilitate the interaction between the application and data storage systems, such as databases, files, APIs, and external services. In game development, data access components play a critical role in managing and manipulating game data, including player profiles, levels, achievements, and more.
Role of Data Access Components
Data Retrieval: Data access components retrieve information from various sources, such as databases or external APIs, to provide the game with the necessary data to function.
Data Storage: These components handle the storage of game-related data, ensuring that player progress, scores, settings, and other information are safely stored and retrieved.
Data Manipulation: Data access components enable the modification, updating, and manipulation of game data, supporting features like saving game progress or recording high scores.
Caching and Performance: To improve performance, data access components often incorporate caching mechanisms to reduce the need for frequent requests to external data sources.
Examples of Data Access Components
Database Connector: This component connects the game to a database system, allowing it to retrieve, store, and manage game-related data.
File Handler: Manages reading from and writing to files, which can be used to store configuration data, save games, or other persistent data.
API Integrations: These components facilitate interactions with external services and APIs, such as social media integration, online leaderboards, and multiplayer functionality.
Networking Libraries: In online games, networking libraries handle data transmission and communication between players and servers.
Design Considerations
Abstraction: Data access components should provide a clear and consistent interface that abstracts the underlying data storage implementation details.
Security: Ensuring data security and implementing proper authentication and authorization mechanisms is crucial to protect sensitive game data.
Optimization: Data access components should be optimized for performance to minimize delays when reading or writing data.
Error Handling: These components should include robust error handling mechanisms to gracefully handle unexpected situations, such as network outages or data corruption.
Integration with Other Components
Business Logic: Data access components are integrated with business logic components to provide the necessary data for gameplay mechanics and progression.
User Interface: UI components may display information retrieved from data access components, such as player profiles, high scores, and achievements.
Event Systems: Data access components can interact with event systems to trigger updates or actions based on changes in game data.
Data Access Components ensure that game data is effectively managed, stored, and accessed, enhancing the player experience by enabling features like progression tracking, saving and loading game states, and providing personalized content.
Read more about Data Design here.
Communication Components
Communication Components are crucial elements in software development, including game development, that enable communication and interaction between different parts of a system or between different systems. In the context of games, communication components facilitate interactions between players, between players and servers, and between various game systems.
Role of Communication Components
Player Interactions: Communication components enable players to interact with each other, exchange messages, collaborate, or compete in multiplayer and online games.
Server Communication: These components facilitate communication between players and game servers, allowing for real-time updates, synchronization, and multiplayer gameplay.
Event Broadcasting: Communication components can broadcast events, notifications, or messages to different parts of the game, triggering specific actions or behaviors.
External Integrations: These components enable integration with external services, APIs, and platforms, such as social media sharing, analytics, and payment gateways.
Examples of Communication Components
Networking Libraries: These libraries provide the necessary tools and protocols to establish connections and transmit data over networks, whether for multiplayer or client-server interactions.
Chat Systems: Chat systems are communication components that enable players to send text or voice messages to each other in real time.
Multiplayer Frameworks: These components manage the complexities of player interactions in multiplayer games, including synchronization, matchmaking, and peer-to-peer communication.
Push Notifications: Communication components for mobile games can handle push notifications to keep players engaged and informed even when they’re not actively playing.
Design Considerations
Scalability: Communication components should be designed to handle varying levels of traffic, from a few players to large-scale multiplayer environments.
Reliability: Ensuring reliable communication is crucial for maintaining a smooth player experience, even in cases of network disruptions or player fluctuations.
Security: Communication components should incorporate encryption and authentication mechanisms to protect player data and prevent unauthorized access.
Latency Management: Minimizing latency is essential for real-time interactions, especially in fast-paced games where even a small delay can affect gameplay.
Integration with Other Components
Game Logic: Communication components interact with game logic components to ensure that player actions and decisions are communicated accurately to the game world.
User Interface: UI components can display messages, notifications, or updates received from communication components.
Data Access: Communication components might work in tandem with data access components to retrieve and update player profiles, progress, and achievements.
Communication Components are a crucial part of modern games, enabling multiplayer experiences, social interactions, and real-time updates. These components contribute to the immersive and dynamic nature of contemporary games by allowing players to connect, compete, and collaborate with each other across various platforms and devices.
Utility Components
Utility Components, also known as utility classes or helper classes, are essential elements in software development that provide general-purpose functionalities and tools that can be used across different parts of an application. In game development, utility components are used to encapsulate common functionalities that are not specific to a particular domain but are needed in various parts of the game.
Role of Utility Components
Code Reusability: Utility components encapsulate reusable code that can be utilized across different parts of the game without duplicating the same logic.
General-Purpose Functionality: These components provide functions and tools that are not tied to a specific game feature but can be handy in various situations.
Simplifying Complex Operations: Utility components can simplify complex operations or calculations, making code more concise and easier to maintain.
Examples of Utility Components
Math Utilities: Utility classes that provide common mathematical operations, such as vector manipulation, collision detection, or interpolation.
Time and Timer Utilities: Components that manage time-related operations, including handling timers, delays, and measuring elapsed time.
String Utilities: Helper classes that offer string manipulation functions, like formatting, parsing, and concatenation.
File Utilities: Components that assist in file management, including reading and writing files, managing directories, and handling file paths.
Debugging Utilities: Utility components with functions for logging, debugging, and error reporting to facilitate troubleshooting during development.
Design Considerations
Modularity: Utility components should be designed in a modular fashion, providing well-defined interfaces for different functionalities.
Single Responsibility: Each utility component should have a clear and specific purpose to maintain a clean and organized codebase.
Dependency Management: Utility components should minimize dependencies on other parts of the game to maintain independence and reusability.
Integration with Other Components
Game Logic: Utility components can be used within game logic components to perform specific calculations, operations, or optimizations.
User Interface: UI components might utilize utility components to format text, handle input validation, or manage animations.
Data Access: Utility components might assist in formatting and parsing data before interacting with data access components.
Utility Components help streamline development by providing a library of reusable tools that can simplify common tasks and enhance the overall code quality. By encapsulating frequently used functions and functionalities, utility components contribute to more efficient development and maintenance processes in game projects.
Benefits of Components
In component-based development, software systems are constructed by assembling pre-built, reusable components rather than writing everything from scratch. This approach offers several benefits:
Faster Development: Reusing existing components reduces development time and effort, allowing developers to focus on implementing unique features.
Consistency: Components adhere to established design patterns and best practices, ensuring a consistent and well-structured codebase.
Maintenance: Components can be updated or replaced independently, making it easier to maintain and enhance the system over time.
Scalability: Components can be designed to scale horizontally, allowing the application to handle increased workloads more effectively.
Challenges of Components
Integration: While components promote modular design, integrating various components and ensuring smooth interactions can be complex, especially in large systems.
Compatibility: Components need to be compatible with each other in terms of interfaces, data formats, and dependencies.
Component Bloat: Overusing components or creating overly granular components can lead to excessive complexity and hinder development.
In summary, components are fundamental building blocks of software architecture that promote modularity, reusability, and maintainability. By designing well-defined components and assembling them strategically, developers can create software systems that are flexible, efficient, and responsive to changing requirements.
For more information about Components in Unity Game Engine read here.