In Part 4 of C#, we delve into several fundamental concepts that significantly impact the structure, functionality, and organization of code. Access modifiers play a pivotal role in determining the visibility and accessibility of various code elements, such as classes, methods, and properties.
Understanding and appropriately applying access modifiers like “public,” “private,” “protected,” and “internal” is crucial for designing well-encapsulated and maintainable codebases.
Constants provide a means to declare fixed, unchanging values in a program, often used for mathematical constants or configuration settings, promoting code readability and maintainability by giving meaningful names to important values.
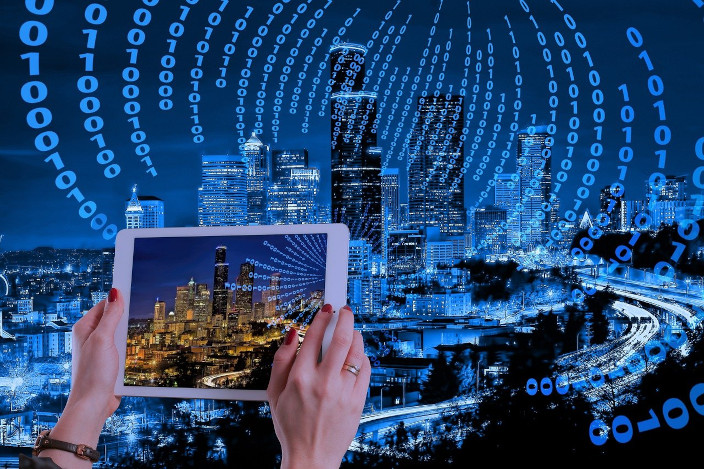
Static elements, including static methods and fields, enable the creation of members associated with a class rather than instances, facilitating shared resources and utility functions across an entire program. Static constructors also come into play for initializing static members.
Ref and out parameters offer mechanisms to pass variables by reference or return multiple values from methods, respectively. Ref parameters allow methods to modify the original variable passed as an argument, while out parameters indicate that a method is expected to assign a value to the parameter, enhancing code flexibility and allowing methods to communicate additional information beyond their return values.
Lastly, generics, marked by type parameters, introduce the capability to write code that can work with different data types while maintaining type safety. This powerful feature fosters code reusability, readability, and efficiency by eliminating the need for repetitive type-specific implementations and promoting versatile solutions.
Access Modifiers in C#
Access modifiers play a fundamental role in controlling the visibility and accessibility of class members. These modifiers include “public,” “private,” “protected,” and “internal.” “Public” allows unrestricted access to a member from any part of the program, making it widely accessible. On the other hand, “private” restricts access to within the same class, ensuring encapsulation and data hiding.
“Protected” allows access within the same class and its subclasses, promoting inheritance and extension of base classes. Lastly, “internal” permits access within the same assembly, promoting encapsulation at a broader level. These access modifiers are crucial for managing the scope and security of code elements, contributing to robust and maintainable C# programs.
Access modifiers can be applied to classes and interfaces to determine whether they are publicly accessible or confined to specific scopes within a program. Methods and properties can also be adorned with access modifiers, with “public” methods providing external access to an object’s behavior while “private” properties restrict access to the internal state, emphasizing encapsulation.
Furthermore, fields, often referred to as instance variables, can be similarly controlled using access modifiers, ensuring that data remains hidden or accessible only where necessary. Constructors, the special methods responsible for object initialization, can have access modifiers, allowing developers to dictate how objects are created and whether external code can invoke them.
Nested types, classes, or enums defined within other classes or structures, are also subject to access modifiers, enabling precise control over their visibility and scope. By leveraging access modifiers across these elements, C# developers can craft well-structured, secure, and maintainable codebases that adhere to fundamental principles like encapsulation and information hiding, which are vital for robust software development.
Constant and Read-only Properties in C#
Constant and read-only properties serve as mechanisms for ensuring the immutability of data within a class. Constants, declared using the “const” keyword, represent values that remain fixed throughout the program’s execution and cannot be modified. These are typically used for values that are known at compile-time and do not change, such as mathematical constants or configuration settings.
On the other hand, read-only properties, declared with the “readonly” keyword, allow developers to create properties whose values can be set only in the constructor of a class or object initializer. This means that while read-only properties can change during object construction, they remain constant throughout the object’s lifetime.
They are often employed when an initial value is required, but it should not be altered afterward. Both constant and read-only properties contribute to code reliability by promoting data immutability and enhancing code maintainability by clearly defining the intent of certain data values within a C# program.
public class ConstantsExample
{
// Constant field
public const int MaxValue = 100; // A constant maximum value that never changes
// Readonly field
public readonly string CreationDate;
public ConstantsExample(string creationDate)
{
// Initialize the readonly field in the constructor
CreationDate = creationDate;
}
public void DisplayValues()
{
Console.WriteLine($"Max Value: {MaxValue}");
Console.WriteLine($"Creation Date: {CreationDate}");
}
}
Static in C#
The “static” keyword allows developers to create members (methods, fields, properties) associated with a type rather than a specific instance of that type. When applied to a method or field, the “static” keyword means that the method or field belongs to the class itself rather than individual objects instantiated from that class.
This characteristic makes static members accessible without needing an instance of the class, making them useful for utility functions, constants, or shared resources across all instances. Static methods are often used for performing operations that are not dependent on the state of an object but are related to the class.
Static fields can be used to create shared data that remains consistent across all instances of the class. Additionally, static constructors (defined using a static constructor block) enable the initialization of static members or other static logic when the class is first accessed or used.
using System;
public class GeometryCalculator
{
// Static method to calculate the area of a rectangle
public static double CalculateRectangleArea(double length, double width)
{
return length * width;
}
// Static method to calculate the area of a circle
public static double CalculateCircleArea(double radius)
{
return Math.PI * Math.Pow(radius, 2);
}
}
class Program
{
static void Main()
{
double rectangleArea = GeometryCalculator.CalculateRectangleArea(5.0, 3.0);
double circleArea = GeometryCalculator.CalculateCircleArea(2.5);
Console.WriteLine($"Area of Rectangle: {rectangleArea}");
Console.WriteLine($"Area of Circle: {circleArea}");
}
}
Method Overloading in C#
Method overloading allows developers to define multiple methods within a class with the same name but different parameter lists. These methods can have different numbers or types of parameters, enabling the same method name to be used for different purposes or with varying input data.
When you invoke an overloaded method, the compiler determines which version of the method to call based on the number and types of arguments provided. Method overloading enhances code readability and flexibility by enabling developers to create methods that perform similar operations on different data types or with different parameter combinations.
using System;
public class Calculator
{
// Method to add two integers
public int Add(int a, int b)
{
return a + b;
}
// Method to add two doubles
public double Add(double a, double b)
{
return a + b;
}
}
class Program
{
static void Main()
{
Calculator calculator = new Calculator();
int resultInt = calculator.Add(5, 3);
double resultDouble = calculator.Add(2.5, 3.7);
Console.WriteLine($"Integer Addition Result: {resultInt}");
Console.WriteLine($"Double Addition Result: {resultDouble}");
Console.ReadLine();
}
}
ref Parameters in C#
Ref parameters provide a mechanism for passing variables to methods by reference rather than by value. When a parameter is marked as “ref” in a method signature, any changes made to that parameter within the method will directly affect the original variable passed as an argument.
This is particularly useful when you need a method to modify the value of a variable passed as an argument and have those changes reflected back in the calling code. Ref parameters are often employed in scenarios where you want to return multiple values from a method or need to manipulate the original data directly.
using System;
public class BankAccount
{
public string AccountHolder;
public double Balance;
public BankAccount(string accountHolder, double balance)
{
AccountHolder = accountHolder;
Balance = balance;
}
}
public class TransactionProcessor
{
public void Deposit(ref BankAccount account, double amount)
{
account.Balance += amount;
}
public void Withdraw(ref BankAccount account, double amount)
{
if (account.Balance >= amount)
{
account.Balance -= amount;
}
else
{
Console.WriteLine("Insufficient balance for withdrawal.");
}
}
}
class Program
{
static void Main()
{
BankAccount myAccount = new BankAccount("John Doe", 1000.0);
TransactionProcessor processor = new TransactionProcessor();
Console.WriteLine($"Initial Balance: {myAccount.Balance}");
double depositAmount = 500.0;
processor.Deposit(ref myAccount, depositAmount);
Console.WriteLine($"Balance after Deposit: {myAccount.Balance}");
double withdrawalAmount = 300.0;
processor.Withdraw(ref myAccount, withdrawalAmount);
Console.WriteLine($"Balance after Withdrawal: {myAccount.Balance}");
Console.ReadLine();
}
}
out Parameters in C#
Out parameters provide a way to return multiple values from a method. When a parameter is marked as “out” in a method signature, it indicates that the method is expected to assign a value to that parameter, which can then be accessed by the calling code after the method call.
They are particularly useful when a method needs to return more than one value or when it needs to modify a parameter passed by reference but doesn’t require the initial value to be provided by the caller. They are often used in scenarios such as error handling, where a method can return both a result and an error code or message.
using System;
public class DivisionCalculator
{
public void Divide(int dividend, int divisor, out int quotient, out int remainder)
{
quotient = dividend / divisor;
remainder = dividend % divisor;
}
}
class Program
{
static void Main()
{
DivisionCalculator calculator = new DivisionCalculator();
int dividend = 20;
int divisor = 3;
int resultQuotient, resultRemainder;
calculator.Divide(dividend, divisor, out resultQuotient, out resultRemainder);
Console.WriteLine($"Dividend: {dividend}");
Console.WriteLine($"Divisor: {divisor}");
Console.WriteLine($"Quotient: {resultQuotient}");
Console.WriteLine($"Remainder: {resultRemainder}");
Console.ReadLine();
}
}
Generics in C#
Generics are a powerful programming feature that allows developers to create classes, interfaces, methods, and delegates that work with a wide range of data types while maintaining type safety. By using generics, you can write code that is more flexible and reusable because it doesn’t depend on a specific data type.
Instead, you define placeholders for data types, often referred to as type parameters, within your code. These type parameters are then replaced with specific data types when the code is used or instantiated.
Generics enable you to design data structures and algorithms that can handle various data types without sacrificing compile-time type checking, leading to more robust and efficient code.
They are extensively used in collections (e.g., List<T>), custom data structures, and methods to create versatile and type-safe solutions in C#. Generics enhance code reusability, maintainability, and performance while providing strong typing and reducing the need for explicit casting.
Generic Classes in C#
Generic classes are a fundamental feature that enables the creation of classes that can work with different data types while maintaining type safety and code reusability. Generic classes are defined with one or more type parameters, allowing developers to create a blueprint for a class that can operate on a wide range of data types.
These type parameters act as placeholders within the class, and they are replaced with specific data types when instances of the class are created. This flexibility makes generic classes particularly useful for creating data structures such as lists, stacks, queues, and custom collections that can accommodate various data types while ensuring type-checking at compile-time.
Generic Methods in C#
Generic methods provide a powerful means to create methods that can work with a wide range of data types while maintaining strong type safety and code flexibility. These methods are defined with one or more type parameters within angle brackets, allowing developers to write code that operates on different data types without sacrificing compile-time type checking.
Generic methods enable you to design functions that can handle various input types and return type-specific results. They are particularly valuable in scenarios where you need to perform the same operation on different data types or when you want to create reusable, type-agnostic algorithms.
Constraint Type Parameters in C#
Constraint type parameters allows developers to specify limitations on the data types that can be used with generic classes or methods. By applying constraints, you can ensure that the type parameters used in generics meet specific criteria, such as implementing particular interfaces, having a default constructor, or inheriting from a certain base class.
These constraints enhance the predictability and reliability of generic code, preventing invalid data types from being used with generic constructs and enabling the compiler to perform compile-time checks to catch type-related errors.
Constraint type parameters are invaluable for creating generic code that is both flexible and safe, allowing you to design generic classes and methods that operate seamlessly with a subset of compatible data types while maintaining type-specific behavior where necessary.
using System;
public class ItemContainer<T> where T : IComparable
{
//where T : IComparable specifies a constraint in C# that restricts the generic type parameter T
//to types that implement the IComparable interface, enabling comparisons between objects of type T.
private T[] items;
private int currentIndex = 0;
public ItemContainer(int capacity)
{
items = new T[capacity];
}
public void AddItem(T item)
{
if (currentIndex < items.Length)
{
items[currentIndex] = item;
currentIndex++;
}
else
{
Console.WriteLine("Item container is full.");
}
}
public T GetItem(int index)
{
if (index >= 0 && index < currentIndex)
{
return items[index];
}
else
{
Console.WriteLine("Invalid index.");
return default(T);
}
}
}
class Program
{
static void Main()
{
// Create an ItemContainer for integers
ItemContainer<int> intContainer = new ItemContainer<int>(5);
intContainer.AddItem(10);
intContainer.AddItem(20);
intContainer.AddItem(30);
// Create an ItemContainer for strings
ItemContainer<string> stringContainer = new ItemContainer<string>(3);
stringContainer.AddItem("Apple");
stringContainer.AddItem("Banana");
// Retrieve items
Console.WriteLine("Int Container Items:");
Console.WriteLine(intContainer.GetItem(0));
Console.WriteLine(intContainer.GetItem(1));
Console.WriteLine(intContainer.GetItem(2));
Console.WriteLine("\nString Container Items:");
Console.WriteLine(stringContainer.GetItem(0));
Console.WriteLine(stringContainer.GetItem(1));
Console.ReadLine();
}
}