In the ever-evolving landscape of game development, creating immersive and lifelike AI characters is a constant challenge. As indie game developers, you might not have access to the vast resources of big studios, but that doesn’t mean your games can’t boast intelligent and engaging AI. This is where Behavior Trees come to your rescue.
Behavior Trees, or BTs for short, are a powerful AI paradigm that simplifies the design and implementation of complex behaviors in your games. Whether you’re working on a strategy, simulation, action-adventure, or any other genre of game, BTs can help you create responsive and dynamic AI characters that enhance the overall player experience.
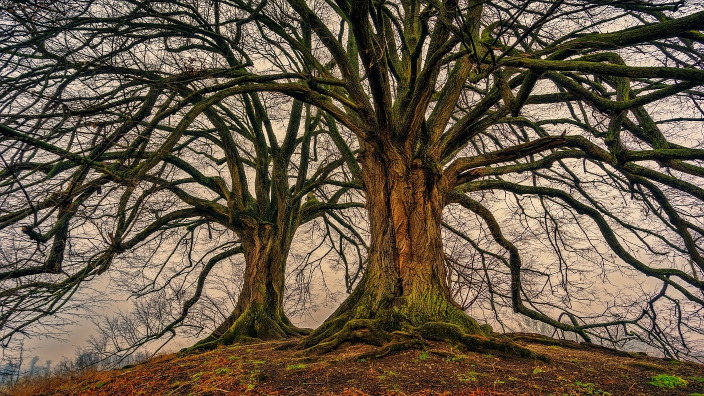
Understanding Behavior Trees
Before we delve into the practical aspects of using Behavior Trees in your games, let’s establish a solid foundation by understanding what BTs are and why they matter in game development.
What Are Behavior Trees?
At their core, Behavior Trees are a hierarchical way of organizing AI behaviors. They are composed of various nodes that define how an AI character should act in response to specific situations. These nodes can range from simple tasks like “move to a target” or “attack an enemy” to more complex decision-making processes.
The key components of a Behavior Tree include:
Nodes
These are the building blocks of a Behavior Tree, each with a specific function. Nodes can be categorized into:
- Selector Nodes: These nodes choose which child node to execute based on certain conditions.
- Sequence Nodes: These nodes execute child nodes sequentially until one fails.
- Action Nodes: These nodes perform specific actions, such as moving, attacking, or interacting.
- Condition Nodes: These nodes check conditions and return success or failure based on the outcome.
Blackboard
The Behavior Tree’s blackboard acts as a shared memory space where data is stored and can be accessed by different nodes. It’s a vital component for communication and decision-making within the tree.
Behavior Trees vs. Other AI Techniques
You might wonder why you should choose Behavior Trees over other AI techniques like finite state machines or decision trees. While all these methods have their merits, Behavior Trees offer some distinct advantages for game developers:
Modularity
Behavior Trees are highly modular. You can break down complex behaviors into smaller, reusable pieces, making them easier to manage and extend.
Hierarchical Structure
Behavior Trees allow you to design behaviors hierarchically. This means you can create high-level goals and sub-goals, offering a clear structure for defining AI behavior.
Dynamic Decision-Making
Behavior Trees excel in dynamic decision-making. The ability to switch between different branches of the tree in response to changing game conditions makes them ideal for responsive and adaptive AI.
Anatomy of a Behavior Tree
Now that we’ve introduced you to the concept of Behavior Trees, let’s roll up our sleeves and dissect these fascinating structures to understand how they function in more detail.
Types of Nodes
Behavior Trees are constructed from several types of nodes, each with its own role in defining AI behavior:
Selector Nodes
These nodes are decision-makers. They evaluate their child nodes one by one until they find one that succeeds. For instance, a selector node might contain child nodes for “attack,” “defend,” and “retreat.” It will choose the first action that the AI character can successfully perform.
Sequence Nodes
Unlike selectors, sequence nodes execute their child nodes in order. They only proceed to the next child if the current one succeeds. Sequences are perfect for defining step-by-step actions.
Action Nodes
Action nodes represent concrete actions that an AI character can perform, such as “move to a target” or “shoot at an enemy.” These nodes contain the actual logic that drives the AI’s actions.
Condition Nodes
Conditions nodes check for certain conditions and return success or failure. They’re essential for decision-making within your Behavior Tree. For example, a condition node might check if the AI character has low health before deciding to retreat.
Node Attributes and Properties
Each node in a Behavior Tree can have attributes and properties that further define its behavior. For instance, an action node might have properties like “weapon type” or “attack range.” These properties can be adjusted and configured to fine-tune the AI’s actions and reactions.
The Role of the Blackboard
The blackboard is a crucial element in a Behavior Tree. It acts as a shared memory space where nodes can read and write data. For example, an action node might write the target’s position to the blackboard, which a subsequent node can then access. The blackboard facilitates communication and data sharing among nodes, making the BT more versatile and dynamic.
In the next section, we’ll dive deeper into designing AI behaviors using Behavior Trees, helping you create intelligent and responsive characters for your games.
Designing AI Behavior with Behavior Trees
Designing AI behavior is a creative process that can greatly impact the player’s experience in your game. Behavior Trees provide an effective framework for crafting AI behaviors that enhance gameplay. In this section, we’ll explore the steps involved in designing AI behavior with Behavior Trees.
Defining the Goals and Objectives
Before diving into the nitty-gritty of creating a Behavior Tree, it’s essential to define clear goals and objectives for your AI character. What role will the AI play in your game? What behaviors should it exhibit? These goals will guide your BT design.
For example, if you’re working on a stealth game, your AI character’s objectives might include:
- Detecting the player when they’re in sight.
- Pursuing the player when detected.
- Losing interest and returning to its initial position after losing sight of the player.
Breaking Down Complex Behaviors
Once you have your goals in mind, it’s time to break down complex behaviors into smaller tasks. This is where BTs shine because they allow you to organize behaviors hierarchically. For example, the “detect player” behavior can be further broken down into sub-behaviors like “scan the environment” and “react to visual cues.”
Hierarchical Organization of Nodes
BTs enable you to create a hierarchical structure for your AI’s decision-making process. High-level goals can be represented by selector nodes, while sub-goals can be organized under sequence nodes. This hierarchical structure makes it easier to manage and maintain your AI’s behavior.
Handling Dynamic Decision-Making
One of the significant advantages of BTs is their ability to handle dynamic decision-making. AI characters can respond to changing game conditions by switching between branches of the tree. For instance, if an AI enemy is chasing a player but encounters an obstacle, it can switch to a “find a path” branch to navigate around the obstacle.
This dynamic nature of BTs ensures that AI characters adapt to the game environment, creating more realistic and engaging experiences for players.
Iterative Development and Playtesting
AI behavior design is rarely a one-and-done process. It involves iterations and playtesting to fine-tune the BT. Observing how the AI behaves in different game scenarios and adjusting based on player feedback is crucial for creating compelling AI.
Remember that Behavior Trees offer flexibility. You can make changes to individual nodes or add new ones to improve AI behavior. This iterative approach allows you to refine your AI over time, ensuring it aligns with your game’s objectives.
In the next section, we’ll move from theory to practice, discussing the implementation of Behavior Trees in your game. We’ll explore the tools and techniques you can use to bring your AI behaviors to life.
Implementing Behavior Trees
Now that you understand the theory behind Behavior Trees and how to design AI behavior, it’s time to roll up your sleeves and bring those AI characters to life in your game. In this section, we’ll explore the practical aspects of implementing BTs, tailored for indie game developers.
Choosing a Game Engine
The first step in implementing BTs is to select a game engine that supports them. Fortunately, many popular game engines offer built-in or third-party BT libraries. Some engines like Unity and Unreal Engine have their own visual BT editors, making it easier to create and visualize your AI’s behavior.
Coding BTs from Scratch vs. Using Existing Libraries
As an indie developer, you might be tempted to code everything from scratch to maintain full control over your project. While this approach can be rewarding, it can also be time-consuming and complex, especially when dealing with AI. Consider using existing BT libraries or visual editors to streamline the process and reduce development time.
Integrating BTs into Your Game
Once you’ve chosen your game engine and BT tools, it’s time to integrate BTs into your game. This typically involves:
- Importing the BT library or plugin into your project.
- Defining the structure of your Behavior Trees using the provided tools or scripting.
- Linking the BTs to your AI characters.
- Implementing the execution of BTs in your game’s logic.
Debugging and Testing Your BTs
Debugging AI behavior can be challenging, but it’s a critical step in the development process. Most BT tools provide debugging features that allow you to visualize the flow of your BT in real-time. Use these features to identify issues and refine your AI’s behavior.
Additionally, playtesting is essential. Observe how your AI characters interact with the game environment and players. Adjust based on the feedback and iterate until you achieve the desired balance between challenge and fun.
Performance Considerations
Efficient AI is crucial for smooth gameplay. Keep an eye on the performance of your BTs, especially if your game has many AI characters. Here are some performance considerations:
Node Evaluation
Minimize expensive computations in your BT nodes. Evaluate conditions and actions efficiently to reduce CPU usage.
Parallel Processing
Some BT libraries support parallel processing, allowing multiple AI characters to update their BTs simultaneously. This can help distribute the workload and improve performance.
Optimizations
Consider implementing optimizations like caching expensive calculations or using data-driven approaches to reduce the computational load on your AI.
By following these implementation guidelines, you can seamlessly integrate Behavior Trees into your game, creating AI characters that react intelligently to the game world and player actions.
Creating a Simple Behavior Tree
In this example, we will create a Behavior Tree for an AI enemy in a shooting game. The enemy’s behavior is as follows: it should first check if the target (player) is within range; if not, it moves closer to the target.
Once it’s within range, it initiates an attack. During the attack, the enemy may take damage, so it should check if it’s in danger (health is low). If this is the case, it should prioritize retreating to safety. To keep things simple, instead of using a blackboard, we will provide nodes access to the enemy’s information.
Please note that this is a basic example. In this example, the Behavior Tree contains hardcoded nodes, which means that to add or remove nodes, we need to modify its code. In practice, for more flexibility and maintainability, we would design the Behavior Tree to accept nodes externally, allowing us to configure and modify it through a form of external configuration or data-driven approach.
Behavior Tree C# Example
using System;
namespace BehaviorTree
{
class Program
{
static void Main(string[] args)
{
//The player
Player player = new Player(10, 10);
//The enemy
int range = 3;
int health = 5;
Enemy enemy = new Enemy(0, 0, range, health, player);
//Create a Behavior Tree for the enemy
BehaviorTree behaviorTree = new BehaviorTree(enemy);
Console.WriteLine("");
//Game loop
for (int i = 0; i < 15; i++)
{
Console.WriteLine("Game loop step: " + i.ToString());
behaviorTree.Execute();
Console.WriteLine("");
}
Console.ReadLine();
}
}
}
//A simple representation of the player.
using System;
namespace BehaviorTree
{
public class Player
{
public int X;
public int Y;
public Player(int x, int y)
{
X = x;
Y = y;
Console.WriteLine("Player starts at (" + X.ToString() + "," + Y.ToString() + ")");
}
public bool GetDistance(int x, int y, int range)
{
if (Math.Abs(X - x) <= range || Math.Abs(Y - y) <= range)
return true;
else
return false;
}
}
}
//A simple representation of the enemy.
using System;
namespace BehaviorTree
{
public class Enemy
{
public int X;
public int Y;
public int Range;
public int Health;
private bool playerInRange;
private Player thePlayer;
public Enemy(int x, int y, int range, int health, Player player)
{
X = x;
Y = y;
Range = range;
Health = health;
thePlayer = player;
Console.WriteLine("Enemy starts at (" + X.ToString() + "," + Y.ToString() + ") with weapon range: " + range.ToString());
}
public void Move(int x, int y)
{
X = x;
Y = y;
playerInRange = thePlayer.GetDistance(X, Y, Range);
Console.WriteLine("Enemy Moved to (" + X.ToString() + "," + Y.ToString() + ")");
}
public void GetDamage()
{
Health-=2;
}
public bool IsPlayerInRange()
{
return playerInRange;
}
public bool IsInDanger()
{
return (Health < 2) ? true : false;
}
}
}
//A Basic BehaviorTree class
namespace BehaviorTree
{
public class BehaviorTree
{
//Root node of the Behavior Tree
private Node rootNode;
// Constructor to initialize the Behavior Tree
public BehaviorTree(Enemy enemy)
{
// Create the nodes
Node chasePlayerNode = new ChasePlayerNode(enemy);
Node isPlayerInRangeNode = new IsPlayerInRangeNode(enemy);
Node attackNode = new AttackNode(enemy);
Node isEnemyInDangerNode = new IsEnemyInDangerNode(enemy);
Node retreatNode = new RetreatNode(enemy);
// Create a Selector Node as the root
rootNode = new SelectorNode();
// Add child nodes to the Selector Node
rootNode.AddChild(chasePlayerNode);
rootNode.AddChild(isPlayerInRangeNode);
rootNode.AddChild(attackNode);
rootNode.AddChild(isEnemyInDangerNode);
rootNode.AddChild(retreatNode);
}
// Execute the Behavior Tree
public void Execute()
{
rootNode.Evaluate();
}
}
}
//Selector Node. These nodes are decision-makers.
//They evaluate their child nodes one by one until they find one that succeeds.
using System;
using System.Collections.Generic;
namespace BehaviorTree
{
public class SelectorNode : Node
{
private List<Node> children = new List<Node>();
public SelectorNode() : base("SELECTOR"){ }
public override void AddChild(Node child)
{
children.Add(child);
}
public override void Evaluate()
{
foreach (Node child in children)
{
Console.WriteLine(child.Name + " evaluated");
child.Evaluate();
// If the child node succeeds, stop iterating and return success
if (child.Status == NodeStatus.Success)
return;
}
}
}
}
//Action node to chase the player.
using System;
namespace BehaviorTree
{
public class ChasePlayerNode : Node
{
private Enemy enemy;
public ChasePlayerNode(Enemy enemy) : base("CHASE PLAYER")
{
this.enemy = enemy;
}
public override void AddChild(Node child)
{
throw new NotImplementedException();
}
public override void Evaluate()
{
if (enemy.IsInDanger())
Status = NodeStatus.Failure;
else
{
if (enemy.IsPlayerInRange())
{
Console.WriteLine("Player already in range. No movement.");
Status = NodeStatus.Failure;
}
else
{
Console.WriteLine("Chasing the player...");
enemy.Move(enemy.X + 1, enemy.Y + 1);
Status = NodeStatus.Success;
}
}
}
}
}
//Condition node to check if the player is within attack range
using System;
namespace BehaviorTree
{
public class IsPlayerInRangeNode : Node
{
private Enemy enemy;
public IsPlayerInRangeNode(Enemy enemy) : base("IS_PLAYER_IN_RANGE")
{
this.enemy = enemy;
}
public override void AddChild(Node child)
{
throw new NotImplementedException();
}
public override void Evaluate()
{
if (enemy.IsInDanger())
{
//Console.WriteLine("Player is in danger.");
Status = NodeStatus.Failure;
}
else
{
if (enemy.IsPlayerInRange())
{
Console.WriteLine("Player in range...");
Status = NodeStatus.Failure;
}
else
{
Console.WriteLine("Chasing the player...");
Status = NodeStatus.Success;
}
}
}
}
}
//Action node to attack the player.
using System;
namespace BehaviorTree
{
public class AttackNode : Node
{
private Enemy enemy;
public AttackNode(Enemy enemy) : base("ATTACK PLAYER")
{
this.enemy = enemy;
}
public override void AddChild(Node child)
{
throw new NotImplementedException();
}
public override void Evaluate()
{
if (!enemy.IsInDanger())
{
Console.WriteLine("Enemy is strong. Health: " + enemy.Health.ToString());
Console.WriteLine("Attacking the player...");
enemy.GetDamage();
Status = NodeStatus.Success;
}
else
{
Status = NodeStatus.Failure;
}
}
}
}
//Condition node to check if the enemy is in danger (low health).
using System;
namespace BehaviorTree
{
public class IsEnemyInDangerNode : Node
{
private Enemy enemy;
public IsEnemyInDangerNode(Enemy enemy) : base("IS_ENEMY_IN_DANGER")
{
this.enemy = enemy;
}
public override void AddChild(Node child)
{
throw new NotImplementedException();
}
public override void Evaluate()
{
if (enemy.IsInDanger())
{
Console.WriteLine("Enemy is in danger. Health: " + enemy.Health.ToString());
Status = NodeStatus.Failure;
}
else
{
Console.WriteLine("Enemy is strong. Health: " + enemy.Health.ToString());
Status = NodeStatus.Success;
}
}
}
}
//Action node to retreat.
using System;
namespace BehaviorTree
{
public class RetreatNode : Node
{
private Enemy enemy;
public RetreatNode(Enemy enemy) : base("RETREAT")
{
this.enemy = enemy;
}
public override void AddChild(Node child)
{
throw new NotImplementedException();
}
public override void Evaluate()
{
// Logic to retreat
Console.WriteLine("Enemy retreating...");
enemy.Move(enemy.X - 1, enemy.Y - 1);
Status = NodeStatus.Success;
}
}
}
//Base Node class
namespace BehaviorTree
{
public abstract class Node
{
public string Name;
public NodeStatus Status;
public Node(string name)
{
Name = name;
}
public abstract void AddChild(Node child);
public abstract void Evaluate();
}
}
//Enum to represent the status of a node (Success, Failure, or Running)
namespace BehaviorTree
{
public enum NodeStatus
{
Success,
Failure,
Running
}
}
Best Practices for Using Behavior Trees
As you embark on your journey of utilizing Behavior Trees in your indie game development, it’s crucial to follow some best practices to make the most of this powerful AI technique. Here, we’ll share some tried-and-true tips to ensure your BTs are not only effective but also maintainable and adaptable.
Keeping the Behavior Trees Readable and Maintainable
Modular Design
Organize your BTs in a modular fashion. Use sub-trees for common behaviors that multiple AI characters share. This keeps your Behavior Trees more manageable and promotes code reusability.
Comment and Document
Comment your BT nodes and provide documentation for complex behavior branches. This helps not only you but also your team members understand and maintain the AI code.
Naming Conventions
Use clear and descriptive names for nodes and variables in your Behavior Trees. This makes it easier to understand their purpose immediately.
Balancing Complexity and Simplicity
Avoid Overcomplication
While BTs are versatile, resist the urge to create overly complex trees. Simplicity often leads to more predictable and maintainable AI.
Clear Hierarchy
Ensure a clear hierarchy in your BTs. High-level goals should be easy to distinguish from lower-level actions and conditions.
Avoid Deep Nesting
Limit deep nesting of nodes within your BT. Deep trees can become hard to follow and debug.
Fine-Tuning for Optimal Gameplay
Iterate and Playtest
Behavior Tree design is an iterative process. Continuously playtest your AI to find areas for improvement and adjust accordingly.
Player Experience
Keep the player’s experience in mind. AI behaviors should enhance the gameplay, not frustrate players. Balance challenge with fairness.
Difficulty Levels
Consider implementing different BTs or adjusting node properties for varying difficulty levels to cater to a broader audience.
Debugging and Monitoring
Debugging Tools
Leverage built-in debugging tools provided by your game engine or BT library. Visualize the execution of your BTs in real-time to identify issues.
Logging
Implement logging in your BT nodes to record AI decisions and actions. This can be invaluable for post-mortem analysis and debugging.
Remember that Behavior Trees are a tool in your game development toolbox and mastering them takes time and practice. By following these best practices, you’ll be well on your way to creating AI behaviors that not only meet your game’s objectives but also captivate and engage your players.
Challenges and Common Pitfalls
As you venture further into the world of Behavior Trees (BTs) in game development, it’s essential to be aware of the challenges and common pitfalls that may arise during the implementation and fine-tuning of AI behaviors. Understanding these challenges can help you navigate them effectively and create more polished and engaging games.
Avoiding Common Mistakes in BT Design
Overcomplicating Behavior Trees
One of the most common mistakes is creating overly complex BTs. While they offer flexibility, it’s important to strike a balance between simplicity and sophistication. An excessively intricate tree can become challenging to maintain and debug.
Lack of Modularity
Failing to structure your Behavior Trees in a modular way can lead to difficulties in reusing behaviors across different AI characters. Modular design promotes code reusability and maintainability.
Unpredictable Behaviors
Inconsistent AI behavior can frustrate players. Ensure that your BTs produce reliable and predictable results to provide a fair and enjoyable gaming experience.
Handling Complex State Transitions
State Transition Confusion
When dealing with complex state transitions in your BTs, such as moving from “idle” to “attack” or “patrol” to “chase,” make sure transitions are well-defined and follow logical patterns. Ambiguity in transitions can result in erratic AI behavior.
Memory and Context Management
Managing information on the blackboard is crucial. Avoid cluttering the blackboard with unnecessary data and ensure that relevant information is stored and updated appropriately to support decision-making.
Fine-Tuning
Fine-tuning AI behaviors can be a delicate process. Striking the right balance between difficulty and fairness requires thorough playtesting and iteration.
Managing AI Behavior Consistency
Consistency Across Platforms
If you plan to release your game on multiple platforms, ensure that AI behavior remains consistent across different hardware and operating systems. Compatibility issues can arise if your BTs rely on platform-specific features.
Multiplayer Considerations
If your game features multiplayer modes, you must account for synchronization and replication of AI behaviors across multiple clients. Inconsistent AI behavior can lead to unfair advantages or confusion among players.
Player-Character Interaction
AI characters should interact convincingly with player-controlled characters. Make sure that AI behaviors respond appropriately to player actions and provide a cohesive gameplay experience.
Navigating these challenges and avoiding common pitfalls is an ongoing process in AI behavior design. Regular playtesting, open communication with your development team, and a willingness to iterate and refine your BTs are essential for creating AI that enhances your game rather than detracting from it.
In the final sections of this guide, we’ll explore the future trends and advanced topics related to Behavior Trees in game development, helping you stay at the forefront of AI innovation in the indie game industry.
Future Trends and Advanced Topics
As an indie game developer, staying ahead of the curve is crucial to creating innovative and captivating gaming experiences. In this section, we’ll explore some future trends and advanced topics related to Behavior Trees (BTs) in game development, providing you with insights into the evolving landscape of AI in gaming.
Evolution of BTs in Game Development
Behavior Trees have come a long way and continue to evolve. Here are some trends to watch for:
Machine Learning Integration
The integration of machine learning techniques with BTs is on the horizon. This can lead to AI characters that learn and adapt to player strategies over time, creating more challenging and immersive gameplay experiences.
Procedural Content Generation
BTs can be used to generate procedural content, such as level layouts, enemy placement, and mission structures. This allows for dynamically generated game worlds that keep players engaged.
Combining BTs with Other AI Techniques
While BTs are powerful on their own, combining them with other AI techniques can open new possibilities:
Finite State Machines (FSMs)
FSMs can be used in conjunction with BTs to handle lower-level, immediate decision-making while using BTs for higher-level, strategic behaviors. This hybrid approach can lead to more responsive AI characters.
Reinforcement Learning
Integrating reinforcement learning algorithms with BTs can result in AI characters that learn and adapt through trial and error. This can be particularly useful for creating adaptable opponents in strategy and simulation games.
Machine Learning and BTs
Machine learning techniques, such as neural networks and deep reinforcement learning, are pushing the boundaries of AI in games:
Neural Networks for Decision-Making
Neural networks can be used within BTs to make complex decisions, such as predicting player behavior and adjusting AI responses accordingly.
Deep Reinforcement Learning (DRL)
DRL can be employed to train AI agents to perform complex tasks, making them more intelligent and adaptable. This can be applied to non-player characters (NPCs) and in-game entities.
Adaptive AI with Behavior Trees
Adaptive AI is a hot topic in game development. Here’s how BTs can play a role:
Player Profiling
BTs can be used to profile player behavior and adapt AI opponents accordingly. This ensures that the game remains challenging and enjoyable for players of different skill levels.
Dynamic Difficulty Adjustment
BTs can dynamically adjust the difficulty level based on the player’s performance, creating a tailored experience for each player.
Staying informed about these advanced topics and trends can help you leverage Behavior Trees and other AI techniques effectively in your indie game development. As technology continues to advance, indie developers can push the boundaries of what’s possible in gaming, creating unique and memorable experiences for players.
Conclusion
Congratulations, indie game developer, for embarking on this journey into the world of Behavior Trees in game development. We hope this guide has equipped you with valuable insights and practical knowledge to harness the power of BTs and create more engaging and intelligent AI characters for your games.
Here are some key takeaways to remember:
Behavior Trees Simplify AI Design: BTs provide a structured and hierarchical approach to designing AI behavior, making it more manageable and adaptable.
Design with Player Experience in Mind: AI behavior should enhance the player’s experience, offering challenge and engagement without frustration.
Iterate and Playtest: AI behavior design is an iterative process. Regular playtesting and feedback are essential for fine-tuning your BTs.
Stay Informed about Trends: The world of AI in gaming is constantly evolving. Keep an eye on emerging trends and technologies to stay at the forefront of game development.
Combine Techniques for Innovation: Consider combining BTs with other AI techniques and machine learning to create more dynamic and adaptive AI characters.
Adaptive AI Enhances Engagement: Adaptive AI, enabled by BTs, can provide tailored experiences for players of different skill levels, enhancing player retention and enjoyment.
As an indie game developer, you have the creative freedom to explore new possibilities and push the boundaries of AI in your games. Behavior Trees offer a versatile and powerful toolset to help you achieve your vision and captivate your audience.
So, go forth and create immersive gaming experiences that leave a lasting impact. Embrace the potential of Behavior Trees, and may your indie games be filled with engaging AI characters that bring your virtual worlds to life. We look forward to playing your creations in the exciting future of indie game development!
If you enjoyed this read, you might also find this article interesting.