C# Part 1 introduces the fundamental concepts and building blocks that underpin C# development. The guide begins with an introduction to C#, highlighting its history, significance, and real-world applications. It sets the stage by explaining how C# fits into the broader landscape of programming languages and why it is a valuable skill for aspiring developers.
Moving forward, the course delves into the core elements of C# programming, starting with variables. You gain a deep understanding of how to declare, define, and manipulate variables to store data effectively. Variables are the vessels that hold essential information in a program, and mastering their use is vital for any coding endeavor.
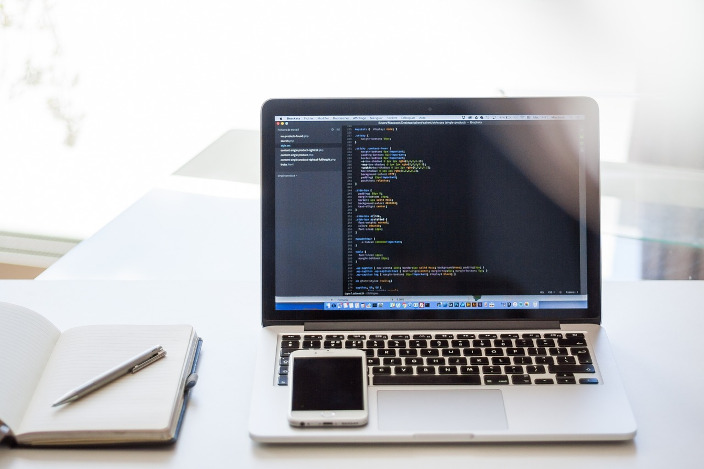
Furthermore, the course explores methods, which are the engines behind C# programs. You learn how to create, call, and manage methods to encapsulate and execute code efficiently. By the end of Part 1, learners are equipped with a solid foundation in C#, setting the stage for more advanced topics and in-depth exploration of the language’s capabilities in subsequent parts of the course.
Introduction
C# (pronounced “C-sharp”) is a versatile, modern, and object-oriented programming language developed by Microsoft. It was introduced in the early 2000s and has since become a prominent language in the software development industry.
C# is renowned for its simplicity, readability, and robustness, making it an excellent choice for a wide range of applications, from desktop software and web development to game development and mobile app creation.
One of C#’s key features is its close integration with the Microsoft .NET framework, which provides a rich library of pre-built functions and tools that simplify and expedite development. This integration enables developers to build powerful and efficient applications with ease.
C# supports various programming paradigms, including imperative, declarative, and object-oriented programming, offering developers flexibility in how they approach problem-solving. Its strong type system and static typing ensure early detection of errors, enhancing code reliability and maintainability.
With C#, developers can leverage the extensive Visual Studio IDE, also developed by Microsoft, to streamline the development process, debug code efficiently, and access a wealth of resources for learning and support.
.NET Framework
The .NET Framework is a comprehensive and widely used software development platform created by Microsoft. It provides developers with a versatile and robust environment for building and deploying various types of applications, from desktop software and web applications to cloud-based solutions.
At its core, the .NET Framework offers a common runtime environment that supports multiple programming languages, including C#, Visual Basic .NET, and F#. This flexibility allows developers to choose the language that best suits their project’s requirements while still leveraging the rich features of the .NET ecosystem.
One of the standout features of the .NET Framework is its extensive class library, known as the Base Class Library (BCL), which includes a vast array of pre-built functions and components. These libraries simplify many common programming tasks, such as database connectivity, file I/O, and user interface development.
Additionally, the .NET Framework includes the Common Language Runtime (CLR), which manages memory allocation, handles exception handling, and ensures type safety, enhancing application stability and security. As a result, developers can focus more on writing application-specific code and less on low-level technicalities, leading to increased productivity and faster development cycles.
The .NET Framework continues to evolve, with Microsoft introducing .NET Core and eventually .NET 5 and beyond, providing cross-platform capabilities, improved performance, and a broader range of development opportunities, ensuring its relevance in modern software development.
Diverse Applications of C#: From Desktop to Web and Beyond
C# is a versatile programming language with a wide range of applications across various domains. It is commonly used in software development to build desktop applications, web applications, and mobile apps.
In the realm of desktop applications, C# is often employed to create Windows-based software using technologies like Windows Presentation Foundation (WPF) for building rich, interactive user interfaces. This makes it an ideal choice for developing productivity tools, business applications, and multimedia software that require a native Windows experience.
C# is also a popular language for web development, particularly when used in conjunction with ASP.NET, a web framework developed by Microsoft. With ASP.NET, developers can build dynamic and data-driven web applications, from content management systems and e-commerce platforms to web services and APIs.
It offers strong support for server-side logic, making it a reliable choice for handling complex business logic and data processing on the server, and it integrates seamlessly with databases, ensuring efficient data management.
Moreover, C# has gained traction in game development through the Unity game engine, making it a staple in the gaming industry. This allows developers to create cross-platform games for consoles, mobile devices, and PCs with the benefits of C#’s syntax and extensive libraries.
In the mobile app development space, C# shines through Xamarin, a framework that allows developers to create cross-platform mobile apps for iOS and Android using C#. This enables code sharing between platforms, reducing development time and effort while maintaining a high level of performance.
In summary, C# finds its utility across a wide spectrum of development scenarios, making it a valuable tool for programmers looking to tackle diverse projects in today’s software landscape.
Harnessing the Power of C# in Unity Game Development
C# in Unity game development is a potent combination that has revolutionized the gaming industry. Unity, a versatile and widely-used game engine, provides a robust framework for creating games for various platforms, from mobile devices to consoles and PCs.
At the heart of Unity’s scripting capabilities lies C#, which serves as the primary programming language for developing game logic, creating interactive gameplay elements, and managing in-game behavior.
One of the key advantages of using C# in Unity is its accessibility and familiarity. C# is a widely-adopted language, making it accessible to a broad community of developers. Its syntax is relatively straightforward, which means that both beginners and experienced programmers can quickly get up to speed with Unity development.
Moreover, Unity’s extensive documentation and a thriving online community offer abundant resources for learning and troubleshooting, further enhancing the appeal of using C# in Unity.
C# also brings performance and versatility to Unity game development. C# scripts in Unity are compiled to native code, which results in efficient and high-performance gameplay experiences. Developers can leverage C#’s object-oriented features to create modular and reusable code, making game development more manageable and less error-prone.
Additionally, C# allows for seamless integration with external libraries and APIs, enabling developers to enhance their games with third-party tools and services. Overall, C# in Unity game development empowers creators to build immersive and engaging gaming experiences across a multitude of platforms.
C# Integration in Alternative Game Engines: Expanding Possibilities in Game Development
C# Integration in alternative game engines represents a significant shift in the landscape of game development, opening new avenues for developers to create interactive and immersive gaming experiences.
While Unity remains one of the primary game engines that heavily relies on C#, several alternative game engines have also recognized the language’s versatility and adopted it as a primary or secondary scripting language. This expansion of C# into other game engines not only broadens the horizons for developers but also streamlines the development process and enhances cross-platform capabilities.
One notable example of C# integration can be found in the Godot game engine. Godot offers native support for C# alongside its scripting language, GDScript. This dual-language support provides developers with flexibility, allowing them to choose the language that aligns best with their project’s requirements and their own expertise.
The inclusion of C# in Godot brings the benefits of a mature, well-documented language into a versatile game engine, making it easier for developers to create games for various platforms while leveraging the extensive libraries and resources available for C#.
Unreal Engine, another prominent game engine, has also incorporated C# through third-party plugins and integrations. While Unreal Engine primarily uses C++ for coding, the addition of C# through plugins like UnrealCLR provides developers with an alternative option for scripting. This integration simplifies the development process for those already proficient in C# and allows them to harness the Unreal Engine’s powerful graphics capabilities and robust physics systems.
Consequently, C# integration in these alternative game engines is contributing to the democratization of game development by providing accessible and familiar tools to a broader range of developers, ultimately leading to more diverse and innovative games.
The Art of Code Communication: Comments in C#
Comments allow developers to embed explanatory notes within their source code, providing insights into the purpose, functionality, or nuances of specific code blocks or individual lines. These comments are entirely ignored by the compiler, making them a tool exclusively for human readers.
By adding comments, developers can document their code, making it easier for themselves and others to understand and modify it in the future. Well-commented code is not only a valuable aid for troubleshooting and debugging but also a cornerstone of effective collaboration among developers, ensuring that the intent and logic behind the code remain clear throughout the software development lifecycle.
// This is a single-line comment
/* This is a multi-line comment.
It can span across multiple lines,
providing detailed information about the code. */
Variables in C#
In programming, a variable is a fundamental concept used to store and manage data. Think of it as a labeled container that holds information, such as numbers, text, or objects, which can be used and manipulated throughout a program.
Defining Variable in C#
In C#, you can define a variable by specifying its data type followed by a name, allowing you to reference and modify the stored data. For example, consider this C# snippet:
int age; // Here, 'int' signifies the data type (integer), and 'age' is the variable name.
In this case, we’ve defined an integer variable called ‘age.’ This variable can now store whole numbers, and you can assign values to it like this:
age = 30; // Assigning the value 30 to the 'age' variable.
Variables are indispensable in programming, serving as placeholders for data that can change or be manipulated as a program executes, allowing developers to work with dynamic information effectively.
Types of Variables in C#
Variables in programming can take on various data types, each defining the kind of data they can store. In C#, these data types include integers, floating-point numbers, characters, and more.
These variable types allow programmers to work with diverse types of data, providing flexibility and precision in managing information within a program. Choosing the appropriate data type is crucial for optimizing memory usage and ensuring data accuracy in any coding endeavor. Following are the built-in primitive data types in C#.
- sbyte: Signed 8-bit integer.
- byte: Unsigned 8-bit integer.
- short: Signed 16-bit integer.
- ushort: Unsigned 16-bit integer.
- int: Signed 32-bit integer.
- uint: Unsigned 32-bit integer.
- long: Signed 64-bit integer.
- ulong: Unsigned 64-bit integer.
- float: Single-precision floating-point number.
- double: Double-precision floating-point number.
- decimal: Decimal number with higher precision.
- char: Single 16-bit Unicode character.
- string: A sequence of characters (Unicode text).
- bool: Boolean value (true or false).
- object: The base type for all types in C#.
- dynamic: A type that bypasses compile-time type checking (late binding).
- var: Allows the compiler to infer the data type from the assigned value.
- Additionally, C# supports user-defined types, structures, enums, and reference types like classes.
Access Modifiers in C#
Variable access modifiers in C# are essential for controlling the visibility and accessibility of variables within a program. They determine which parts of code can read or modify a particular variable. C# provides several access modifiers, including public, private, protected, internal, and protected internal, each serving a distinct purpose.
The public access modifier allows variables to be accessed from anywhere in the program, making them widely visible. In contrast, private restricts access to only within the defining class or struct, ensuring encapsulation and data integrity. Protected enables access from derived classes, while internal permits access within the same assembly, enhancing code organization and separation of concerns. Protected internal combines the functionalities of both protected and internal, allowing access within the same assembly and from derived classes.
By judiciously choosing the appropriate access modifier, developers can strike a balance between security, encapsulation, and code maintainability, ensuring that variables are accessed and manipulated in a controlled and purposeful manner within a C# program.
// This variable is publicly accessible from anywhere.
public int publicVar = 42;
// This variable is only accessible within this class.
private string privateVar = "I am a secret.";
// This variable is accessible within this class and derived classes.
protected double protectedVar = 3.14;
// This variable is accessible within the same assembly.
internal bool internalVar = true;
// This variable is accessible within the same assembly and derived classes.
protected internal char protectedInternalVar = 'A';
Variable Declaration in C#
Variable declaration in C# is the process of specifying a variable’s name and data type, informing the compiler about its existence and how to allocate memory for it. For instance, int age; declares an integer variable named age. In this example, the data type is int, representing whole numbers, and the variable name is age.
After declaration, you can assign values to the variable, like age = 30;, which sets the age variable to the value 30. Variable declarations play a vital role in code clarity and organization, ensuring that developers understand the type of data a variable holds and its purpose within the program, which fosters better code readability and maintainability.
Variable Definition versus Variable Declaration
The terms “variable definition” and “variable declaration” are often used interchangeably, but they have distinct meanings in the context of programming.
Variable declaration refers to the act of specifying a variable’s name and, optionally, its data type, informing the compiler of its existence and type, without necessarily assigning it a value. For example, in C#, int age; is a variable declaration where age is declared as an integer variable.
Variable definition, on the other hand, goes a step further. It not only declares the variable but also assigns it an initial value. For instance, int age = 30; is both a declaration and a definition because it specifies the variable’s name, data type, and initializes it with the value 30.
Variable Assignment in C#
Variable assignment is the dynamic process that allows variables to store, update, and manipulate data during the program’s execution. In languages like C#, you can use the assignment operator (=) to assign a value to a variable.
For example, int age = 30; assigns the value 30 to the integer variable age. Variable assignment is not limited to initial values; you can change a variable’s value as needed throughout your code.
This flexibility is vital for developing dynamic and interactive software, as it enables the program to respond to changing conditions and user input. Understanding how and when to assign values to variables is essential for creating functional and responsive programs.
Type conversions in C#
Type conversions in C# play a pivotal role in ensuring the compatibility and flexibility of data within a program. These conversions facilitate the transformation of data from one data type to another, allowing for seamless interaction between different parts of a program.
Implicit Conversions
In C#, type conversions can be categorized into two main types: implicit and explicit. Implicit conversions occur when the compiler can automatically convert one data type to another without any loss of data or information, such as converting an integer to a double. When dealing with C# implicit conversions, it’s crucial to consider a few key aspects to ensure smooth and safe data manipulation.
First and foremost, one should be aware of potential data loss. Implicit conversions typically involve converting a smaller data type to a larger one, and while the compiler handles this automatically, it can lead to data truncation if the value exceeds the range of the destination type. Therefore, programmers should exercise caution when relying on implicit conversions to avoid unintended loss of precision or errors.
Additionally, understanding the hierarchy of data types is vital. Implicit conversions follow a specific hierarchy determined by the C# type system. Being aware of this hierarchy helps in predicting how data will be converted and ensuring that conversions are logical and sensible within the context of your code.
Lastly, it’s essential to maintain code readability and avoid ambiguity. While implicit conversions can be convenient, excessive use or chaining of implicit conversions may lead to code that is difficult to understand. In such cases, explicitly specifying conversions (casting) or providing comments can enhance code clarity and make it more maintainable.
The Type System Hierarchy of C# for Implicit Conversions
The type system hierarchy of C# for implicit conversions follows a specific order known as widening or promotion. Implicit conversions can occur when moving from a lower-ranked data type to a higher-ranked one, ensuring that no data is lost in the process.
This hierarchy begins with the simplest data types, such as integers and floating-point numbers, and gradually moves up to more complex types, such as structs and classes. For example, implicit conversions can occur from an integer to a float, from a derived class to its base class, or from an enum to its underlying integral type.
However, implicit conversions in the opposite direction—going from a higher-ranked type to a lower-ranked one—require explicit casting to avoid potential data loss. Understanding this hierarchy is crucial for writing code that flows smoothly and avoids unexpected data-related issues.
Implicit Conversion Pitfalls
Data Loss: One of the most significant pitfalls is data loss during implicit conversions. For instance, if you try to convert a large floating-point number to an integer using an implicit conversion, you will lose the fractional part of the number, potentially leading to inaccurate results.
double largeNumber = 1234.5678;
int integerResult = largeNumber; // Implicit conversion truncates the decimal part, resulting in 1234.
Loss of Precision: Implicit conversions between data types with different precision can result in unexpected behavior. For instance, when converting a decimal to a float or double, you may encounter precision loss, affecting calculations.
decimal highPrecisionValue = 1234.567890123456789012345m;
float floatValue = highPrecisionValue; // Implicit conversion results in precision loss.
Overflow: Implicit conversions can lead to overflow errors when converting from a larger data type to a smaller one. If the value being converted exceeds the range of the destination type, it can cause unexpected results or exceptions.
long largeValue = long.MaxValue;
int intValue = largeValue; // Implicit conversion may result in overflow.
Unintended Type Promotion: Implicit conversions can promote a data type to a larger, more complex type unintentionally. This can lead to unnecessary memory consumption and performance issues.
byte smallValue = 42;
int promotedValue = smallValue; // Implicit conversion promotes byte to int.
Loss of Sign Information: Converting unsigned data types to signed data types through implicit conversion can lead to a loss of sign information, potentially causing unexpected behavior.
uint unsignedValue = 4294967295;
int signedValue = unsignedValue; // Implicit conversion may result in loss of
Explicit Conversions – Casting
On the other hand, explicit conversions, also known as casting, require the programmer to specify the conversion explicitly, which may involve potential data loss or exceptions if not done carefully.
When working with explicit conversions in C#, careful consideration is essential to maintain data integrity and prevent runtime errors. Explicit conversions, also known as casting, require the programmer to explicitly specify the conversion from one data type to another.
It’s important to keep in mind that these conversions can lead to data loss or unexpected behavior if not handled correctly. As a result, it’s crucial to perform error checking before casting to ensure that the operation is safe and will not result in data truncation, overflow, or other undesirable consequences.
Additionally, explicit conversions should be used sparingly, as they can make code less readable and should only be employed when there is a clear and necessary reason to convert data from one type to another.
Explicit Conversion Pitfalls
Invalid Cast Exception: Attempting to cast incompatible data types can lead to an InvalidCastException at runtime. For instance, casting a string to an integer without proper validation can result in an exception.
string text = "123";
int number = (int)text; // InvalidCastException at runtime.
Data Loss: Explicitly casting from a larger data type to a smaller one can result in data loss or overflow if the value exceeds the range of the destination type.
long largeValue = long.MaxValue;
int intValue = (int)largeValue; // Data loss due to overflow.
Loss of Precision: Casting from a high-precision data type like double to a lower-precision type like float can lead to a loss of precision and affect the accuracy of calculations.
double highPrecisionValue = 1234.567890123456789012345;
float floatValue = (float)highPrecisionValue; // Loss of precision.
Null Reference Exception: When casting reference types, like objects or class instances, to an incompatible type, a null reference exception can occur if the object is null.
object obj = null;
string str = (string)obj; // NullReferenceException.
Unchecked Conversions: Explicit conversions do not check for bounds or validity by default. It’s possible to cast values that might make no sense in the context of your program.
int invalidValue = (int)10000000000; // No compiler warning, but the value is too lar
Inferred Declarations in C# – Var
Inferred declarations, often associated with the use of the var keyword, allow for more concise and flexible code. Instead of explicitly specifying the data type of a variable, developers can use var, and the compiler will automatically determine the appropriate data type based on the assigned value.
This feature simplifies code writing, reduces redundancy, and makes code more maintainable, especially when dealing with complex or lengthy data types. Inferred declarations are particularly useful when working with LINQ queries or when the data type’s name might be lengthy or less relevant, as it emphasizes the variable’s purpose over its exact type.
While inferred declarations offer advantages in readability and flexibility, it’s essential to use them judiciously to maintain code clarity and ensure that the code remains self-explanatory to other developers.
One key issue using inferred declarations is reduced code clarity, as it obscures the actual data type of a variable, potentially making the code less self-explanatory. This lack of explicit type information can hinder the understanding of code, especially in larger and more complex projects, or when collaborating with other developers.
Additionally, inferred declarations may not be suitable when the variable’s type is crucial for documentation or when dealing with methods or interfaces that require explicit type declarations. Overuse of var can also lead to inconsistent coding practices within a project, making the codebase less maintainable.
Custom Types in C#
Custom types are user-defined data structures that allow developers to encapsulate and represent complex data in a way that fits their specific needs. These custom types, often created using classes or structs, provide a higher level of abstraction, enabling developers to model real-world entities or abstract concepts more accurately.
By defining custom types, developers can bundle data and associated behaviors (methods) into cohesive units, promoting code organization, modularity, and reusability. This approach enhances the maintainability and scalability of software projects, making it easier to work with and understand the codebase. In addition to classes and structs, C# offers other ways to define custom types.
Enums (Enumerations)
Enums allow you to define a set of named integral constants. They are useful for representing a fixed set of related values. Enums are value types and are often used to improve code readability by providing meaningful names for specific integer values.
enum DaysOfWeek { Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday }
Delegates
Delegates are custom types that represent references to methods with a particular parameter list and return type. They are commonly used in event handling and callback scenarios, allowing for dynamic method invocation.
delegate void MyDelegate(int x);
Interfaces
Interfaces define a contract that classes can implement. They specify a set of methods and properties that implementing classes must provide. Interfaces are used to achieve polymorphism and code abstraction.
interface IDrawable
{
void Draw();
}
Records (C# 9.0 and later)
Records are a concise way to define data-only classes with value semantics. They automatically generate properties, equality checks, and other methods for you, making them suitable for immutable data.
record Person(string FirstName, string LastName);
Tuples
Tuples are a lightweight way to create custom types for grouping multiple values together. They are particularly useful for returning multiple values from a method or as a way to express ad-hoc data structures.
(int, string) person = (25, "Alice");
Enums with Flags
When working with sets of bit flags, you can use enums with the [Flags] attribute to create custom types for bitwise operations.
[Flags]
enum Permissions { Read = 1, Write = 2, Execute = 4 }
Variable Scope in C#
Variable scope refers to the region of code where a particular variable is accessible and can be used. C# defines several levels of scope.
Block Scope
Variables declared within a block of code, typically enclosed within curly braces {}, have block scope. They are only accessible within that block and its nested blocks. Once the block exits, the variable goes out of scope and cannot be used.
{
int x = 10; // x is in block scope
}
// x is out of scope here
Method Scope
Variables declared as method parameters or within a method have method scope. They are accessible throughout the entire method but are not visible outside of it.
void MyMethod()
{
int y = 20; // y has method scope
}
// y is out of scope here
Class Scope
Variables declared at the class level (instance variables) are accessible by all methods and properties within the class. They exist if the class instance exists.
class MyClass
{
int z = 30; // z has class scope
void MyMethod()
{
// z can be accessed here
}
}
Namespace and Global Scope
Variables declared at the namespace level are accessible to all classes and methods within that namespace. Global variables can be accessed across different namespaces, but their use is generally discouraged due to potential naming conflicts and reduced code maintainability.
Value Types and Reference Types in C#
In C#, data types can be broadly categorized into two categories: reference types and value types. Understanding the distinction between these two is fundamental for efficient memory management and proper variable behavior.
Value Types
Value types include simple data types like integers (int), floating-point numbers (float), and structures (structs). These types store their actual data directly in memory, and when you assign a value type to another variable or pass it as an argument to a method, a copy of the data is made.
As a result, changes to one variable do not affect others, ensuring isolation and predictability. Value types are usually stored on the stack, making them faster to allocate and deallocate. However, this also means that they have a limited scope, and their memory is released when they go out of scope.
Reference Types
On the other hand, reference types, such as classes, interfaces, and arrays, store a reference to the data in memory rather than the actual data itself. When you assign a reference type to another variable or pass it to a method, you are passing a reference, so changes made to one instance affect all references to that instance.
Reference types are typically stored on the heap, making them more flexible for dynamic memory allocation but slower than value types in terms of memory management. Understanding the differences between reference and value types is crucial for efficient resource utilization and ensuring the desired behavior of your C# programs.
Stack versus Heap
Stack and heap are two distinct areas of memory used for different purposes. The stack is a region of memory used for storing local variables and managing function call frames, following a Last-In, First-Out (LIFO) order. It is generally efficient and has limited memory, making it suitable for storing variables with a short lifespan.
In contrast, the heap is a larger and more flexible region of memory used for dynamic memory allocation, where objects are created and deallocated manually. The heap is suitable for storing data with a longer lifespan, such as objects that need to persist beyond the scope of a single function call, but it requires more careful memory management and can be less efficient due to its dynamic nature.
Generics in C#
C# Generics is a powerful feature that allows developers to create reusable, type-safe, and efficient code by writing classes, methods, and interfaces that can work with various data types without sacrificing type safety.
By using generic types and methods, developers can design code that adapts to different data types at compile time, rather than relying on runtime casting or conversion. This not only enhances code reusability but also improves performance by eliminating the need for boxing and unboxing operations.
Generics enable the creation of highly flexible and adaptable code libraries and data structures, such as collections (e.g., List<T>), where the type parameter T represents the specific data type the collection will hold.
public class MyGenericClass<T>
{
private T myField;
public MyGenericClass(T value)
{
myField = value;
}
public T GetValue()
{
return myField;
}
}
Null safety in C#
Null safety aims to eliminate null reference exceptions and enhance code reliability. With the introduction of features like nullable value types and nullable reference types in C# 8.0 and later versions, developers have more powerful tools to handle null values safely.
Nullable value types, represented by a ? modifier (e.g., int?), allow variables to hold either a value or a null, offering a safer alternative to traditional value types. Nullable reference types, on the other hand, help catch potential null reference exceptions at compile time by annotating reference types with nullability information.
This means that developers must explicitly handle null cases or indicate that a variable cannot be null. By promoting null safety, C# encourages robust code that reduces the risk of runtime errors and improves code maintainability and quality, especially in large and complex software projects.
Methods in C#
A method signature in programming defines the essential characteristics of a method, such as its name, return type, and the types and order of its parameters. It serves as a unique identifier for a specific method within a program.
Method Signature
In languages like C#, the method signature is a critical component of method overloading and determining method compatibility in interfaces and base classes. For example, in the method signature
int CalculateSum(int a, int b)
“CalculateSum” is the method name, “int” is the return type, and “int a, int b” specifies the parameters, their types, and their order.
Method Parameters
Method parameters are inputs that a function or method in programming can accept to perform specific tasks or computations. They act as placeholders for values that are passed into the method when it is called. Parameters enable developers to create reusable and versatile code, allowing functions to work with different data without needing to be rewritten.
In C#, method parameters are defined within parentheses following the method name and serve as a means of communication between the calling code and the method itself. For example, a method that calculates the sum of two numbers might have parameters like int a and int b, and when the method is called with specific values, such as CalculateSum(5, 10), those values are used within the method’s logic.
Method Return Types
Method return values in programming represent the data or information that a function or method provides as output after it has executed its instructions. These return values allow functions to communicate results or computed data back to the calling code.
In C#, the return value is often defined by specifying the data type that the method will return, and it can be of any valid data type, from integers and strings to custom objects. For instance, a method that calculates the area of a rectangle might have a return value defined as double, indicating it will return a decimal number.
When the method is invoked, it performs the calculation and provides the result as its return value. This feature is crucial for retrieving and using the output of functions, making it possible to store, manipulate, and display the results of computations or operations within a program.
C# Methods in Unity
C# methods play a pivotal role in Unity game development, facilitating the creation of interactive and dynamic gameplay experiences. Unity leverages C# methods extensively for various purposes, and understanding their specifics is essential for game developers.
Unity Built-in Methods
In Unity, C# methods can be divided into two broad categories: built-in Unity methods and custom methods. Built-in Unity methods, often referred to as Unity’s “lifecycle methods,” are predefined functions that Unity calls automatically at specific points during a game’s execution.
For instance, the Start() method is invoked when an object is initialized, while Update() is called every frame. These methods enable developers to control the behavior of game objects and implement game logic seamlessly.
Custom Methods
Custom methods, on the other hand, are functions that developers create to perform specific tasks or implement custom game functionality. These methods can be called from Unity’s built-in methods or in response to player input, events, or other triggers.
One of the key features of C# methods in Unity is their ability to interact with Unity’s components and GameObjects. Developers can access and manipulate the properties, transform, and behavior of game objects using C# methods.
Additionally, methods in Unity often work in tandem with Unity’s event system, allowing developers to respond to events like collisions, button clicks, or animation events, enhancing interactivity and gameplay dynamics.
Coroutines
Furthermore, Unity supports coroutines, a specific type of C# method that can pause their execution and resume later. Coroutines are frequently used for animations, delays, and asynchronous tasks in Unity. They enable developers to create smooth and responsive gameplay experiences by handling time-consuming operations without freezing the game.
In conclusion, C# methods in Unity provide a robust framework for developers to control and customize their games. By mastering the use of these methods, developers can create engaging and interactive gaming experiences that captivate players and bring their creative visions to life.
For a general discussion about programming languages read this.
Take a tour of C#, the language used in Unity, which is used in our guides.